Golang development: Real-time data push based on WebSockets
Golang development: Real-time data push based on WebSockets
Abstract: This article will introduce how to use Golang to develop real-time data push function based on WebSockets. First, we'll explain what WebSockets are and why you should use them for real-time data push. We then provide some Golang code examples showing how to use the Gorilla WebSocket library to develop a simple real-time data push server.
Introduction:
In Web development, real-time data push (Real-time streaming) is a very common requirement. Real-time data push allows applications to transmit data to clients in real time, and enables clients to receive and display this data in real time. The traditional HTTP request-response model is not the best choice for real-time data push because it requires the client to make frequent requests to obtain the latest data. WebSockets provides a more efficient and real-time solution.
What are WebSockets?
WebSockets is a technology provided by HTML5 for real-time two-way communication between the client and the server. It allows the server to actively push data to the client without the client making a request first. Compared with the traditional HTTP request-response model, WebSockets has the following advantages:
- The connection between the client and the server is persistent, and there is no need to frequently establish and disconnect connections, saving time Network overhead.
- The server can actively push data to the client without waiting for the client's request.
- The communication between the client and the server is full-duplex, and data can be sent and received at the same time.
Golang implements real-time data push for WebSockets:
The following code example will demonstrate how to use Golang to develop a simple real-time data push server. We will use Gorilla WebSocket library to implement WebSockets functionality.
First, we need to introduce the Gorilla WebSocket library into the project:
go get github.com/gorilla/websocket
The following is a code example of a simple Golang real-time data push server:
package main import ( "fmt" "log" "net/http" "github.com/gorilla/websocket" ) var clients = make(map[*websocket.Conn]bool) // connected clients var broadcast = make(chan Message) // broadcast channel // Message 接收和发送的数据结构体 type Message struct { Message string `json:"message"` } func main() { // 创建一个简单的文件服务器,用于向客户端提供Web页面 fs := http.FileServer(http.Dir("public")) http.Handle("/", fs) // 注册处理函数 http.HandleFunc("/ws", handleConnections) // 启动消息广播协程 go handleMessages() // 启动服务器 fmt.Println("Server started on http://localhost:8000") err := http.ListenAndServe(":8000", nil) if err != nil { log.Fatal("ListenAndServe: ", err) } } func handleConnections(w http.ResponseWriter, r *http.Request) { // 升级HTTP连接为WebSockets ws, err := websocket.Upgrade(w, r, nil, 1024, 1024) if err != nil { log.Fatal(err) } // 当前连接关闭时,从全局连接池中删除 defer func() { delete(clients, ws) ws.Close() }() // 将新的客户端连接添加到全局连接池中 clients[ws] = true for { var msg Message // 读取客户端发送的消息 err := ws.ReadJSON(&msg) if err != nil { log.Printf("error: %v", err) delete(clients, ws) break } // 将收到的消息发送到广播频道 broadcast <- msg } } func handleMessages() { for { // 从广播频道接收消息 msg := <-broadcast // 向所有客户端发送消息 for client := range clients { err := client.WriteJSON(msg) if err != nil { log.Printf("error: %v", err) client.Close() delete(clients, client) } } } }
In the above code example , we first created a simple file server to serve web pages to clients. Then, we defined a WebSocket connection processing function handleConnections
to handle new client connection requests. In this function, we add the client connection to the global connection pool and loop to read the messages sent by the client and send them to the broadcast channel.
At the same time, we also define a message broadcast function handleMessages
, which is used to receive messages from the broadcast channel and send messages to all connected clients.
Finally, we registered the handler function by calling the http.HandleFunc
function and started the server.
Conclusion:
This article introduces how to use Golang to develop a real-time data push function based on WebSockets. With the help of Gorilla WebSocket library, we can easily implement a simple real-time data push server. By using WebSockets, we can effectively achieve real-time data transmission and provide a better user experience.
Appendix:
The complete sample code and related resources can be found in the following repository: [https://github.com/your-github-repository](https://github.com/your- github-repository).
The above is the detailed content of Golang development: Real-time data push based on WebSockets. For more information, please follow other related articles on the PHP Chinese website!
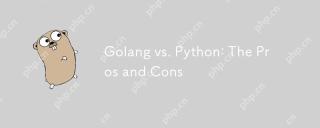
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
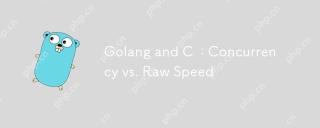
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
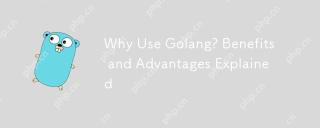
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
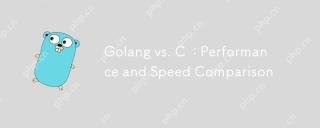
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
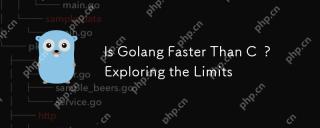
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
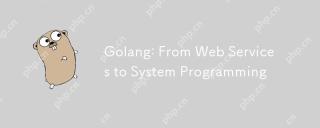
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
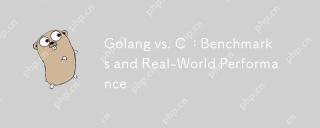
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
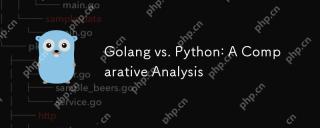
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
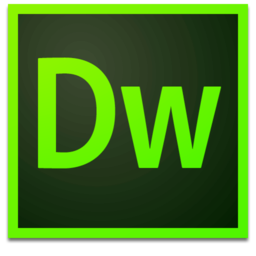
Dreamweaver Mac version
Visual web development tools
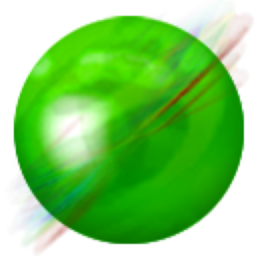
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software