To convert a Python array to a list, use the list() constructor or a generator expression. 1) Import the array module and create an array. 2) Use list(arr) or [x for x in arr] to convert it to a list, considering performance and memory efficiency for large datasets.
When it comes to converting a Python array to a Python list, the process is straightforward, but there are some nuances and best practices worth exploring. Let's dive into this topic, sharing insights and practical examples along the way.
In Python, what we commonly refer to as "arrays" are actually instances of the array
module, which provides a more memory-efficient and type-specific alternative to Python's built-in list
. Converting an array
to a list
is essential when you need the flexibility and functionality of a list
, such as the ability to hold different data types or to use list-specific methods.
Here's how you can convert an array
to a list
:
import array # Create an array of integers arr = array.array('i', [1, 2, 3, 4, 5]) # Convert the array to a list lst = list(arr) print(lst) # Output: [1, 2, 3, 4, 5]
This simple conversion works well, but there are some deeper considerations to keep in mind:
Performance: The
list()
constructor is efficient for small to medium-sized arrays. However, for very large arrays, you might want to consider using a list comprehension or a generator expression to avoid creating an intermediate list in memory.Type Safety: When you convert an
array
to alist
, you lose the type safety that thearray
provides. This means you can now add elements of different types to the list, which might lead to unexpected behavior if not managed carefully.Memory Usage: Arrays are more memory-efficient than lists because they store elements of a single type. Converting to a list might increase memory usage, especially for large datasets.
Let's explore a more memory-efficient approach using a generator expression:
import array # Create an array of integers arr = array.array('i', [1, 2, 3, 4, 5]) # Convert the array to a list using a generator expression lst = [x for x in arr] print(lst) # Output: [1, 2, 3, 4, 5]
This method is particularly useful for large arrays as it doesn't create an intermediate list in memory. It's also more flexible because you can add conditions or transformations within the comprehension.
Now, let's consider a scenario where you might want to transform the data during conversion:
import array # Create an array of integers arr = array.array('i', [1, 2, 3, 4, 5]) # Convert the array to a list with a transformation lst = [x * 2 for x in arr] print(lst) # Output: [2, 4, 6, 8, 10]
This approach allows you to perform operations on each element as you convert, which can be very powerful in data processing tasks.
When working with arrays and lists, it's important to be aware of potential pitfalls:
Data Integrity: After conversion, ensure that the data remains consistent with your application's requirements. If you need to maintain type safety, consider using a
numpy
array instead of a Pythonlist
.Performance Optimization: For performance-critical applications, always benchmark different methods of conversion to find the most efficient approach for your specific use case.
Error Handling: Be prepared to handle potential errors, such as type mismatches or memory issues when dealing with large datasets.
In conclusion, converting a Python array
to a list
is simple, but understanding the implications and choosing the right method can significantly impact your code's performance and maintainability. Whether you're dealing with small datasets or processing large amounts of data, these insights and examples should guide you in making informed decisions about your data structures and conversions.
The above is the detailed content of How can you convert a Python array to a Python list?. For more information, please follow other related articles on the PHP Chinese website!
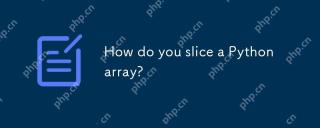
The basic syntax for Python list slicing is list[start:stop:step]. 1.start is the first element index included, 2.stop is the first element index excluded, and 3.step determines the step size between elements. Slices are not only used to extract data, but also to modify and invert lists.
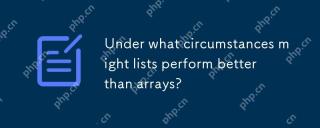
Listsoutperformarraysin:1)dynamicsizingandfrequentinsertions/deletions,2)storingheterogeneousdata,and3)memoryefficiencyforsparsedata,butmayhaveslightperformancecostsincertainoperations.
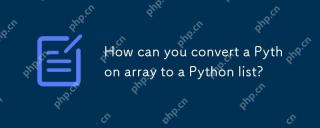
ToconvertaPythonarraytoalist,usethelist()constructororageneratorexpression.1)Importthearraymoduleandcreateanarray.2)Uselist(arr)or[xforxinarr]toconvertittoalist,consideringperformanceandmemoryefficiencyforlargedatasets.
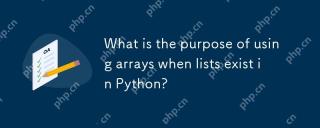
ChoosearraysoverlistsinPythonforbetterperformanceandmemoryefficiencyinspecificscenarios.1)Largenumericaldatasets:Arraysreducememoryusage.2)Performance-criticaloperations:Arraysofferspeedboostsfortaskslikeappendingorsearching.3)Typesafety:Arraysenforc
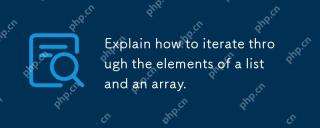
In Python, you can use for loops, enumerate and list comprehensions to traverse lists; in Java, you can use traditional for loops and enhanced for loops to traverse arrays. 1. Python list traversal methods include: for loop, enumerate and list comprehension. 2. Java array traversal methods include: traditional for loop and enhanced for loop.
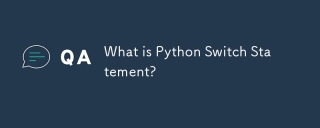
The article discusses Python's new "match" statement introduced in version 3.10, which serves as an equivalent to switch statements in other languages. It enhances code readability and offers performance benefits over traditional if-elif-el
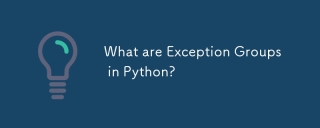
Exception Groups in Python 3.11 allow handling multiple exceptions simultaneously, improving error management in concurrent scenarios and complex operations.
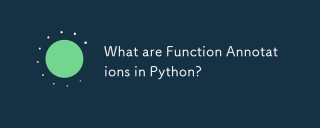
Function annotations in Python add metadata to functions for type checking, documentation, and IDE support. They enhance code readability, maintenance, and are crucial in API development, data science, and library creation.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
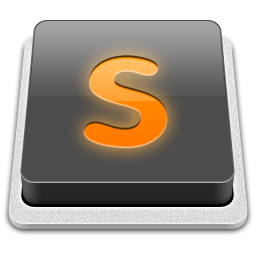
SublimeText3 Mac version
God-level code editing software (SublimeText3)
