


How to use PHP microservices to implement distributed message queues and communication
Introduction:
With the rapid development of Internet applications, there is a need for large-scale distributed systems More and more urgent. Distributed systems can improve system availability, scalability, and performance. One of the important components is the message queue and communication mechanism. This article will introduce how to use PHP microservice architecture to implement distributed message queues and communication, and provide specific code examples.
1. What is microservice architecture
Microservice architecture is an architectural design pattern that splits applications into small, independently running services. Each service can be deployed, expanded and managed independently, and services communicate through lightweight communication mechanisms. Microservice architecture can provide better maintainability, scalability and reliability.
2. Distributed message queue
Distributed message queue is a mechanism used for asynchronous communication in distributed systems. It enables decoupling, resilience and reliability. Messages in the message queue can be consumed by different services, allowing different services to work together in a loosely coupled manner. Commonly used distributed message queues include Kafka, RabbitMQ, etc.
- Installing RabbitMQ
First, you need to install RabbitMQ. You can download and install RabbitMQ through the official website. For specific installation steps, please refer to the official documentation. - Create producers and consumers
Next create a producer and a consumer. The sample code is as follows:
require_once __DIR__.' /vendor/autoload.php';
use PhpAmqpLibConnectionAMQPStreamConnection;
use PhpAmqpLibMessageAMQPMessage;
// Producer
$connection = new AMQPStreamConnection('localhost', 5672, 'guest ', 'guest');
$channel = $connection->channel();
$channel->queue_declare('hello', false, false, false, false);
$msg = new AMQPMessage('Hello World!');
$channel->basic_publish($msg, '', 'hello');
echo " [x] Sent 'Hello World!'
";
$channel->close();
$connection->close();
// Consumer
$connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest');
$channel = $connection->channel();
$channel->queue_declare(' hello', false, false, false, false);
echo " [*] Waiting for messages. To exit press CTRL C
";
$callback = function ($msg) {
echo ' [x] Received ', $msg->body, "
";
};
$channel->basic_consume('hello', '', false, true, false, false, $callback);
while ($channel->is_consuming()) {
$channel->wait();
}
? >
- Run producers and consumers
Run the code of producers and consumers in the command line:
php producer.php
php consumer.php
The producer will send a message to the queue, and the consumer will receive and print the message. You can test the message distribution mechanism by running the consumer multiple times.
3. Microservice communication
In the microservice architecture, services need to communicate with each other to work together. Commonly used microservice communication methods include HTTP, RPC, message queue, etc.
- Communicate using HTTP
HTTP is a commonly used microservice communication protocol that can communicate through HTTP requests and responses. Common PHP HTTP libraries include Guzzle, Symfony HTTP Client, etc. The sample code is as follows:
require 'vendor/autoload.php';
use GuzzleHttpClient;
$client = new Client( );
$response = $client->request('GET', 'https://example.com');
echo $response->getBody();
?>
- Use RPC communication
RPC (Remote Procedure Call) is a communication protocol used in distributed systems. It allows different services to communicate by calling functions. Common PHP RPC libraries include gRPC, Thrift, etc. The sample code is as follows:
require_once 'vendor/autoload.php';
use HelloworldHelloRequest;
use HelloworldHelloResponse;
use HelloworldGreeterClient;
$client = new GreeterClient('localhost:50051', [
'credentials' => GrpcChannelCredentials::createInsecure(),
]);
$request = new HelloRequest();
$request-> ;setName('World');
$response = $client->SayHello($request);
echo $response->getMessage();
?>
- Communication using message queues
In distributed systems, using message queues for communication can achieve decoupling, resilience, and reliability. For sample code, please refer to the distributed message queue example in the previous section.
Conclusion:
PHP microservice architecture can achieve asynchronous communication in distributed systems by using message queues and communication mechanisms. Through the sample code, we can understand how to use PHP microservices to implement distributed message queues and communication. These technologies can improve system reliability and performance and provide an effective solution for the development of distributed systems.
The above is the detailed content of How to implement distributed message queues and communication using PHP microservices. For more information, please follow other related articles on the PHP Chinese website!
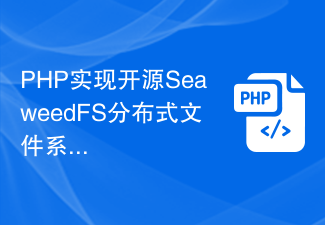
在分布式系统的架构中,文件管理和存储是非常重要的一部分。然而,传统的文件系统在应对大规模的文件存储和管理时遇到了一些问题。为了解决这些问题,SeaweedFS分布式文件系统被开发出来。在本文中,我们将介绍如何使用PHP来实现开源SeaweedFS分布式文件系统。什么是SeaweedFS?SeaweedFS是一个开源的分布式文件系统,它用于解决大规模文件存储和
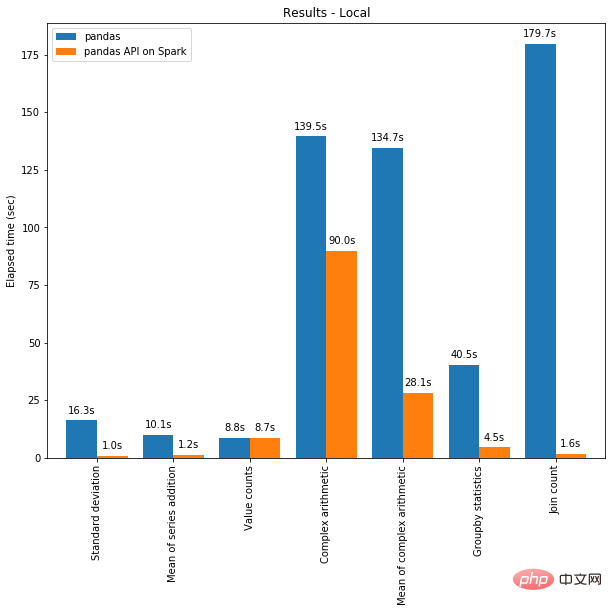
使用Python做数据处理的数据科学家或数据从业者,对数据科学包pandas并不陌生,也不乏像云朵君一样的pandas重度使用者,项目开始写的第一行代码,大多是importpandasaspd。pandas做数据处理可以说是yyds!而他的缺点也是非常明显,pandas只能单机处理,它不能随数据量线性伸缩。例如,如果pandas试图读取的数据集大于一台机器的可用内存,则会因内存不足而失败。另外pandas在处理大型数据方面非常慢,虽然有像Dask或Vaex等其他库来优化提升数
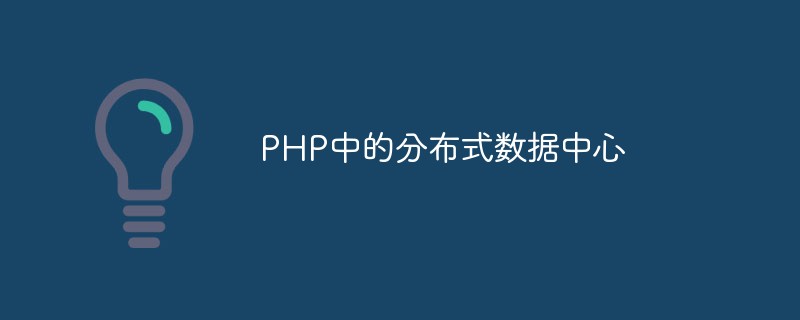
随着互联网的快速发展,网站的访问量也在不断增长。为了满足这一需求,我们需要构建高可用性的系统。分布式数据中心就是这样一个系统,它将各个数据中心的负载分散到不同的服务器上,增加系统的稳定性和可扩展性。在PHP开发中,我们也可以通过一些技术实现分布式数据中心。分布式缓存分布式缓存是互联网分布式应用中最常用的技术之一。它将数据缓存在多个节点上,提高数据的访问速度和
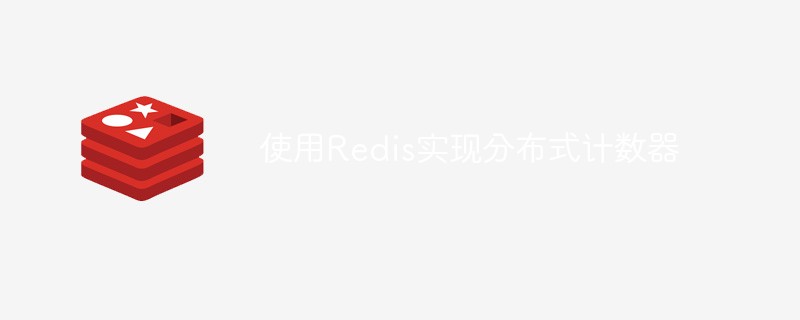
什么是分布式计数器?在分布式系统中,多个节点之间需要对共同的状态进行更新和读取,而计数器是其中一种应用最广泛的状态之一。通俗地讲,计数器就是一个变量,每次被访问时其值就会加1或减1,用于跟踪某个系统进展的指标。而分布式计数器则指的是在分布式环境下对计数器进行操作和管理。为什么要使用Redis实现分布式计数器?随着分布式计算的普及,分布式系统中的许多细节问题也
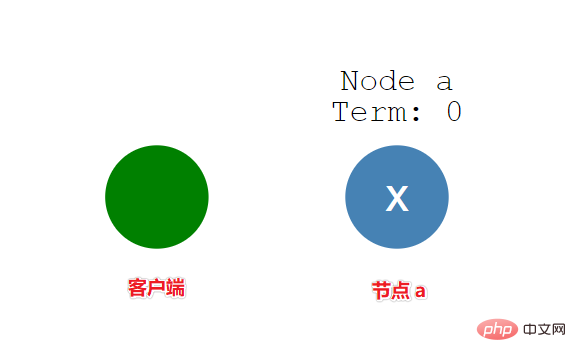
一、Raft 概述Raft 算法是分布式系统开发首选的共识算法。比如现在流行 Etcd、Consul。如果掌握了这个算法,就可以较容易地处理绝大部分场景的容错和一致性需求。比如分布式配置系统、分布式 NoSQL 存储等等,轻松突破系统的单机限制。Raft 算法是通过一切以领导者为准的方式,实现一系列值的共识和各节点日志的一致。二、Raft 角色2.1 角色跟随者(Follower):普通群众,默默接收和来自领导者的消息,当领导者心跳信息超时的
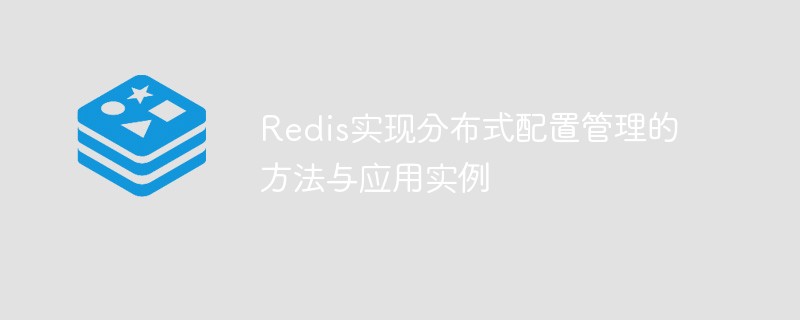
Redis实现分布式配置管理的方法与应用实例随着业务的发展,配置管理对于一个系统而言变得越来越重要。一些通用的应用配置(如数据库连接信息,缓存配置等),以及一些需要动态控制的开关配置,都需要进行统一管理和更新。在传统架构中,通常是通过在每台服务器上通过单独的配置文件进行管理,但这种方式会导致配置文件的管理和同步变得十分复杂。因此,在分布式架构下,采用一个可靠
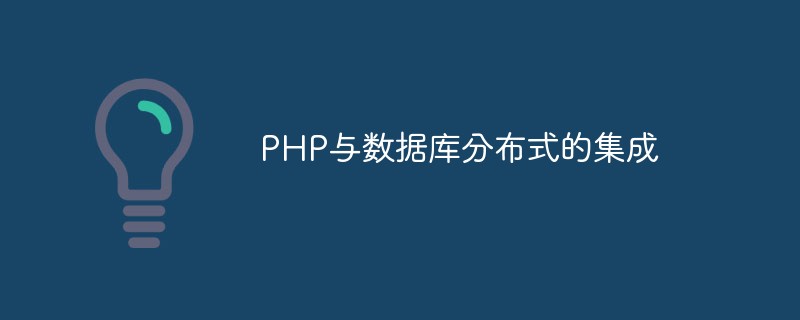
随着互联网技术的发展,对于一个网络应用而言,对数据库的操作非常频繁。特别是对于动态网站,甚至有可能出现每秒数百次的数据库请求,当数据库处理能力不能满足需求时,我们可以考虑使用数据库分布式。而分布式数据库的实现离不开与编程语言的集成。PHP作为一门非常流行的编程语言,具有较好的适用性和灵活性,这篇文章将着重介绍PHP与数据库分布式集成的实践。分布式的概念分布式
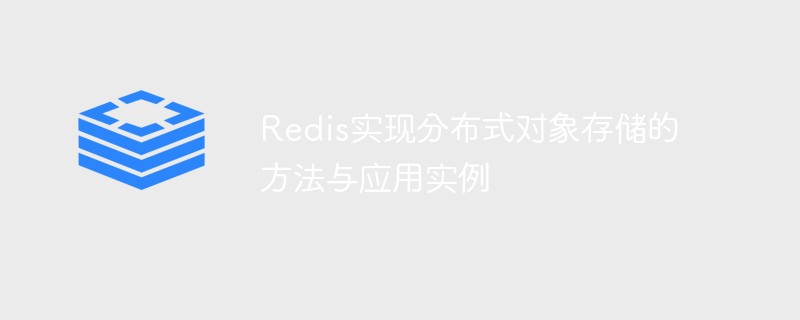
Redis实现分布式对象存储的方法与应用实例随着互联网的快速发展和数据量的快速增长,传统的单机存储已经无法满足业务的需求,因此分布式存储成为了当前业界的热门话题。Redis是一个高性能的键值对数据库,它不仅支持丰富的数据结构,而且支持分布式存储,因此具有极高的应用价值。本文将介绍Redis实现分布式对象存储的方法,并结合应用实例进行说明。一、Redis实现分


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
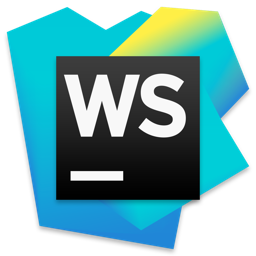
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
