The basic syntax for Python list slicing is list[start:stop:step]. 1. start is the first element index included, 2. stop is the first element index excluded, 3. step determines the step size between elements. Slices are not only used to extract data, but also to modify and invert lists.
Slicing a Python array, or more accurately a list, is a fundamental skill that every Python programmer should master. It's not just about cutting a piece of data; it's about understanding how to manipulate and access elements efficiently. Let's dive into the world of list slicing and explore its nuances.
When you're working with Python lists, slicing is your Swiss Army knife. It allows you to extract, modify, or even reverse portions of your list with ease. The basic syntax is list[start:stop:step]
, where start
is the index of the first element you want to include, stop
is the index of the first element you want to exclude, and step
determines the stride between elements.
Here's a simple example to get you started:
my_list = [0, 1, 2, 3, 4, 5] sliced_list = my_list[1:4] print(sliced_list) # Output: [1, 2, 3]
This code snippet slices my_list
from index 1 to 3 (remember, the stop index is exclusive), giving us [1, 2, 3]
.
Now, let's explore some more advanced slicing techniques. Ever needed to reverse a list? Slicing makes it a breeze:
reversed_list = my_list[::-1] print(reversed_list) # Output: [5, 4, 3, 2, 1, 0]
By using a step of -1
, we traverse the list from end to begin, effectively reversing it.
But slicing isn't just about reading data; it's also about modifying it. You can use slicing to replace portions of a list:
my_list[1:4] = [10, 20, 30] print(my_list) # Output: [0, 10, 20, 30, 4, 5]
This operation replaces the elements at indices 1, 2, and 3 with new values.
One of the beauty of Python slicing is its flexibility. You can omit any of the start
, stop
, or step
parameters, and Python will fill in sensible defaults. For instance, omitting start
means "start from the beginning," omitting stop
means "go until the end," and omitting step
means "use a step of 1."
Here's an example of using these defaults:
# Start from the beginning, go until index 3 print(my_list[:3]) # Output: [0, 10, 20] # Start from index 2, go until the end print(my_list[2:]) # Output: [20, 30, 4, 5] # Every second element print(my_list[::2]) # Output: [0, 20, 4]
Slicing also works with negative indices, which count from the end of the list. This can be particularly useful when you want to access elements from the end without knowing the list's length:
# Last three elements print(my_list[-3:]) # Output: [30, 4, 5] # Every second element, starting from the second-to-last print(my_list[-2::-2]) # Output: [4, 20, 0]
Now, let's talk about some common pitfalls and how to avoid them. One mistake I've seen is assuming that slicing creates a new list. In reality, slicing creates a shallow copy:
original = [[1, 2], [3, 4]] sliced = original[:] sliced[0][0] = 100 print(original) # Output: [[100, 2], [3, 4]] print(sliced) # Output: [[100, 2], [3, 4]]
As you can see, modifying the nested list in the sliced version affects the original. If you need a deep copy, consider using the copy
module.
Another thing to keep in mind is performance. While slicing is generally efficient, it can be slow for very large lists, especially if you're creating many slices. In such cases, consider using generators or iterators to process data in chunks.
In terms of best practices, always be mindful of your slicing operations. They can make your code more readable and concise, but overuse can lead to confusion. Use meaningful variable names for your slices, and consider adding comments to explain complex slicing operations.
To wrap up, slicing in Python is a powerful tool that, when mastered, can significantly enhance your coding efficiency and readability. It's not just about cutting lists; it's about understanding and manipulating data in a way that's both elegant and effective. So, go ahead, slice and dice your Python lists with confidence!
The above is the detailed content of How do you slice a Python array?. For more information, please follow other related articles on the PHP Chinese website!
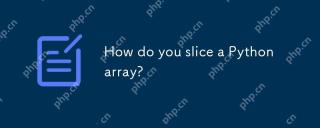
The basic syntax for Python list slicing is list[start:stop:step]. 1.start is the first element index included, 2.stop is the first element index excluded, and 3.step determines the step size between elements. Slices are not only used to extract data, but also to modify and invert lists.
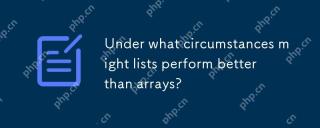
Listsoutperformarraysin:1)dynamicsizingandfrequentinsertions/deletions,2)storingheterogeneousdata,and3)memoryefficiencyforsparsedata,butmayhaveslightperformancecostsincertainoperations.
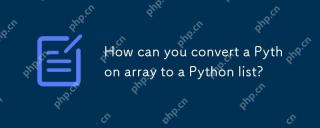
ToconvertaPythonarraytoalist,usethelist()constructororageneratorexpression.1)Importthearraymoduleandcreateanarray.2)Uselist(arr)or[xforxinarr]toconvertittoalist,consideringperformanceandmemoryefficiencyforlargedatasets.
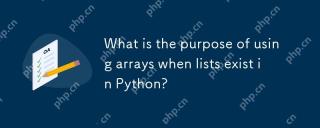
ChoosearraysoverlistsinPythonforbetterperformanceandmemoryefficiencyinspecificscenarios.1)Largenumericaldatasets:Arraysreducememoryusage.2)Performance-criticaloperations:Arraysofferspeedboostsfortaskslikeappendingorsearching.3)Typesafety:Arraysenforc
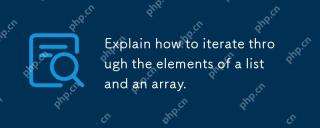
In Python, you can use for loops, enumerate and list comprehensions to traverse lists; in Java, you can use traditional for loops and enhanced for loops to traverse arrays. 1. Python list traversal methods include: for loop, enumerate and list comprehension. 2. Java array traversal methods include: traditional for loop and enhanced for loop.
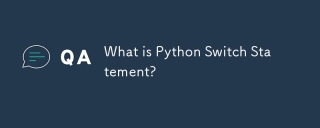
The article discusses Python's new "match" statement introduced in version 3.10, which serves as an equivalent to switch statements in other languages. It enhances code readability and offers performance benefits over traditional if-elif-el
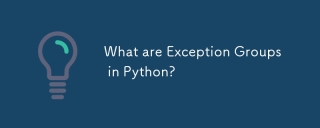
Exception Groups in Python 3.11 allow handling multiple exceptions simultaneously, improving error management in concurrent scenarios and complex operations.
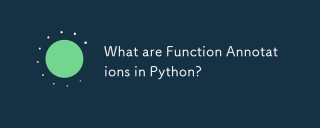
Function annotations in Python add metadata to functions for type checking, documentation, and IDE support. They enhance code readability, maintenance, and are crucial in API development, data science, and library creation.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
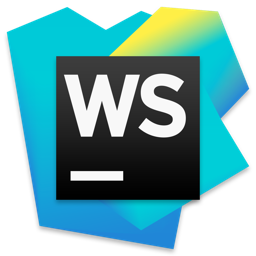
WebStorm Mac version
Useful JavaScript development tools
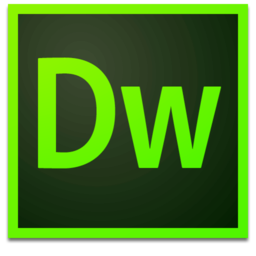
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
