Concurrency control in Golang and Go WaitGroup
Concurrency control in Golang and Go WaitGroup
In Golang, we can use goroutine to implement concurrent execution tasks. However, in some cases, we need to control the number of concurrent executions to avoid excessive resource consumption or concurrency contention issues. Golang provides some methods to achieve concurrency control, the most commonly used of which is to use Go WaitGroup.
Go WaitGroup is a counting semaphore used to wait for a group of goroutines to complete execution. When we start a group of goroutines, we can use WaitGroup to track the status of these goroutines and perform the next step after all goroutines are completed.
Below we use a specific code example to demonstrate concurrency control in Golang and the use of Go WaitGroup.
package main import ( "fmt" "sync" "time" ) func main() { numWorkers := 5 var wg sync.WaitGroup for i := 0; i < numWorkers; i++ { wg.Add(1) // 每启动一个goroutine,等待组加1 go worker(i, &wg) } wg.Wait() // 等待所有goroutine完成 fmt.Println("All workers have finished") } func worker(id int, wg *sync.WaitGroup) { defer wg.Done() // goroutine执行完毕,等待组减1 fmt.Printf("Worker %d started ", id) time.Sleep(time.Second) // 模拟耗时操作 fmt.Printf("Worker %d finished ", id) }
In this example, we have 5 goroutines, and the task of each execution is to call the worker function. First, we defined a WaitGroup variable wg and started 5 goroutines in the main function. Each goroutine calls the worker function and operates the WaitGroup by passing a pointer to wg.
In the worker function, we use defer wg.Done() to reduce the count of the waiting group after the goroutine completes execution. This means that each goroutine will call the Done() function to notify the WaitGroup when it is completed. Then, we added some simulated time-consuming operations to each worker function to observe the effect of concurrent execution.
In the main function, we call wg.Wait() to wait for all goroutines to complete. This will cause the main function to block after all goroutines have finished executing, until the WaitGroup count reaches zero.
Run the above code, you will see output similar to the following:
Worker 0 started Worker 1 started Worker 2 started Worker 3 started Worker 4 started Worker 3 finished Worker 2 finished Worker 0 finished Worker 1 finished Worker 4 finished All workers have finished
As can be seen from the output, all goroutines start and run in a concurrent manner, and are notified after completion main function.
By using Go WaitGroup, we can easily control the number of concurrent executions and perform subsequent operations after all goroutines are completed. This is very helpful for handling large-scale concurrent tasks or limiting resource consumption.
To sum up, concurrency control and Go WaitGroup in Golang are important tools for implementing concurrent programming. We can use WaitGroup to track and control the execution of a group of goroutines to ensure the correctness and stability of concurrent operations. In this way, we can better utilize multi-core processors and resources and improve program execution efficiency.
The above is the detailed content of Concurrency control in Golang and Go WaitGroup. For more information, please follow other related articles on the PHP Chinese website!
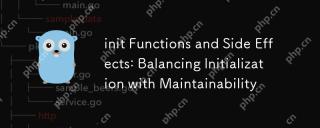
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
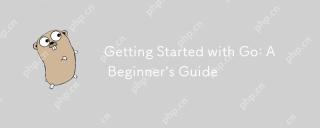
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
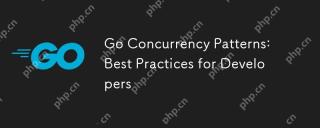
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
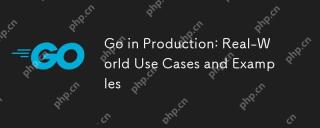
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
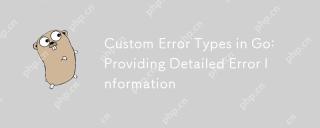
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
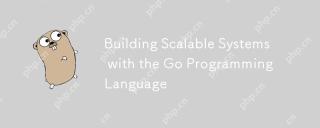
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
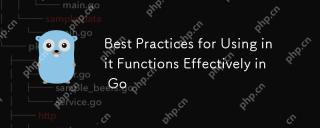
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
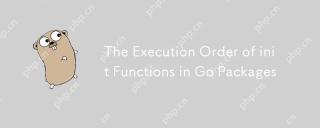
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
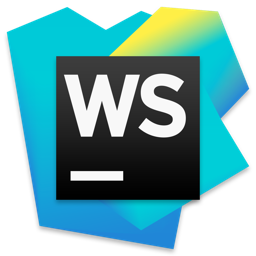
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
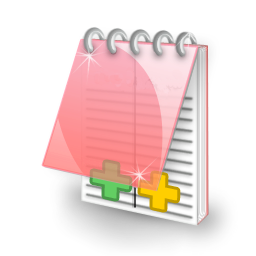
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
