How to optimize code structure and modularity in PHP development
How to optimize the code structure and modularization in PHP development
As a scripting language widely used in Web development, PHP’s flexibility and ease of use create A large number of PHP applications. However, as projects increase in size and complexity, developers need to pay more attention to code structure and modularity to improve code readability, maintainability, and scalability. In this article, I will introduce some methods to optimize code structure and modularization in PHP development, and provide specific code examples.
- Using namespaces
Namespaces are a feature introduced in PHP5 that allow developers to organize code into different logical packages to avoid naming conflicts. By using namespaces, we can better organize the code and improve the readability and maintainability of the code. The following is an example of using namespaces:
namespace MyAppControllers; class UserController { // ... }
- Using classes and objects
Object-oriented programming is an important concept in PHP development. By using classes and objects, we Code can be better organized and code flexibility and reusability can be achieved. Here is an example of using classes and objects:
class User { private $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } $user = new User("John"); echo $user->getName(); // 输出 "John"
- Using design patterns
Design patterns are a general solution for solving problems in software design and development. Frequently Asked Questions. In PHP development, we can use some common design patterns, such as singleton pattern, factory pattern, observer pattern, etc., to improve the scalability and flexibility of the code. The following is an example of using the factory pattern:
interface UserInterface { public function getName(); } class User implements UserInterface { private $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } class UserFactory { public static function createUser($name) { return new User($name); } } $user = UserFactory::createUser("John"); echo $user->getName(); // 输出 "John"
- Using automatic loading
PHP provides an automatic loading mechanism that can automatically load the required class files, thereby simplifying the code Class introduction and file inclusion operations in . By using the automatic loading mechanism, we can avoid manually introducing and including class files, improving the readability and maintainability of the code. Here is an example of using autoloading:
spl_autoload_register(function ($class) { require_once 'path/to/classes/' . $class . '.php'; }); $user = new User("John"); echo $user->getName(); // 输出 "John"
- Use modular architecture
Modularizing your code is an effective way to organize your code so that functionality is related code together and decouple the code through interfaces and dependency injection. By using a modular architecture, we can better manage and maintain the code and achieve code scalability. The following is an example of using modularity:
namespace MyAppControllers; use MyAppModelsUserModel; class UserController { private $userModel; public function __construct(UserModel $userModel) { $this->userModel = $userModel; } public function getUser($id) { return $this->userModel->getUser($id); } } $userController = new UserController(new UserModel()); $user = $userController->getUser(1);
Summary:
Optimizing the code structure and modularization in PHP development is the key to improving code quality and development efficiency. By using namespaces, classes and objects, design patterns, automatic loading, and modular architecture, we can better organize and manage code and improve code readability, maintainability, and scalability. I hope the methods and examples provided in this article will be helpful to you in optimizing code structure and modularization in PHP development.
The above is the detailed content of How to optimize code structure and modularity in PHP development. For more information, please follow other related articles on the PHP Chinese website!
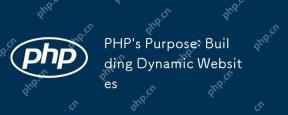
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
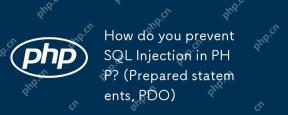
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
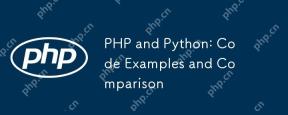
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
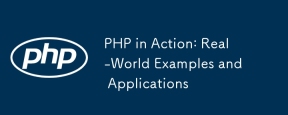
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
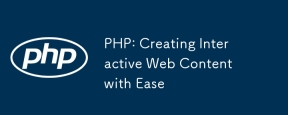
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
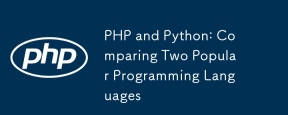
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
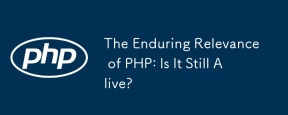
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
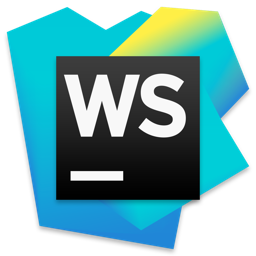
WebStorm Mac version
Useful JavaScript development tools
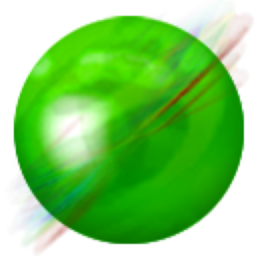
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment