How to process JSON data in Python requires specific code examples
Introduction
JSON (JavaScript Object Notation) is a commonly used Data exchange format, widely used for data transfer between various programming languages and platforms. In Python, we can use the built-in json
module to process JSON data. This article will introduce how to use the json
module in Python to parse and generate JSON data, and provide some specific code examples.
Parsing JSON data
When we need to get values from JSON data, we can use the json.loads()
function to parse the JSON string. Here is a simple example:
import json # JSON字符串 json_str = '{"name": "Alice", "age": 25}' # 解析JSON字符串 data = json.loads(json_str) # 获取值 name = data["name"] age = data["age"] print(name) # 输出: Alice print(age) # 输出: 25
In the above example, we first import the json
module. Then, we define a string json_str
that contains JSON data. Next, we use the json.loads()
function to parse the string into a Python object. Finally, we can get the value by key.
Generate JSON data
When we need to convert a Python object into a JSON string, we can use the json.dumps()
function. Here is an example:
import json # Python对象 data = { "name": "Bob", "age": 30 } # 生成JSON字符串 json_str = json.dumps(data) print(json_str) # 输出: {"name": "Bob", "age": 30}
In the above example, we have defined a dictionary object data
which contains name and age. We then use the json.dumps()
function to convert the Python object into a JSON string. Finally, we print out the generated JSON string.
Handling nested JSON data
Sometimes, JSON data may contain nested structures. In this case, we can use recursion to process nested JSON data. Here is an example:
import json # JSON字符串 json_str = '{"name": "Alice", "age": 25, "children": [{"name": "Bob", "age": 5}, {"name": "Charlie", "age": 3}]}' # 解析JSON字符串 data = json.loads(json_str) # 获取值 name = data["name"] age = data["age"] children = data["children"] # 遍历子对象 for child in children: child_name = child["name"] child_age = child["age"] print(child_name, child_age) print(name) # 输出: Alice print(age) # 输出: 25
In the above example, we have defined a JSON string containing nested structures json_str
. We use the json.loads()
function to parse the string into a Python object and get the value by key. When we encounter nested structures, we can iterate through the sub-objects by key and get their values.
Processing JSON data in files
In addition to processing JSON strings, we can also process JSON data stored in files. Here is an example:
import json # 打开文件 with open("data.json") as file: # 解析JSON数据 data = json.load(file) # 获取值 name = data["name"] age = data["age"] print(name) # 输出: Alice print(age) # 输出: 25
In the above example, we use the open()
function to open a file named data.json
and use json.load()
Function parses JSON data from a file. We can then get the value by key.
Summary
This article introduces how to process JSON data in Python and provides some specific code examples. Whether parsing JSON data or generating JSON data, the json
module can help us process JSON data easily. I hope this article can help readers better apply the json
module to deal with JSON data problems.
The above is the detailed content of How to process JSON data in Python. For more information, please follow other related articles on the PHP Chinese website!
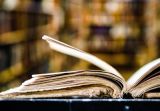
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
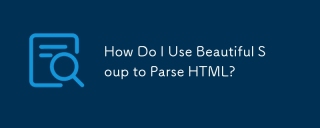
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
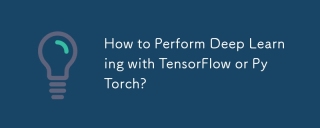
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
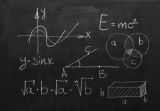
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
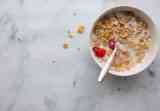
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
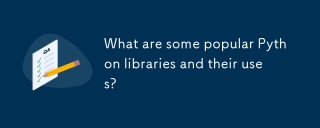
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
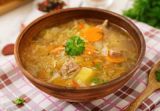
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
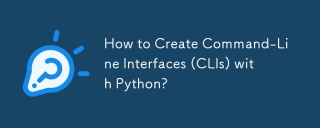
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
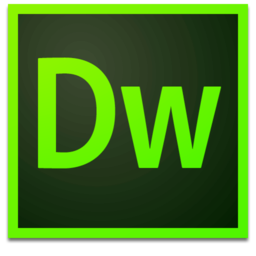
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
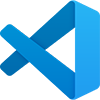
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
