C# and .NET have adapted to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET 5 introduce record type and performance optimization. 2) .NET Core enhances cloud native and containerized support. 3) ASP.NET Core integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
introduction
In the ever-changing world of technology, the C# and .NET ecosystems have become indispensable tools for developers. They are not only the pride of Microsoft, but also the strong support of the global developer community. Through this article, we will explore how C# and .NET can adapt to the wave of emerging technologies and prepare for future development. Whether you are a beginner or experienced developer, after reading this article, you will have a deeper understanding of the role of C# and .NET in future technologies.
Review of basic knowledge
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. It combines the power of C and the simplicity of Java to increase the productivity of developers. .NET is a development platform launched by Microsoft that supports a variety of programming languages and libraries, helping developers create various types of applications, from desktop applications to web services, and then mobile applications.
C# and .NET have undergone multiple updates and improvements over the past few years, enhancing their functionality and performance. Understanding these basics is essential for us to explore how they adapt to new technologies.
Core concept or function analysis
Evolution of C# and .NET
The evolution of C# and .NET has always been the focus of Microsoft. As technology continues to develop, they are also constantly adapting to new needs and trends. The release of C# 9.0 and .NET 5 marks an important milestone, introducing many new features and improvements such as record types, pattern matching enhancements, and performance optimization.
// Example of record type in C# 9.0 public record Person(string FirstName, string LastName); <p>public class Program { public static void Main() { var person = new Person("John", "Doe"); Console.WriteLine(person); // Output: Person { FirstName = John, LastName = Doe } } }</p>
Record types simplify the creation and use of immutable data, which is increasingly important in modern programming. In this way, C# and .NET demonstrate their keen insights and rapid response to new technology trends.
Cloud native and containerized
The rise of cloud computing and containerization technologies has had a profound impact on C# and .NET. Microsoft launched the Azure cloud platform and optimized .NET to better adapt to the cloud environment. The release of .NET Core further enhances .NET's capabilities in cross-platform and containerization.
// Build .NET Core application using Dockerfile FROM mcr.microsoft.com/dotnet/sdk:5.0 AS build WORKDIR /app <h1 id="Copy-csproj-and-restore-dependencies">Copy csproj and restore dependencies</h1><p> COPY *.csproj ./ RUN dotnet restore</p><h1 id="Copy-the-project-file-and-build-the-release"> Copy the project file and build the release</h1><p> COPY . ./ RUN dotnet publish -c Release -o out</p><h1 id="Build-a-runtime-image"> Build a runtime image</h1><p> FROM mcr.microsoft.com/dotnet/aspnet:5.0 AS runtime WORKDIR /app COPY --from=build /app/out ./ ENTRYPOINT ["dotnet", "MyApp.dll"]</p>
In this way, developers can easily deploy .NET applications to containers for greater portability and scalability. However, containerization also brings some challenges, such as optimization of image size and startup time, which developers need to pay attention to in practice.
Example of usage
Integration with modern web technologies
C# and .NET play an important role in modern web development. With ASP.NET Core, developers can create high-performance web applications and integrate seamlessly with front-end frameworks such as React, Angular, and Vue.js.
// Example of ASP.NET Core integration with React using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; <p>public class Startup { public void ConfigureServices(IServiceCollection services) { services.AddControllersWithViews(); services.AddSpaStaticFiles(configuration => configuration.RootPath = "ClientApp/build"); }</p><pre class='brush:php;toolbar:false;'> public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Error"); app.UseHsts(); } app.UseStaticFiles(); app.UseSpaStaticFiles(); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllerRoute( name: "default", pattern: "{controller}/{action=Index}/{id?}"); }); app.UseSpa(spa => { spa.Options.SourcePath = "ClientApp"; if (env.IsDevelopment()) { spa.UseReactDevelopmentServer(npmScript: "start"); } }); }
}
This integration not only improves development efficiency, but also allows C# and .NET to remain competitive in modern web development. However, developers need to pay attention to the complexity and debugging difficulty caused by front-end separation.
Machine Learning and Artificial Intelligence
With the popularity of machine learning and artificial intelligence technologies, C# and .NET have also begun to make efforts in this regard. Microsoft has launched ML.NET, an open source framework for machine learning, allowing developers to train and deploy machine learning models using C# and .NET.
// Example of sentiment analysis using ML.NET using Microsoft.ML; using Microsoft.ML.Data; <p>public class SentimentData { [LoadColumn(0)] public string SentimentText;</p><pre class='brush:php;toolbar:false;'> [LoadColumn(1)] public bool Sentiment;
}
public class SentimentPrediction { [ColumnName("PredictedLabel")] public bool Prediction { get; set; }
public float Score { get; set; }
}
class Program { static void Main(string[] args) { MLContext mlContext = new MLContext();
// Load data var data = mlContext.Data.LoadFromTextFile<SentimentData>("sentiment_data.tsv", hasHeader: true); // Build and train the model var pipeline = mlContext.Transforms.Text.FeaturizeText("Features", nameof(SentimentData.SentimentText)) .Append(mlContext.BinaryClassification.Trainers.SdcaLogisticRegression()); var model = pipeline.Fit(data); // Prediction var predictionEngine = mlContext.Model.CreatePredictionEngine<SentimentData, SentimentPrediction>(model); var sampleStatement = new SentimentData { SentimentText = "This is a great movie!" }; var prediction = predictionEngine.Predict(sampleStatement); Console.WriteLine($"Sentiment: {(Convert.ToBoolean(prediction.Prediction) ? "Positive" : "Negative")}"); }
}
With ML.NET, developers can leverage C# and .NET for machine learning tasks. However, training and optimization of machine learning models requires a large amount of data and computing resources, which poses new challenges for developers.
Performance optimization and best practices
In practical applications, performance optimization and best practices are crucial for C# and .NET development. By using technologies such as asynchronous programming, parallel processing, and memory management, developers can significantly improve application performance.
// Asynchronous programming example using System; using System.Threading.Tasks; <p>class Program { static async Task Main(string[] args) { Console.WriteLine("Starting..."); await DoWorkAsync(); Console.WriteLine("Finished!"); }</p><pre class='brush:php;toolbar:false;'> static async Task DoWorkAsync() { await Task.Delay(1000); // Simulate time-consuming operation Console.WriteLine("Work completed."); }
}
Asynchronous programming can improve application responsiveness and throughput, but developers also need to pay attention to the complexity of asynchronous code and the difficulty of debugging. In addition, developers need to pay attention to the readability and maintenance of the code, and follow SOLID principles and design patterns to ensure the quality and scalability of the code.
In general, C# and .NET demonstrate their strong vitality and flexibility in the process of constantly adapting to new technologies. Through continuous innovation and optimization, they will continue to play an important role in future technological development.
The above is the detailed content of C# .NET and the Future: Adapting to New Technologies. For more information, please follow other related articles on the PHP Chinese website!
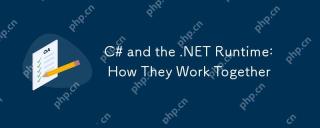
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
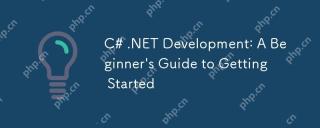
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
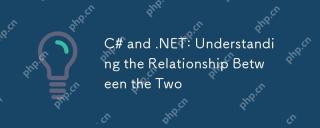
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
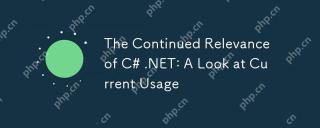
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
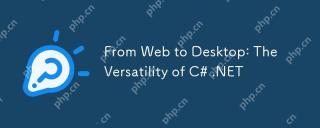
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
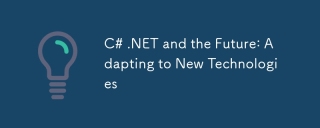
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
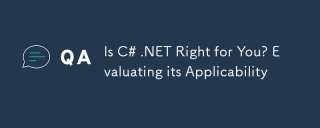
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
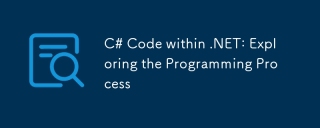
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
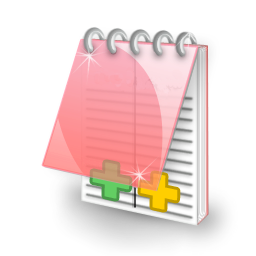
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
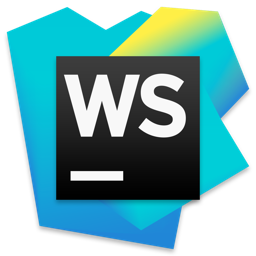
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.