How to develop distributed search functionality using Redis and PHP
How to use Redis and PHP to develop distributed search functions
Distributed search is one of the very common requirements in modern Internet applications. It can help users quickly and accurately Search for the information you need. Among them, Redis is a very fast and flexible in-memory database, while PHP is a scripting language widely used in web development. This article will introduce how to use Redis and PHP to develop distributed search functions and provide detailed code examples.
- Install Redis and PHP extensions
First, you need to install Redis and PHP extensions on the server to ensure that Redis functions can be used normally. For the installation of Redis, you can refer to the official documentation or corresponding tutorials. PHP extensions can be installed through package managers (such as apt, yum, etc.) or through source code compilation and installation. - Design search index structure
Before using Redis for distributed search, you need to design the data structure of the search index. A common approach is to use a Sorted Set to store the index and a Hash to store the details of each document. It can be designed in the following way:
索引: ZADD index:<关键词> <权重> <文档ID> 文档: HMSET doc:<文档ID> title <标题> content <内容>
Among them,
- Build a search index
Before conducting a search, you first need to establish a search index in Redis. You can first store the content, titles and other information of all documents in Redis, and create appropriate indexes for each document.
// 获取文档列表 $documents = [/* 文档列表 */]; // 遍历文档列表 foreach ($documents as $document) { // 生成文档ID $docId = $document['id']; // 将文档信息存储为Hash $redis->hMSet("doc:$docId", [ 'title' => $document['title'], 'content' => $document['content'] ]); // 对文档进行分词,并将分词结果存储到索引中 $keywords = /* 对文档进行分词处理 */; foreach ($keywords as $keyword) { $redis->zAdd("index:$keyword", $document['weight'], $docId); } }
The above code traverses the document list, stores each document as a Redis hash, performs word segmentation on each document, and stores the word segmentation results in the corresponding index.
- Execute search function
After the search index is established, you can search. You can find the matching document ID from the index based on the keywords entered by the user, and obtain the detailed information of the document based on the document ID.
// 获取用户输入的关键词 $keyword = /* 用户输入的关键词 */; // 根据关键词从索引中获取文档ID列表 $documentIds = $redis->zRangeByLex("index:$keyword", '-', '+'); // 根据文档ID获取文档的详细信息 $documents = []; foreach ($documentIds as $docId) { $documents[] = $redis->hGetAll("doc:$docId"); } // 对搜索结果进行展示 foreach ($documents as $document) { /* 对搜索结果进行展示的逻辑 */ }
The above code obtains the document ID list that matches the keyword from the index, and obtains the detailed information of the document from Redis based on the document ID. Finally, the search results can be displayed according to needs.
Summary:
By using Redis and PHP to develop distributed search functions, the speed and efficiency of search can be improved, and large-scale data storage and search can be supported. This article describes how to design the data structure of a search index, and how to build the index and perform search functions. At the same time, detailed PHP code examples are provided to facilitate developers to get started quickly. I hope this article will be helpful to readers who use Redis and PHP to develop distributed search functions.
The above is the detailed content of How to develop distributed search functionality using Redis and PHP. For more information, please follow other related articles on the PHP Chinese website!
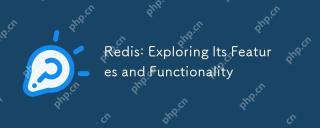
Redis stands out because of its high speed, versatility and rich data structure. 1) Redis supports data structures such as strings, lists, collections, hashs and ordered collections. 2) It stores data through memory and supports RDB and AOF persistence. 3) Starting from Redis 6.0, multi-threaded I/O operations have been introduced, which has improved performance in high concurrency scenarios.
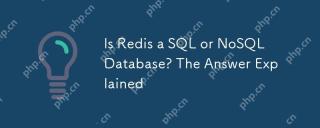
RedisisclassifiedasaNoSQLdatabasebecauseitusesakey-valuedatamodelinsteadofthetraditionalrelationaldatabasemodel.Itoffersspeedandflexibility,makingitidealforreal-timeapplicationsandcaching,butitmaynotbesuitableforscenariosrequiringstrictdataintegrityo
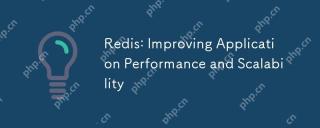
Redis improves application performance and scalability by caching data, implementing distributed locking and data persistence. 1) Cache data: Use Redis to cache frequently accessed data to improve data access speed. 2) Distributed lock: Use Redis to implement distributed locks to ensure the security of operation in a distributed environment. 3) Data persistence: Ensure data security through RDB and AOF mechanisms to prevent data loss.
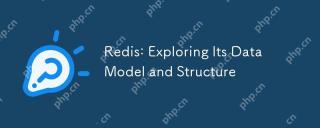
Redis's data model and structure include five main types: 1. String: used to store text or binary data, and supports atomic operations. 2. List: Ordered elements collection, suitable for queues and stacks. 3. Set: Unordered unique elements set, supporting set operation. 4. Ordered Set (SortedSet): A unique set of elements with scores, suitable for rankings. 5. Hash table (Hash): a collection of key-value pairs, suitable for storing objects.
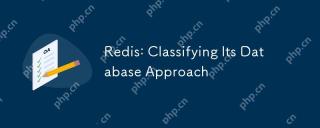
Redis's database methods include in-memory databases and key-value storage. 1) Redis stores data in memory, and reads and writes fast. 2) It uses key-value pairs to store data, supports complex data structures such as lists, collections, hash tables and ordered collections, suitable for caches and NoSQL databases.
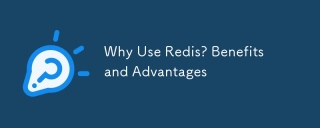
Redis is a powerful database solution because it provides fast performance, rich data structures, high availability and scalability, persistence capabilities, and a wide range of ecosystem support. 1) Extremely fast performance: Redis's data is stored in memory and has extremely fast read and write speeds, suitable for high concurrency and low latency applications. 2) Rich data structure: supports multiple data types, such as lists, collections, etc., which are suitable for a variety of scenarios. 3) High availability and scalability: supports master-slave replication and cluster mode to achieve high availability and horizontal scalability. 4) Persistence and data security: Data persistence is achieved through RDB and AOF to ensure data integrity and reliability. 5) Wide ecosystem and community support: with a huge ecosystem and active community,
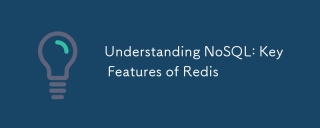
Key features of Redis include speed, flexibility and rich data structure support. 1) Speed: Redis is an in-memory database, and read and write operations are almost instantaneous, suitable for cache and session management. 2) Flexibility: Supports multiple data structures, such as strings, lists, collections, etc., which are suitable for complex data processing. 3) Data structure support: provides strings, lists, collections, hash tables, etc., which are suitable for different business needs.
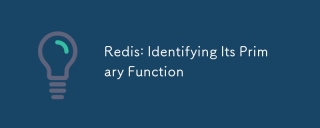
The core function of Redis is a high-performance in-memory data storage and processing system. 1) High-speed data access: Redis stores data in memory and provides microsecond-level read and write speed. 2) Rich data structure: supports strings, lists, collections, etc., and adapts to a variety of application scenarios. 3) Persistence: Persist data to disk through RDB and AOF. 4) Publish subscription: Can be used in message queues or real-time communication systems.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
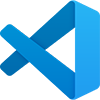
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
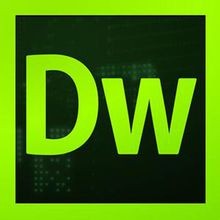
Dreamweaver CS6
Visual web development tools