How to implement counting sorting algorithm in C#
How to implement the counting sort algorithm in C
#Counting sort is a simple but effective sorting algorithm that can achieve O(n k) time complexity Sort a set of integers, where n is the number of elements to be sorted and k is the range of elements to be sorted.
The basic idea of counting sorting is to create an auxiliary array to count the number of occurrences of each element in the sequence to be sorted. Then, by performing a sum operation on the auxiliary array, the position of each element in the ordered sequence is obtained. Finally, based on the statistical results of the auxiliary array, the elements are put back into the original array to complete the sorting.
The following is a specific code example for implementing the counting sorting algorithm in C#:
using System; class CountingSort { public static void Sort(int[] array) { if (array == null || array.Length == 0) { return; } // 找到待排序序列中的最大值和最小值 int min = array[0]; int max = array[0]; for (int i = 1; i < array.Length; i++) { if (array[i] < min) { min = array[i]; } if (array[i] > max) { max = array[i]; } } // 创建辅助数组count,用于统计待排序序列中每个元素的出现次数 int[] count = new int[max - min + 1]; // 统计每个元素的出现次数 for (int i = 0; i < array.Length; i++) { count[array[i] - min]++; } // 对辅助数组进行求和操作,得到每个元素在有序序列中的位置 for (int i = 1; i < count.Length; i++) { count[i] += count[i - 1]; } // 创建临时数组,用于存储排序结果 int[] sortedArray = new int[array.Length]; // 根据辅助数组的统计结果,将元素放回原始数组中 for (int i = array.Length - 1; i >= 0; i--) { int index = count[array[i] - min] - 1; sortedArray[index] = array[i]; count[array[i] - min]--; } // 将排序结果拷贝回原始数组 Array.Copy(sortedArray, array, array.Length); } // 测试计数排序算法 static void Main(string[] args) { int[] array = { 5, 2, 9, 3, 1, 6, 8, 4, 7 }; Console.WriteLine("原始数组:"); PrintArray(array); Sort(array); Console.WriteLine("排序结果:"); PrintArray(array); } // 打印数组 static void PrintArray(int[] array) { foreach (int element in array) { Console.Write(element + " "); } Console.WriteLine(); } }
In the above code, we first find the maximum and minimum values in the sequence to be sorted, and then create the auxiliary array count to Count the occurrences of each element. Next, the position of each element in the ordered sequence is obtained by performing a sum operation on the auxiliary array. Finally, based on the statistical results of the auxiliary array, the elements are put back into the original array to complete the sorting.
In the test code, we use a sample array to test the counting sort algorithm. The output shows the original array and the sorted results.
Through the above code examples, we can understand how to implement the counting sorting algorithm in C#. Counting sort is a simple but effective sorting algorithm, especially suitable for situations where the range of elements in the sequence to be sorted is small. By mastering the principles and implementation of counting sorting algorithms, we can choose the most suitable sorting algorithm when sorting is needed and improve the efficiency of the program.
The above is the detailed content of How to implement counting sorting algorithm in C#. For more information, please follow other related articles on the PHP Chinese website!
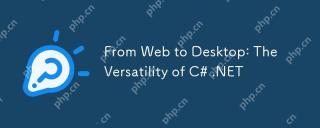
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
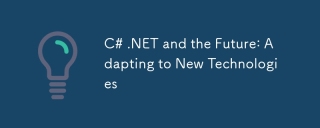
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
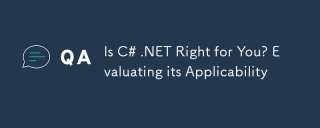
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
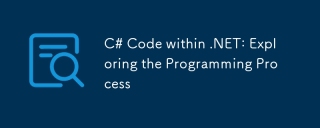
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.
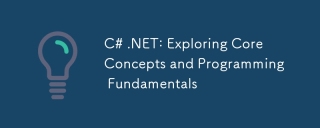
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.
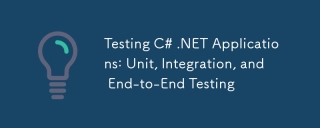
Testing strategies for C#.NET applications include unit testing, integration testing, and end-to-end testing. 1. Unit testing ensures that the minimum unit of the code works independently, using the MSTest, NUnit or xUnit framework. 2. Integrated tests verify the functions of multiple units combined, commonly used simulated data and external services. 3. End-to-end testing simulates the user's complete operation process, and Selenium is usually used for automated testing.
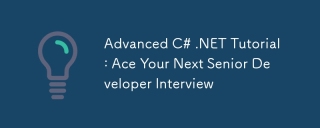
Interview with C# senior developer requires mastering core knowledge such as asynchronous programming, LINQ, and internal working principles of .NET frameworks. 1. Asynchronous programming simplifies operations through async and await to improve application responsiveness. 2.LINQ operates data in SQL style and pay attention to performance. 3. The CLR of the NET framework manages memory, and garbage collection needs to be used with caution.
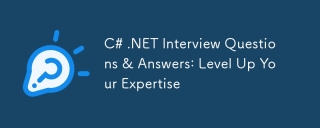
C#.NET interview questions and answers include basic knowledge, core concepts, and advanced usage. 1) Basic knowledge: C# is an object-oriented language developed by Microsoft and is mainly used in the .NET framework. 2) Core concepts: Delegation and events allow dynamic binding methods, and LINQ provides powerful query functions. 3) Advanced usage: Asynchronous programming improves responsiveness, and expression trees are used for dynamic code construction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
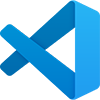
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software