


Architecture Analysis: Application of Go WaitGroup in Distributed Systems
Introduction:
In modern distributed systems, in order to improve the performance and throughput of the system Volume often requires the use of concurrent programming techniques to handle a large number of tasks. As a powerful concurrent programming language, Go language is widely used in the development of distributed systems. Among them, WaitGroup is an important concurrency primitive provided by the Go language, which is used to wait for the completion of a group of concurrent tasks. This article will start from the perspective of distributed systems, explore the application of Go WaitGroup in distributed systems, and provide specific code examples.
- What is Go WaitGroup?
Go WaitGroup is a concurrency primitive provided in the sync package of the Go language standard library, which is used to wait for the completion of a group of concurrent tasks. Its main function is for the main thread to wait for a set of subtasks to complete before continuing execution. In distributed systems, a large number of concurrent tasks often need to be processed. Using WaitGroup can easily manage and control the concurrent execution of these tasks. - Basic usage of Go WaitGroup
In the Go language, using WaitGroup requires the following steps:
(1) First create a WaitGroup object, which can be created by calling the New() function of WaitGroup corresponding object.
(2) Then use the Add() method to increase the number of tasks that need to be waited for. This number is the number of concurrent tasks.
(3) Then call the Done() method of the Add() method at the starting position of each task to indicate that the task has been completed.
(4) Finally, call the Wait() method in the main thread to wait for the completion of all tasks.
The following is a specific code example:
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup wg.Add(5) // 增加任务数量为5,即有5个并发任务 for i := 0; i < 5; i++ { go func(i int) { defer wg.Done() // 表示当前任务已经完成 // 执行具体的任务 fmt.Printf("Task %d executing ", i) }(i) } wg.Wait() // 等待所有任务完成 fmt.Println("All tasks completed") }
In the above code example, we created a WaitGroup object and added 5 tasks. Then a loop is used to create 5 concurrent tasks, and the specific logic of each task is implemented in an anonymous function. At the beginning of each task, we call the Done() method of the Add() method to indicate that the task has been completed. Finally, the Wait() method is called in the main thread to wait for the completion of all tasks. During the execution of the task, we can add arbitrary logic code.
- Application of Go WaitGroup in distributed systems
In distributed systems, it is often necessary to process a large number of tasks concurrently, such as concurrently grabbing data from multiple remote servers, and then Process and analyze. In this case, using WaitGroup can easily manage and control the concurrent execution of these tasks.
For example, we can capture data from multiple remote servers concurrently, then wait for the completion of all tasks in the main thread, and finally process and analyze the data. Call the Add() method at the beginning of each task to increase the number of tasks, and call the Done() method at the end of the task to indicate task completion. The main thread calls the Wait() method to wait for the completion of all tasks.
The specific code examples are as follows:
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup servers := []string{"serverA", "serverB", "serverC"} for _, server := range servers { wg.Add(1) // 增加任务数量 go func(server string) { defer wg.Done() // 表示当前任务已经完成 // 从远程服务器上抓取数据 data := fetchDataFromRemoteServer(server) // 处理和分析数据 processData(data) }(server) } wg.Wait() // 等待所有任务完成 fmt.Println("All tasks completed") } func fetchDataFromRemoteServer(server string) string { // 实现从远程服务器上抓取数据的逻辑 return fmt.Sprintf("Data from %s", server) } func processData(data string) { // 实现数据处理和分析的逻辑 fmt.Println("Processing data:", data) }
In the above code examples, we use WaitGroup to manage and control the execution of concurrent tasks. Indicate task completion by increasing the number of tasks and then calling the Done() method at the beginning of each task. The main thread calls the Wait() method to wait for the completion of all tasks. In the implementation of each task, we can capture, process and analyze data according to specific business needs.
Conclusion:
This article discusses the application of Go WaitGroup in distributed systems from the perspective of distributed systems, and provides specific code examples. By using WaitGroup, we can easily manage and control the execution of concurrent tasks and improve the performance and throughput of distributed systems. In actual applications, the functions of WaitGroup can be flexibly used and expanded according to specific needs and business logic to meet the needs of distributed systems. In concurrent programming, mastering the skills of using WaitGroup is of great significance for developing high-performance and highly scalable distributed systems.
The above is the detailed content of Architecture Analysis: Application of Go WaitGroup in Distributed Systems. For more information, please follow other related articles on the PHP Chinese website!
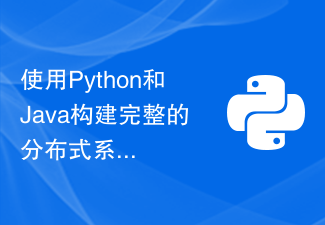
随着云计算和大数据技术的发展,分布式系统的应用越来越广泛,尤其是在企业级应用中。构建分布式系统可以提高系统的可伸缩性和容错性,使得系统更加稳定和可靠。在本文中,我们将介绍如何使用Python和Java构建一个完整的分布式系统。分布式系统通常由多个计算节点组成,这些节点可以是不同的计算机或者是运行在不同进程中的程序。这些节点之间通过通信协议进行通信,协同完成任
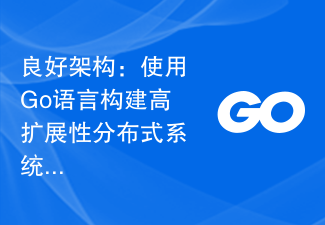
作为一款高性能的编程语言,Go语言在分布式系统的建设中非常流行。它的高速度和极低的延迟时间让开发人员更加容易实现高扩展性的分布式架构。在构建分布式系统前,需考虑的架构问题非常繁琐。如何设计出更加易于维护、可扩展和稳定的架构是所有分布式系统开发者面临的重要问题。使用Go语言来构建分布式系统,可以使这些架构选择变得更加简单和明晰。高效的协程Go语言天生支持协程,
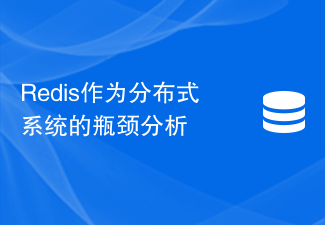
Redis作为一个开源的基于内存的键值存储系统,正被越来越多的企业使用于其分布式系统中,因为其高性能、可靠性和灵活性。但是,在一些情况下,Redis作为分布式系统中的瓶颈,可能会影响系统的整体性能。本文将探讨Redis在分布式系统中的瓶颈原因及其解决方法。Redis中的单线程模型Redis采用的是单线程模型,这意味着一个Redis实例只能够处理一条命令,即使
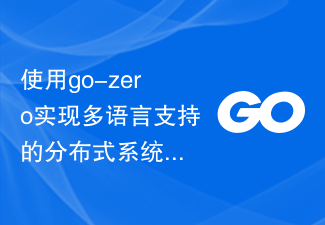
在当今全球化的时代,多语言支持的分布式系统已经成为许多企业的必要需求。为了实现多语言支持,开发人员需要在系统中处理不同的语言翻译和本地化问题。但是,很多人往往会遇到一系列的挑战,如何管理海量的本地化内容,如何快速切换语言、如何高效地管理翻译人员等等。这些问题对于开发系统来说非常具有挑战性和复杂性。在这样的情况下,使用go-zero这个高性能微服务框架来搭建多
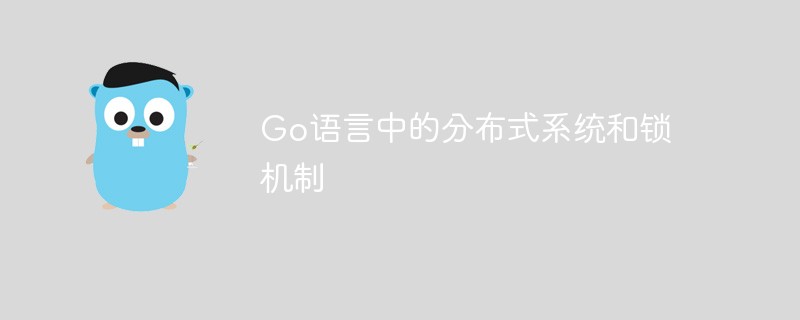
随着互联网的不断发展,分布式系统已经成为了应用领域中的热点话题之一。在分布式系统中,锁机制是一个重要的问题,特别是在涉及到并发的应用场景中,锁机制的效率和正确性越来越受到人们的重视。在这篇文章中,我们将介绍Go语言中的分布式系统和锁机制。分布式系统Go语言是一种开源的、现代的编程语言,具有高效、简洁、易于学习和使用等特点,在工程师团队中已经得到了广泛的应用和
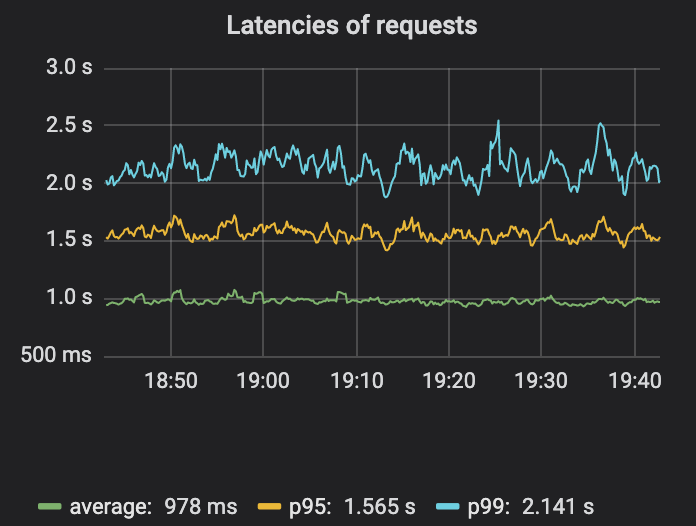
本文是Uber的工程师GergelyOrosz的文章,原文地址在:https://blog.pragmaticengineer.com/operating-a-high-scale-distributed-system/在过去的几年里,我一直在构建和运营一个大型分布式系统:优步的支付系统。在此期间,我学到了很多关于分布式架构概念的知识,并亲眼目睹了高负载和高可用性系统运行的挑战(一个系统远远不是开发完了就完了,线上运行的挑战实际更大)。构建系统本身是一项有趣的工作。规划系统如何处理10x/100
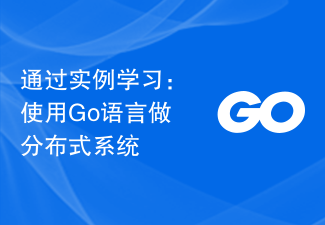
在当今互联网高速发展的背景下,分布式系统已经成为了大型企业和组织中不可或缺的一部分。而作为一门高效、强大且易于理解的编程语言,Go语言已经成为了开发分布式系统的首选语言之一。在本文中,我们将通过实例学习如何使用Go语言开发分布式系统。第一步:理解分布式系统在深入学习Go语言之前,我们需要理解什么是分布式系统。简单来说,分布式系统是由多个独立的计算机节点组成,
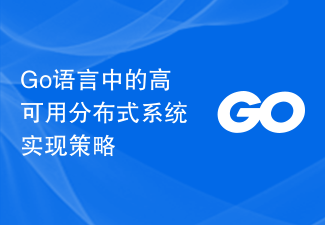
如何在Go语言开发中实现高可用的分布式系统摘要:随着互联网的快速发展,分布式系统的需求越来越大。如何在Go语言开发中实现高可用的分布式系统成为了一个重要的问题。本文将介绍如何使用Go语言开发高可用的分布式系统。一、介绍分布式系统是由多个独立的节点组成的,节点之间通过网络进行通信和协调。高可用是分布式系统的核心要求之一,它能够保证系统在面对各种异常和故障时仍能


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
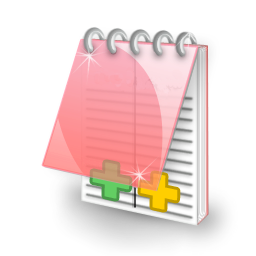
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
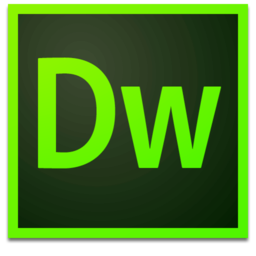
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
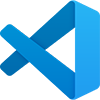
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
