


Realize highly concurrent Select Channels Go programming technology and Golang
Introduction:
In the current highly concurrent programming environment, being able to effectively handle high concurrent requests is very important. The emergence of Golang gives developers a powerful tool to meet this challenge. This article will explore how to use Select Channels programming technology in Golang to achieve highly concurrent processing and provide specific code examples.
1. Select Channels in Golang
Channels in Golang are a synchronization mechanism used for communication between goroutines. By using channels, multiple coroutines can safely pass and receive data. Select Channels is a structure for selecting between multiple channels, similar to switch statements in other programming languages.
In Golang, you can use the Select statement to combine multiple channels to achieve high concurrency processing. The Select statement waits for any one of multiple channels to be ready and executes the corresponding code block. This mechanism allows us to implement non-blocking processing between different channels and improves the concurrency performance of the program.
2. Implementing Highly Concurrent Select Channels Go Programming Technology
The following is a sample code that uses Select Channels to achieve high concurrency:
package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(time.Second * 1) ch1 <- "Hello from goroutine 1" }() go func() { time.Sleep(time.Second * 2) ch2 <- "World from goroutine 2" }() for i := 0; i < 2; i++ { select { case msg1 := <-ch1: fmt.Println(msg1) case msg2 := <-ch2: fmt.Println(msg2) } } }
In the above example, we created two Channels ch1
and ch2
, and send data to the channels in two anonymous functions respectively. The first anonymous function will send data to ch1
after 1 second, and the second anonymous function will send data to ch2
after 2 seconds.
In the main function, we use the Select statement to monitor two channels. Select will wait for the data in ch1
or ch2
to be ready first, and then execute the corresponding code block. Finally, the Select statement is repeatedly executed in the for
loop until the data in both channels is obtained.
By using Select Channels programming technology, we can achieve highly concurrent processing. Multiple coroutines can interact with different channels at the same time, improving the concurrency performance of the program.
Conclusion:
This article introduces how to use the Select Channels programming technology in Golang to achieve highly concurrent processing. By using the Select statement to combine multiple channels, we can achieve non-blocking processing between different channels and improve the concurrency performance of the program. Through concrete code examples, we show how to use Select Channels in Golang to achieve a high degree of concurrency. I hope this article can help readers better understand and apply Select Channels programming technology.
The above is the detailed content of Implementing highly concurrent Select Channels Go programming technology and golang. For more information, please follow other related articles on the PHP Chinese website!
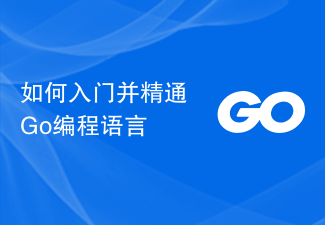
如何入门并精通Go编程语言Go语言是一种由Google开发的开源编程语言,它具有高效、简洁、并发等特点,在近年来受到越来越多开发者的喜爱。对于想要学习和精通Go语言的人来说,本文将提供一些入门和深入学习的建议,并配以具体代码示例,希望能够帮助读者更好地掌握这门语言。一、入门阶段安装Go语言首先,要学习Go语言,你需要在你的计算机上安装Go编译器。可以在官方网
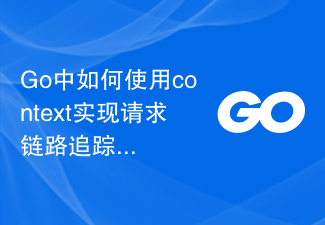
Go中如何使用context实现请求链路追踪在微服务的架构中,请求链路追踪是一种非常重要的技术,用于追踪一个请求在多个微服务之间的传递和处理情况。在Go语言中,我们可以使用context包来实现请求链路追踪,本文将介绍如何使用context进行请求链路追踪,并给出代码示例。首先,我们需要了解一下context包的基本概念和用法。context包提供了一种机制
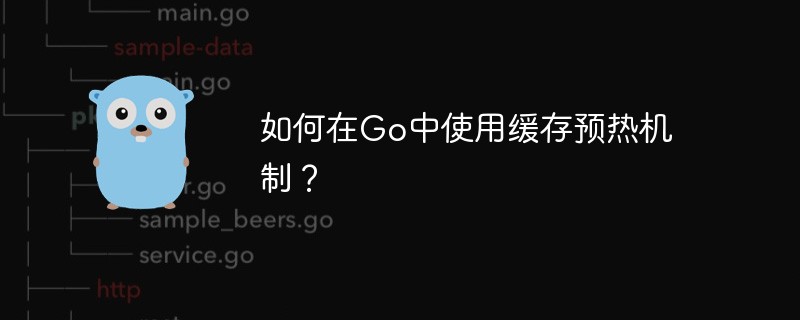
随着互联网技术的飞速发展,越来越多的应用场景需要高效的缓存机制来提高系统的性能和响应速度。在Go语言中,有许多优秀的缓存库,如go-cache、groupcache等,但是如何结合预热机制来使用缓存,是一个值得探讨的话题。本文将介绍什么是预热机制、为什么需要预热机制、Go语言缓存库中的预热机制实现以及如何应用预热机制来提高缓存的使用效率。一、什么是预热机制?
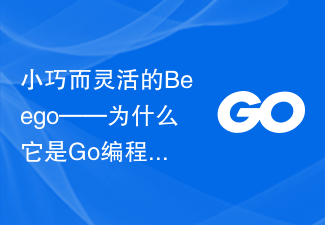
Go语言在近年来成为了云计算、大数据与人工智能等领域开发者们的首选语言之一。Go语言的简洁、高效并具有强大的并发性能和优秀的网络编程特性,同时也是一个快速迭代开发的语言,这让它成为一个越来越受欢迎的编程语言。然而,仅仅依靠Go语言本身并不能充分发挥其潜力。当我们面对多个线程,多个模块,多个协程和复杂的业务逻辑时,我们需要一个好的框架来协助我们完成我们的开发。
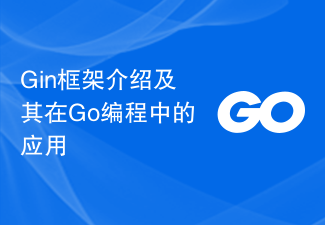
Gin框架是一个轻量级的Go语言Web开发框架,它可以快速构建高性能的Web应用程序。与其他Web框架相比,Gin框架具有许多独特的特点和优势。本文将介绍Gin框架的特点、优势以及在Go编程中的应用。一、Gin框架的特点1.简单易用Gin框架是一个非常简单易用的Web框架,它不需要学习太多的Web开发知识和技能。即使是对Go语言不熟悉的开发者,也可以很快上手
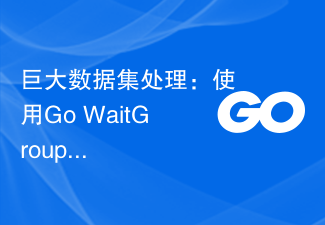
巨大数据集处理:使用GoWaitGroup优化性能引言:随着技术的不断发展,数据量的增长是不可避免的。在处理巨大数据集时,性能优化变得尤为重要。本文将介绍如何使用Go语言中的WaitGroup来优化巨大数据集的处理。了解WaitGroupWaitGroup是Go语言中的一个并发原语,它可以用于协调多个goroutine的执行。WaitGroup有三个方法:
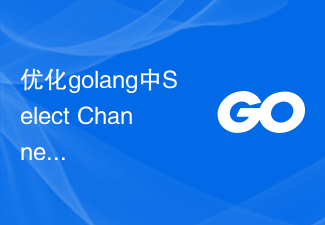
优化golang中SelectChannelsGo并发式编程的性能调优策略引言:随着现代计算机处理器的多核心和并行计算能力的提高,Go语言作为一门并发式编程语言,被广泛采用来开发高并发的后端服务。在Go语言中,使用goroutine和channel可以轻松实现并发编程,提高程序的性能和响应速度。而在并发编程中,使用select语句与channel配合使用
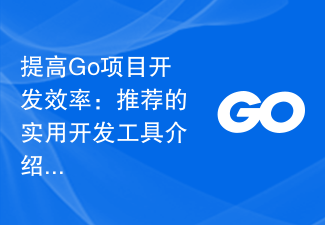
高效开发Go语言项目:值得尝试的实用开发工具介绍Go语言作为一种高效、简洁的编程语言,受到越来越多开发者的青睐。然而,在实际项目开发中,有时候我们可能会遇到一些繁琐的工作,这就需要借助一些实用的开发工具来提高开发效率。本文将介绍几款值得尝试的实用开发工具,并附上具体的代码示例,希望能帮助开发者在Go语言项目开发中更加高效地工作。1.GoLandGoLand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
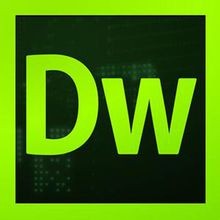
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
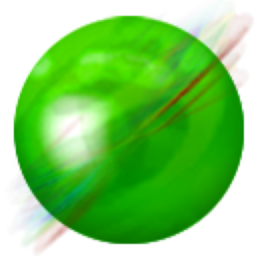
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
