


What core business functions can be achieved by Golang microservice development?
What core business functions can be achieved by Golang microservice development?
With the rapid development of cloud computing and container technology, microservice architecture has become one of the mainstream trends in modern application development. As an efficient, reliable and concurrent programming language, Golang has become an ideal choice for building microservices.
In Golang microservice development, we can achieve the following core business functions:
- RESTful API interface development
As the basis of microservices, the RESTful API interface allows different services to be communicate and interact. Golang provides a rich HTTP library to easily develop and expose RESTful API interfaces. Here is a simple example:
package main import ( "fmt" "log" "net/http" ) func main() { http.HandleFunc("/hello", helloHandler) log.Fatal(http.ListenAndServe(":8080", nil)) } func helloHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Hello, World!") }
- Database Integration
Microservices often need to interact with databases, such as storing and retrieving data. Golang's standard library provides support for SQL and NoSQL databases, such as MySQL, PostgreSQL, MongoDB, etc. The following is an example of using Golang to operate a MySQL database:
package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(localhost:3306)/database") if err != nil { log.Fatal(err) } defer db.Close() rows, err := db.Query("SELECT * FROM users") if err != nil { log.Fatal(err) } defer rows.Close() for rows.Next() { var id int var name string err = rows.Scan(&id, &name) if err != nil { log.Fatal(err) } fmt.Println(id, name) } }
- Message queue and asynchronous task processing
In the microservice architecture, the message queue is used to decouple the communication between different services. Communication, realizing asynchronous task processing, message passing and other functions. Golang provides multiple excellent message queue libraries, such as RabbitMQ, NATS, etc. The following is an example of sending a message to RabbitMQ using Golang:
package main import ( "log" "github.com/streadway/amqp" ) func main() { conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatal(err) } defer conn.Close() ch, err := conn.Channel() if err != nil { log.Fatal(err) } defer ch.Close() q, err := ch.QueueDeclare( "hello", false, false, false, false, nil, ) if err != nil { log.Fatal(err) } err = ch.Publish( "", q.Name, false, false, amqp.Publishing{ ContentType: "text/plain", Body: []byte("Hello, RabbitMQ!"), }, ) if err != nil { log.Fatal(err) } }
- Authentication and Authorization
Authentication and authorization in microservices are an important part of protecting the security of the service. Golang provides libraries for various authentication and authorization mechanisms, such as JWT, OAuth2, etc. The following is an example of using Golang to implement JWT authentication:
package main import ( "fmt" "log" "net/http" "github.com/dgrijalva/jwt-go" ) var jwtKey = []byte("secret_key") type Claims struct { Username string `json:"username"` jwt.StandardClaims } func main() { http.HandleFunc("/login", loginHandler) http.HandleFunc("/protected", authMiddleware(protectedHandler)) log.Fatal(http.ListenAndServe(":8080", nil)) } func loginHandler(w http.ResponseWriter, r *http.Request) { username := r.FormValue("username") password := r.FormValue("password") if username == "admin" && password == "admin" { token := jwt.NewWithClaims(jwt.SigningMethodHS256, Claims{ Username: username, }) tokenString, err := token.SignedString(jwtKey) if err != nil { log.Fatal(err) } fmt.Fprintln(w, tokenString) } else { w.WriteHeader(http.StatusUnauthorized) fmt.Fprintln(w, "Username or password incorrect") } } func protectedHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Protected resource") } func authMiddleware(next http.HandlerFunc) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { tokenString := r.Header.Get("Authorization") token, err := jwt.ParseWithClaims(tokenString, &Claims{}, func(token *jwt.Token) (interface{}, error) { return jwtKey, nil }) if claims, ok := token.Claims.(*Claims); ok && token.Valid { fmt.Println(claims.Username) next(w, r) } else { w.WriteHeader(http.StatusUnauthorized) fmt.Fprintln(w, "Unauthorized") } } }
By using Golang to develop microservices, we can achieve tasks such as RESTful API interface development, database integration, message queue and asynchronous task processing, authentication and Authorization and other core business functions. Golang's simplicity, efficiency, and concurrency features make building reliable microservices easier and more efficient.
The above is the detailed content of What core business functions can be achieved by Golang microservice development?. For more information, please follow other related articles on the PHP Chinese website!
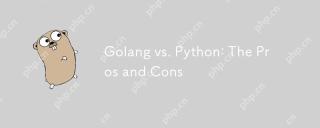
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
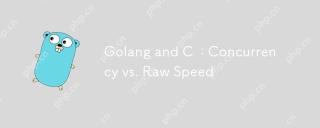
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
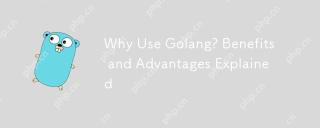
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
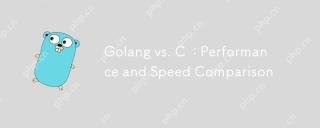
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
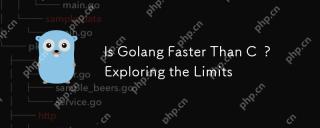
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
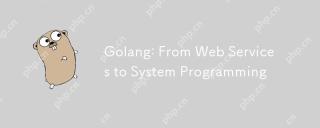
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
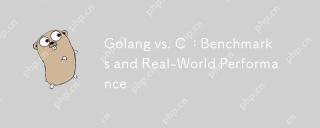
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
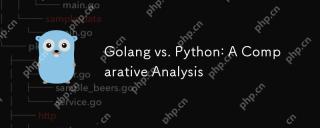
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.