


An in-depth analysis of the garbage collector management principles of Go language
In-depth analysis of the garbage collector management principle of Go language
Garbage Collection (Garbage Collection) is one of the common features in modern programming languages, which can automatically manage memory , avoiding the tedious work of manual memory allocation and release by developers. As a language that takes both development efficiency and performance into account, the design and implementation of Go's garbage collector (Garbage Collector) is a very important part.
The garbage collector of the Go language adopts a generational garbage collection strategy, with three generations: young, mid and old. Among them, the young generation is the object with the shortest survival time, the mid generation is the object that survived the last young generation recycling, and the old generation is the object with the longest survival time. Each generation has a specific memory size. If this size is exceeded, the garbage collector will be triggered.
In the Go language, the garbage collector uses the Three-Color Marking algorithm to mark and recycle garbage objects. Specifically, it is divided into three colors: white, gray and black, which are used to mark the survival status of objects.
First, the garbage collector marks all objects as white, indicating that they have not been scanned by the garbage collector. Then, scan starting from the root object and mark the root object in gray, indicating that it has been scanned but its child objects have not yet been marked. Next, traverse the sub-objects of the gray object one by one and mark them as gray, repeating this process until there are no more gray objects. Finally, mark all scanned objects as black, indicating that they have been fully scanned by the garbage collector and are alive objects. White objects are considered garbage objects and will be recycled and released by the garbage collector.
In the Go language, the specific code implementation of the garbage collector is completed at runtime. There is a goroutine in the runtime package that is specifically responsible for garbage collection. This goroutine will periodically trigger the garbage collection process.
The following is a sample code of the working process of the garbage collector:
package main import "time" type Object struct { data []int next *Object } func main() { var head *Object for i := 0; i < 100000; i++ { obj := &Object{ data: make([]int, 10000), next: head, } head = obj time.Sleep(time.Duration(10) * time.Millisecond) } }
The above code first defines a simple object type Object, including a data field and a next pointer pointing to the next object. . In the main function, a large number of Object objects are created through a loop, and after each object is created, the time.Sleep function is used to pause the program for a period of time to simulate the survival time of the object.
When running the above code, we can observe the garbage collection process by setting the environment variable GODEBUG=gctrace=1
. When the program starts running, the garbage collector will output a piece of debugging information about the garbage collector, including the garbage collector's strategy, heap-related information, etc. As the program runs, the garbage collector will periodically trigger the garbage collection process and output relevant log information.
In summary, the garbage collector management principle of Go language is based on generational and three-color marking. The garbage collector will periodically trigger the garbage collection process, automatically identify and recycle objects that are no longer used, and release memory resources. By gaining a deeper understanding of how the garbage collector works, developers can better optimize and debug their code and play a greater role in developing high-performance Go applications.
The above is the detailed content of An in-depth analysis of the garbage collector management principles of Go language. For more information, please follow other related articles on the PHP Chinese website!
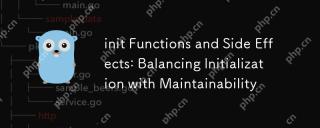
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
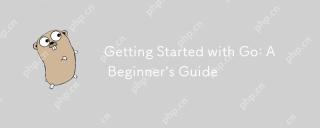
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
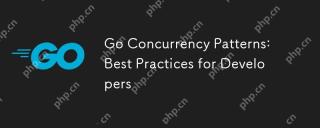
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
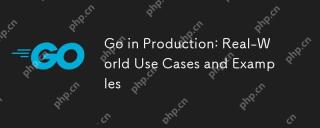
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
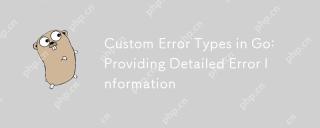
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
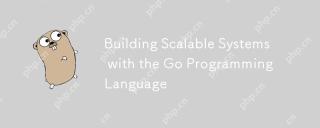
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
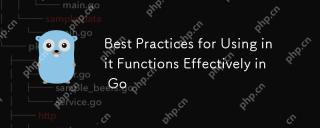
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
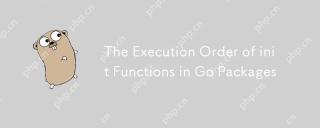
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
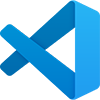
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
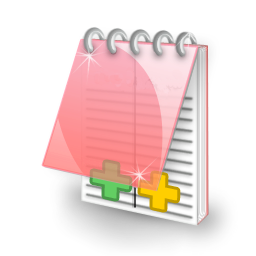
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
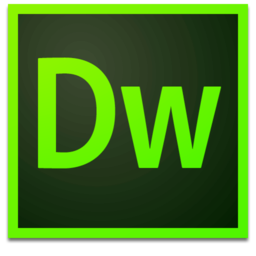
Dreamweaver Mac version
Visual web development tools
