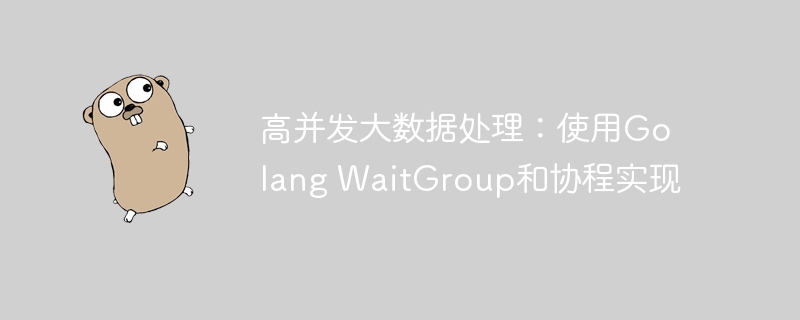
Highly concurrent big data processing: using Golang WaitGroup and coroutine implementation
Abstract: In today's information age, big data processing has become an important issue for various enterprises and organizations. core needs. In order to achieve high-concurrency big data processing, using Golang's WaitGroup and coroutines is an efficient and simple method. This article will introduce how to use Golang's WaitGroup and coroutines to implement high-concurrency big data processing, and attach specific code examples.
Keywords: high concurrency, big data processing, Golang, WaitGroup, coroutine
- Introduction
Nowadays, with the rapid development of Internet technology, big data has become The core needs of all walks of life. Applications that handle big data need to have high concurrency capabilities in order to be able to process large amounts of data efficiently. In the context of this growing demand, using Golang's WaitGroup and coroutines can help us achieve high-concurrency big data processing.
- Golang WaitGroup
Golang's WaitGroup is a synchronization primitive that can be used to wait for the completion of a group of coroutines. When we start a group of coroutines, we can wait for the completion of these coroutines through WaitGroup to ensure that all coroutines have been executed before continuing to perform other operations. WaitGroup has three main methods: Add(), Done() and Wait().
- Add(): Add the number of coroutines to wait to WaitGroup through the Add() method;
- Done(): Notify WaitGroup of one through the Done() method The coroutine has been completed;
- Wait(): Wait for the completion of all coroutines added to the WaitGroup through the Wait() method.
- Coroutine
Coroutine is a lightweight thread that can run on an independent stack and is managed by a user-mode scheduler. In Golang, we can easily use the keyword go to start a coroutine. The startup of the coroutine will not block the main thread and tasks can be executed concurrently. This allows us to efficiently process large amounts of data in a concurrent manner.
- Use Golang WaitGroup and coroutines to implement high-concurrency big data processing
Below we will use an example to show how to use Golang's WaitGroup and coroutines to implement high-concurrency big data processing.
package main
import (
"fmt"
"sync"
)
func processData(data int, wg *sync.WaitGroup) {
defer wg.Done()
// 模拟数据处理过程
// 这里可以做一些复杂的计算、访问数据库等操作
result := data * 2
fmt.Printf("处理数据 %d,结果为 %d
", data, result)
}
func main() {
var wg sync.WaitGroup
// 设置要处理的数据集合
dataList := []int{1, 2, 3, 4, 5}
// 设置WaitGroup等待的协程数量
wg.Add(len(dataList))
// 启动协程进行数据处理
for _, data := range dataList {
go processData(data, &wg)
}
// 等待所有协程完成
wg.Wait()
fmt.Println("所有数据处理完成")
}
In the above code, we first define a processData()
function to simulate the data processing process. In the main function, we create a WaitGroup to wait for the completion of all coroutines. Then, we set the number of waiting coroutines through the Add()
method, and then use the keyword go to start the coroutine for data processing. Finally, wait for all coroutines to complete by calling the Wait()
method.
The above example shows how to use Golang's WaitGroup and coroutines to achieve high-concurrency big data processing. By using a WaitGroup to wait for all coroutines to complete, we can ensure that the data processing process is not interrupted and continue operations after all data processing is completed.
- Conclusion
In big data processing, achieving high concurrency is the key to improving system performance, and using Golang's WaitGroup and coroutines is an efficient and simple method. By using WaitGroup to wait for the completion of all coroutines, we can process large amounts of data with high concurrency and improve the response speed and efficiency of the system. Using Golang's WaitGroup and coroutines can make it easier for us to achieve high-concurrency big data processing needs.
Reference:
- Go Concurrency Patterns: https://blog.golang.org/concurrency-patterns
- Go Language Specification: https: //golang.org/ref/spec
(Word count: 737 words)
The above is the detailed content of Highly concurrent big data processing: implemented using Golang WaitGroup and coroutines. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn