


How to use Golang’s synchronization mechanism to improve the performance of big data processing
Abstract: With the advent of the big data era, the need to process big data is becoming more and more urgent. As a high-performance programming language, Golang's concurrency model and synchronization mechanism make it perform well in big data processing. This article will introduce how to use Golang's synchronization mechanism to improve the performance of big data processing, and provide specific code examples.
1. Introduction
With the development of technologies such as cloud computing, Internet of Things, and artificial intelligence, the scale of data is growing explosively. When dealing with big data, improving performance and efficiency is crucial. As a statically compiled language, Golang has efficient concurrency performance and lightweight threads, making it very suitable for processing big data.
2. Golang’s concurrency model
Golang adopts the CSP (Communicating Sequential Processes) concurrency model to realize communication between coroutines through goroutine and channel. Goroutines are lightweight threads that can execute on multiple cores simultaneously. Channel is a communication pipe between goroutines, used to transfer data and synchronize operations.
3. Golang’s synchronization mechanism
In big data processing, synchronization mechanism is the key. Golang provides a rich synchronization mechanism, including mutex (Mutex), read-write lock (RWMutex), condition variable (Cond), etc. By rationally using these synchronization mechanisms, big data processing performance can be improved.
- Mutex lock (Mutex)
The mutex lock is used to protect the critical section. Only one goroutine is allowed to enter the critical section for execution at the same time. When a goroutine acquires a mutex lock, other goroutines need to wait for the lock to be released. The example code for using a mutex is as follows:
import ( "sync" ) var ( mutex sync.Mutex data []int ) func appendData(num int) { mutex.Lock() defer mutex.Unlock() data = append(data, num) } func main() { for i := 0; i < 10; i++ { go appendData(i) } // 等待所有goroutine执行完毕 time.Sleep(time.Second) fmt.Println(data) }
- Read-write lock (RWMutex)
Read-write lock is used to improve concurrency performance in scenarios where there is more reading and less writing. It allows multiple goroutines to read data at the same time, but only allows one goroutine to write data. The sample code for using the read-write lock is as follows:
import ( "sync" ) var ( rwMutex sync.RWMutex data []int ) func readData() { rwMutex.RLock() defer rwMutex.RUnlock() fmt.Println(data) } func writeData(num int) { rwMutex.Lock() defer rwMutex.Unlock() data = append(data, num) } func main() { for i := 0; i < 10; i++ { if i%2 == 0 { go readData() } else { go writeData(i) } } // 等待所有goroutine执行完毕 time.Sleep(time.Second) }
- Condition variable (Cond)
Condition variable is used to wake up the waiting goroutine when a certain condition is met. It enables more fine-grained collaboration between goroutines. The example code for using condition variables is as follows:
import ( "sync" ) var ( cond sync.Cond data []int notify bool ) func readData() { cond.L.Lock() for !notify { cond.Wait() } defer cond.L.Unlock() fmt.Println(data) } func writeData(num int) { cond.L.Lock() defer cond.L.Unlock() data = append(data, num) notify = true cond.Broadcast() } func main() { cond.L = &sync.Mutex{} for i := 0; i < 10; i++ { if i%2 == 0 { go readData() } else { go writeData(i) } } // 等待所有goroutine执行完毕 time.Sleep(time.Second) }
4. Summary
Big data processing faces the challenges of massive data and high concurrency. Using Golang’s concurrency model and synchronization mechanism can improve processing performance. This article introduces Golang's concurrency model and common synchronization mechanisms, including mutex locks, read-write locks, and condition variables, and provides corresponding sample code. Proper use of these synchronization mechanisms can give full play to Golang's concurrency advantages and improve the performance and efficiency of big data processing.
The above is the detailed content of How to use Golang's synchronization mechanism to improve the performance of big data processing. For more information, please follow other related articles on the PHP Chinese website!
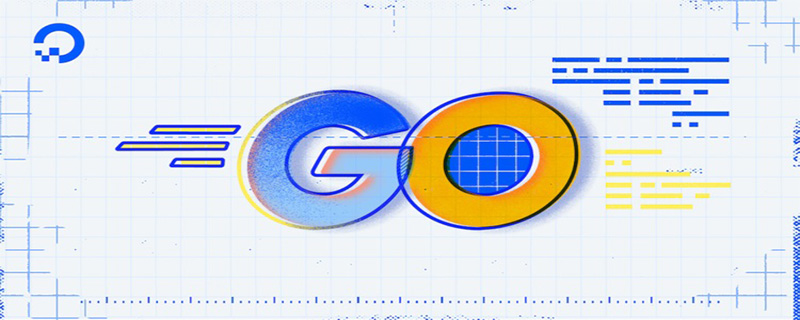
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
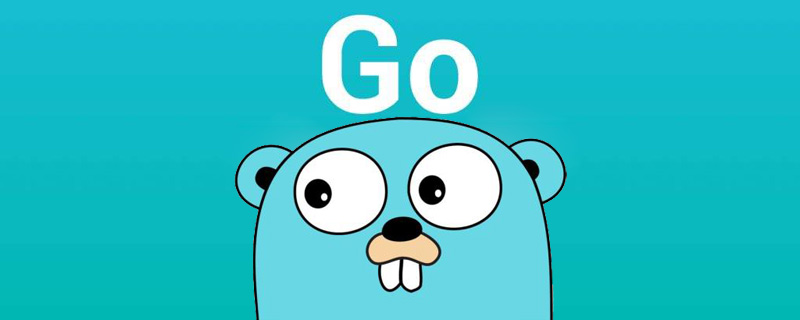
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
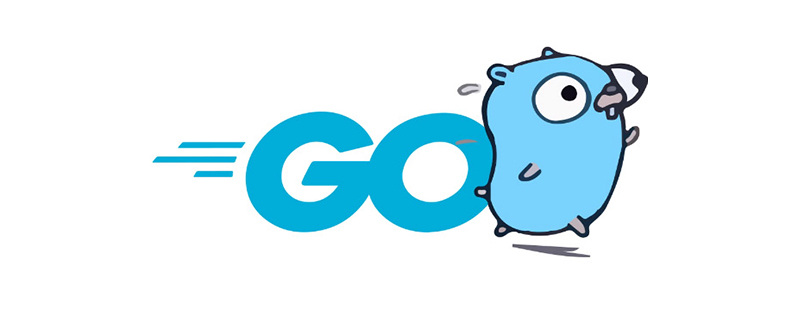
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
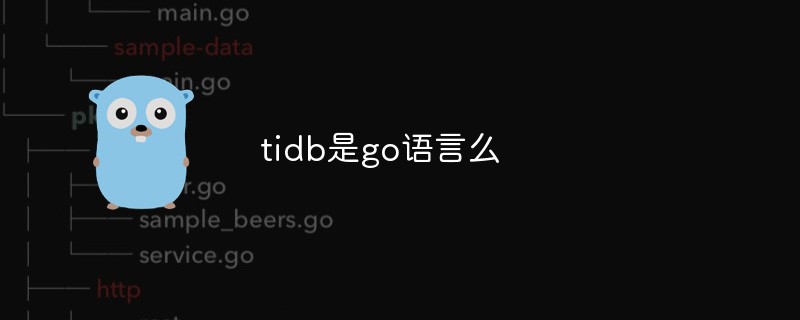
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
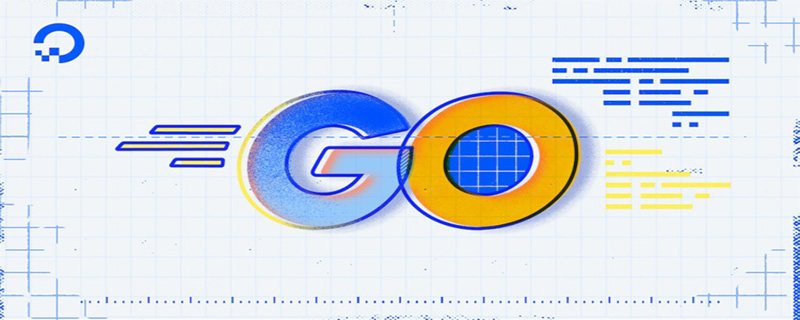
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
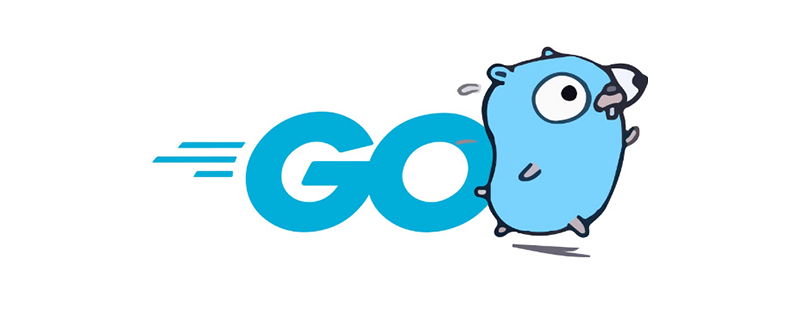
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
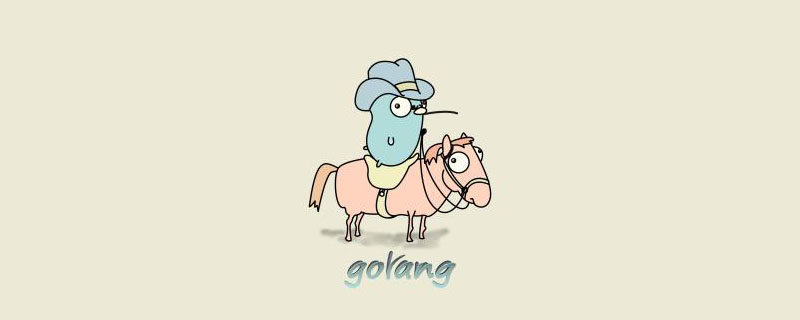
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
