


Testing C# .NET Applications: Unit, Integration, and End-to-End Testing
C# .NET applications test strategies include unit testing, integration testing, and end-to-end testing. 1. Unit testing ensures that the minimum unit of the code works independently, using the MSTest, NUnit or xUnit framework. 2. Integrated testing verifies the functions of multiple units combined, commonly used simulated data and external services. 3. End-to-end testing simulates the user's complete operation process, usually using Selenium for automated testing.
introduction
In the world of software development, testing is like a safety net for the code we write. Especially when developing with C# and .NET, testing is not only a critical step to ensure the quality of your code, but also an art. Today, we will dive into testing strategies for C# .NET applications, including unit testing, integration testing and end-to-end testing. Through this article, you will learn how to effectively test your C# application and understand the advantages and challenges of different test types.
Review of basic knowledge
Testing is everywhere in software development, but we need to clarify several major test types. Unit testing focuses on the smallest unit of code, usually a method or function. Integration tests check whether multiple units work correctly together. End-to-end testing simulates the user's complete operation process to ensure that the entire system works as expected.
In C# .NET, our commonly used testing frameworks include MSTest, NUnit and xUnit. These frameworks provide a wealth of tools and APIs to help us write and run tests.
Core concept or function analysis
Definition and function of unit tests
Unit testing is the minimum granularity of the test, which ensures that each code unit works independently. Through unit testing, we can quickly locate and fix problems and improve the maintainability and reliability of our code. The core of unit testing is its independence and rapid feedback.
A simple unit test example:
using Xunit; public class CalculatorTests { [Fact] public void Add_TwoPositiveNumbers_ReturnsCorrectSum() { // Arrange var calculator = new Calculator(); // Act var result = calculate.Add(2, 3); // Assert Assert.Equal(5, result); } }
This code shows a unit test of a simple addition operation. In this way, we can ensure that the Add
method in Calculator
class works correctly under various inputs.
How integration testing works
The purpose of integration testing is to verify the functionality of multiple units combined. It works by simulating data flow and interaction in a real environment, ensuring that components can work seamlessly. Integration testing often requires more setup and mock data, but it can detect integration problems that unit tests cannot capture.
An example of integration test:
using Xunit; public class UserServiceTests { [Fact] public async Task GetUser_ValidUserId_ReturnsUser() { // Arrange var userService = new UserService(new FakeUserRepository()); var userId = "123"; // Act var user = await userService.GetUser(userId); // Assert Assert.NotNull(user); Assert.Equal("John Doe", user.Name); } }
In this example, we tested the UserService
class, which relies on a user repository. We use a fake repository to simulate real data sources, thus verifying the logic of the service layer.
The implementation principle of end-to-end testing
End-to-end testing simulates the complete operational process of users, usually involving UI interaction and database operations. Its implementation principle is to simulate user behavior through automation tools (such as Selenium) and check whether the system's functions from beginning to end are normal.
An end-to-end test example:
using Xunit; using OpenQA.Selenium; using OpenQA.Selenium.Chrome; public class LoginTests { [Fact] public void Login_ValidCredentials_RedirectsToDashboard() { // Arrange IWebDriver driver = new ChromeDriver(); driver.Navigate().GoToUrl("https://example.com/login"); // Act driver.FindElement(By.Id("username")).SendKeys("user"); driver.FindElement(By.Id("password")).SendKeys("password"); driver.FindElement(By.Id("loginButton")).Click(); // Assert Assert.Contains("Dashboard", driver.Title); driver.Quit(); } }
This example shows how to use Selenium for end-to-end testing, simulate user login operations and verify that it successfully jumps to the dashboard.
Example of usage
Basic usage of unit testing
The basic usage of unit testing is to write independent testing methods, each testing a specific function or behavior. Here is a simple example:
using Xunit; public class StringCalculatorTests { [Fact] public void Add_EmptyString_ReturnsZero() { var calculate = new StringCalculator(); var result = calculate.Add(""); Assert.Equal(0, result); } [Fact] public void Add_SingleNumber_ReturnsNumber() { var calculate = new StringCalculator(); var result = calculate("5"); Assert.Equal(5, result); } }
These test methods test the behavior of the Add
method of StringCalculator
class under empty strings and single numeric inputs, respectively.
Advanced usage of integration testing
Advanced usage of integration testing includes mocking external services and database operations. Here is an example of using the Moq library to simulate external services:
using Xunit; using Moq; public class OrderServiceTests { [Fact] public async Task PlaceOrder_ValidOrder_CallsPaymentService() { // Arrange var mockPaymentService = new Mock<IPaymentService>(); var orderService = new OrderService(mockPaymentService.Object); var order = new Order { Amount = 100 }; // Act await orderService.PlaceOrder(order); // Assert mockPaymentService.Verify(ps => ps.ProcessPayment(order.Amount), Times.Once); } }
In this example, we use the Moq library to simulate the payment service and verify that OrderService
calls the payment service correctly when placing an order.
Common Errors and Debugging Tips for End-to-End Testing
Common errors in end-to-end testing include element positioning failures, insufficient waiting time, etc. Here are some debugging tips:
- Use Explicit Waits to ensure that the element loads:
var wait = new WebDriverWait(driver, TimeSpan.FromSeconds(10)); var element = wait.Until(d => d.FindElement(By.Id("myElement")));
- Use logging to track the test execution process to help locate problems:
using Microsoft.Extensions.Logging; public class LoginTests { private readonly ILogger<LoginTests> _logger; public LoginTests(ILogger<LoginTests> logger) { _logger = logger; } [Fact] public void Login_ValidCredentials_RedirectsToDashboard() { _logger.LogInformation("Starting login test"); // ... Test code... _logger.LogInformation("Login test completed"); } }
Performance optimization and best practices
Performance optimization and best practices are crucial when testing C# .NET applications. Here are some suggestions:
- Test Coverage : Make sure your tests cover critical code paths. Use a tool such as Coverlet to measure test coverage:
dotnet test /p:CollectCoverage=true /p:CoverletOutputFormat=lcov /p:CoverletOutput=./coverage/lcov.info
- Test parallelization : Use the parallelization function of the test framework to accelerate test execution. For example, in xUnit, the parallel execution of tests can be controlled through the
[collection]
attribute:
[Collection("MyCollection")] public class MyTests { // Test method}
- Code readability : Write clear and concise test code with meaningful names and comments:
[Fact] public void CalculateTotalPrice_WithDiscount_ApplyDiscountCorrectly() { // Arrange var order = new Order { Price = 100, Discount = 10 }; // Act var totalPrice = order.CalculateTotalPrice(); // Assert Assert.Equal(90, totalPrice); // 100 - 10% = 90 }
Through these strategies and practices, we can not only improve the efficiency and quality of testing, but also ensure that our C# .NET applications can operate stably in various scenarios. I hope this article will provide you with valuable insights and practical tips to help you go further on the road to testing.
The above is the detailed content of Testing C# .NET Applications: Unit, Integration, and End-to-End Testing. For more information, please follow other related articles on the PHP Chinese website!
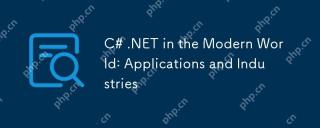
C#.NET is widely used in the modern world in the fields of game development, financial services, the Internet of Things and cloud computing. 1) In game development, use C# to program through the Unity engine. 2) In the field of financial services, C#.NET is used to develop high-performance trading systems and data analysis tools. 3) In terms of IoT and cloud computing, C#.NET provides support through Azure services to develop device control logic and data processing.
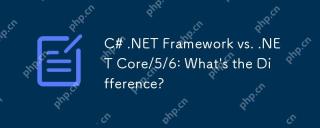
.NETFrameworkisWindows-centric,while.NETCore/5/6supportscross-platformdevelopment.1).NETFramework,since2002,isidealforWindowsapplicationsbutlimitedincross-platformcapabilities.2).NETCore,from2016,anditsevolutions(.NET5/6)offerbetterperformance,cross-
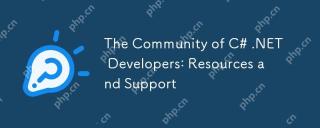
The C#.NET developer community provides rich resources and support, including: 1. Microsoft's official documents, 2. Community forums such as StackOverflow and Reddit, and 3. Open source projects on GitHub. These resources help developers improve their programming skills from basic learning to advanced applications.
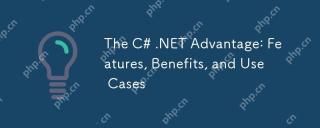
The advantages of C#.NET include: 1) Language features, such as asynchronous programming simplifies development; 2) Performance and reliability, improving efficiency through JIT compilation and garbage collection mechanisms; 3) Cross-platform support, .NETCore expands application scenarios; 4) A wide range of practical applications, with outstanding performance from the Web to desktop and game development.
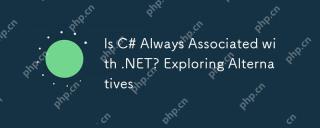
C# is not always tied to .NET. 1) C# can run in the Mono runtime environment and is suitable for Linux and macOS. 2) In the Unity game engine, C# is used for scripting and does not rely on the .NET framework. 3) C# can also be used for embedded system development, such as .NETMicroFramework.
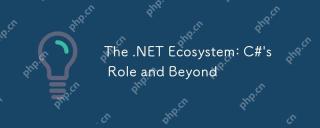
C# plays a core role in the .NET ecosystem and is the preferred language for developers. 1) C# provides efficient and easy-to-use programming methods, combining the advantages of C, C and Java. 2) Execute through .NET runtime (CLR) to ensure efficient cross-platform operation. 3) C# supports basic to advanced usage, such as LINQ and asynchronous programming. 4) Optimization and best practices include using StringBuilder and asynchronous programming to improve performance and maintainability.
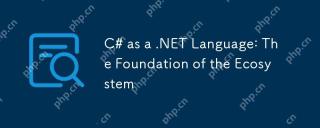
C# is a programming language released by Microsoft in 2000, aiming to combine the power of C and the simplicity of Java. 1.C# is a type-safe, object-oriented programming language that supports encapsulation, inheritance and polymorphism. 2. The compilation process of C# converts the code into an intermediate language (IL), and then compiles it into machine code execution in the .NET runtime environment (CLR). 3. The basic usage of C# includes variable declarations, control flows and function definitions, while advanced usages cover asynchronous programming, LINQ and delegates, etc. 4. Common errors include type mismatch and null reference exceptions, which can be debugged through debugger, exception handling and logging. 5. Performance optimization suggestions include the use of LINQ, asynchronous programming, and improving code readability.
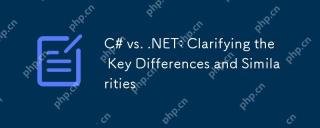
C# is a programming language, while .NET is a software framework. 1.C# is developed by Microsoft and is suitable for multi-platform development. 2..NET provides class libraries and runtime environments, and supports multilingual. The two work together to build modern applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
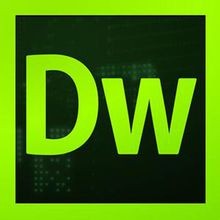
Dreamweaver CS6
Visual web development tools
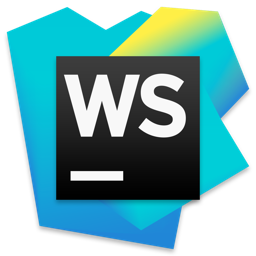
WebStorm Mac version
Useful JavaScript development tools
