Common coding specifications and best practices in Java development
In Java development, good coding specifications and best practices are crucial to maintaining the readability and Maintainability and scalability are very important. This article will introduce some common coding standards and best practices, and provide corresponding code examples.
- Naming convention
In Java, good naming conventions can make the code easier to understand. Here are some naming convention best practices: - Use descriptive variable, method, and class names. Avoid using single characters or abbreviations for names.
- Variable and method names use camel case naming, that is, the first letter is lowercase, and the first letter of subsequent words is capitalized.
- The class name adopts camel case naming method, that is, the first letter is capitalized, and the first letter of subsequent words is capitalized.
Example:
// 不好的命名规范 int a; String s; void m(); // 好的命名规范 int age; String name; void printMessage(); class EmployeeDetails;
- Comments
Good comments can make the code easier to understand and maintain. Here are some best practices for comments: - Add comments in key places, including descriptions of methods, classes, and fields.
- Use natural language instead of just describing the code implementation.
- Avoid using excessive or lengthy comments and only add necessary comments.
- Update comments to reflect code changes.
Example:
/** * 根据给定的数字,计算其平方值。 * @param number 需要计算的数字 * @return 给定数字的平方值 */ public int calculateSquare(int number) { return number * number; }
- Exception handling
In Java, good exception handling helps to improve the robustness of the program. Here are some best practices for exception handling: - Don’t ignore exceptions, at least log them.
- Use try-catch blocks to catch and handle exceptions that may be thrown.
- Avoid handling multiple unrelated exceptions in a try-catch block, they should be handled separately.
- Release resources in the finally block, such as closing a database connection or file.
Example:
try { // 执行可能引发异常的代码 } catch (IOException e) { logger.error("读取文件时发生异常:" + e.getMessage()); } catch (SQLException e) { logger.error("数据库操作异常:" + e.getMessage()); } finally { // 释放资源 }
- Class design
Good class design can make the code more scalable and maintainable. The following are some best practices for class design: - Follow the single responsibility principle, that is, each class should have a clear goal and responsibility.
- Use encapsulation to hide internal implementation details and provide a public interface.
- Avoid excessive inheritance and try to use combination to achieve code reuse.
- Use interfaces and abstract classes to achieve polymorphism and flexibility.
Example:
public interface Shape { double calculateArea(); double calculatePerimeter(); } public class Circle implements Shape { private double radius; public Circle(double radius) { this.radius = radius; } @Override public double calculateArea() { return Math.PI * radius * radius; } @Override public double calculatePerimeter() { return 2 * Math.PI * radius; } }
To sum up, following good coding standards and best practices is very important for Java development. This article introduces some common coding conventions and best practices, including naming conventions, annotations, exception handling, and class design. By following these best practices, you can improve code readability, maintainability, and scalability, thereby improving development efficiency and code quality.
The above is the detailed content of Common coding standards and best practices in Java development. For more information, please follow other related articles on the PHP Chinese website!
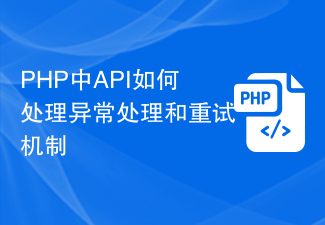
PHP中API如何处理异常处理和重试机制在PHP中,API已经成为许多网站和应用程序的核心,因为它们提供各种功能和功能。然而,在使用API时,我们经常会遇到许多问题,如网络连接问题,响应超时,无效请求等。在这种情况下,我们需要了解如何处理异常和重试机制来确保我们的应用程序的可靠性和稳定性。异常处理在PHP中,异常处理是一种更加优雅和可读的错误处
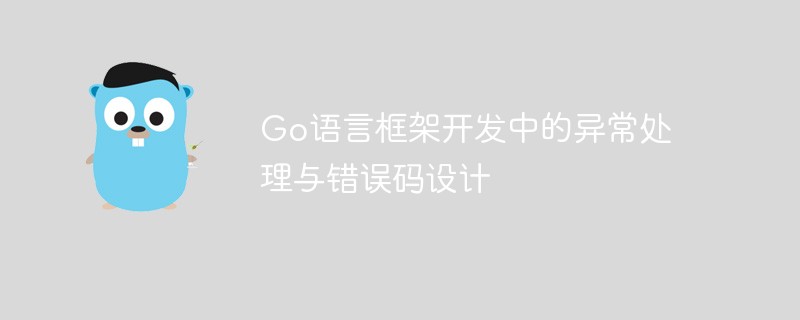
随着互联网技术的不断发展,越来越多的企业开始使用Go语言进行开发。Go语言以其高效、稳定、易用的特点备受开发者的青睐。在企业级开发中,框架是不可或缺的一部分。因此,本文将介绍在Go语言框架开发中,如何进行异常处理与错误码设计。一、什么是异常处理在计算机编程中,异常处理指的是当程序运行过程中出现异常情况时,程序必须采取的措施。这些异常情况包括硬件故障、软件缺陷
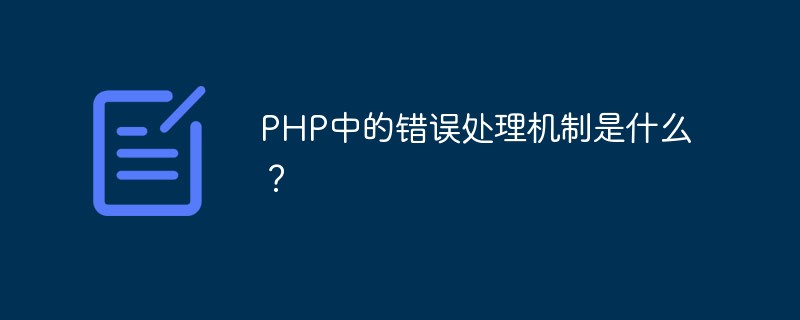
PHP是一种流行而强大的服务器端编程语言,可以用来开发各种Web应用程序。就像其他编程语言一样,PHP也有可能会出现错误和异常。这些错误和异常可能由各种原因引起,如程序错误、服务器错误、用户输入错误等等。为了确保程序的运行稳定性和可靠性,PHP提供了一套完整的错误处理机制。PHP错误处理机制的基本思想是:当发生错误时,程序会停止执行并输出一条错误消息。我们可
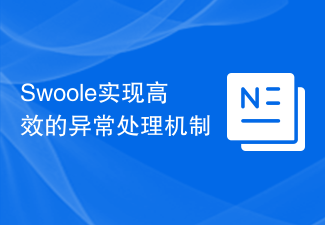
随着Web开发技术的不断发展,开发人员也面临着越来越复杂的业务场景和需求。例如,高并发、大量请求处理、异步任务处理等问题都需要使用高性能的工具和技术来解决。在这种情况下,Swoole成为了一种越来越重要的解决方案。Swoole是一种基于PHP语言的高性能异步网络通信框架。它提供了一些非常有用的功能和特性,例如异步IO、协程、进程管理、定时器和异步客户端,使得
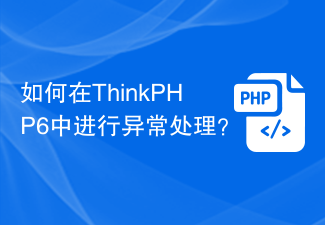
ThinkPHP6是一款非常流行的PHP框架,已经被广泛应用于各种Web应用程序中。在开发过程中,可能会遇到各种异常,如果不及时处理,就会导致程序无法正常运行。本文将介绍如何在ThinkPHP6中进行异常处理,保证Web应用程序的稳定性和可靠性。异常处理的概念异常处理是指在程序正常执行过程中,遇到错误或意外情况时所进行的处理。在开发Web应用程序时,常常会发
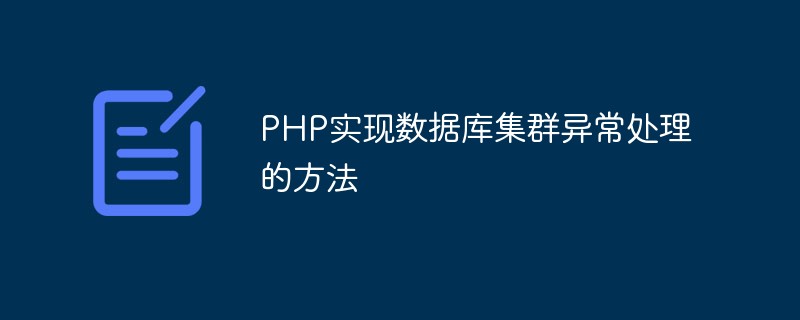
随着互联网的不断发展,越来越多的企业和组织开始规划数据库集群来满足其数据处理需求。数据库集群可能包含数百甚至数千个节点,因此在节点之间确保数据同步和协调非常重要。在该环境下,存在着很多的异常情况,如单节点故障,网络分区,数据同步错误等,并且需要实现实时检测和处理。本文将介绍如何使用PHP实现数据库集群异常处理。数据库集群的概述在数据库集群中,一个单独的
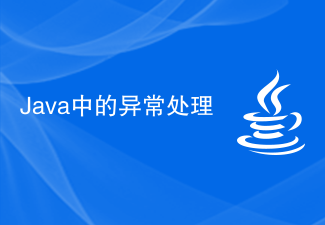
Java是一种面向对象的程序设计语言,由于其很高的稳定性和安全性,成为了一种广泛应用的编程语言。然而,在程序开发过程中,异常总是不可避免的问题。由于Java语言天生具有处理异常的功能,因此它可以通过异常处理机制来避免程序崩溃,保证程序的正常运行。一、Java中的异常概述在Java中,异常是指程序发生了不正常的情况,如数组越界、除数为零、文件未找到等等。但是这
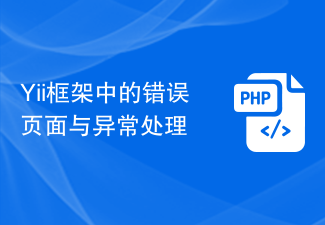
Yii框架是一款广泛应用于Web应用程序开发的高性能PHP框架。在Yii的应用程序中,错误页面和异常处理模块是非常重要的功能之一。本文将简要介绍Yii框架中的错误页面和异常处理模块,并提供一些实用的示例代码,以帮助您更好地理解和使用这些功能。一、错误页面当用户访问一个不存在的页面、发生了错误的连接或者其他错误时,Yii框架会默认显示一个错误页面。这个页面通常


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
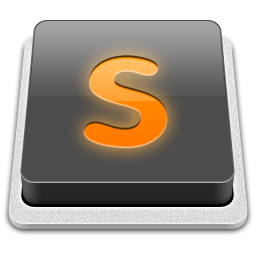
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
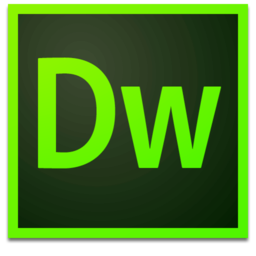
Dreamweaver Mac version
Visual web development tools
