


Java skills experience sharing and best practice summary for database search effect optimization
Java skills experience sharing and best practice summary for optimizing database search effects
In most modern applications, database search is a key operation. Whether you are searching for products on an e-commerce website or searching for users on a social media platform, efficient database search is one of the important factors in improving user experience. In this article, I will share some Java tips and experiences to help you optimize database search results and provide some best practices.
- Using indexes
In the database, indexing is the key to improving search efficiency. By creating indexes on key columns, the database can find matching records faster. In order to maximize the effectiveness of the index, you should select appropriate columns for indexing, and do not blindly create an index for each column, as this will increase storage and maintenance overhead. It's also important to regularly review and optimize indexes, as index performance may degrade as data grows and changes.
The following is an example that demonstrates how to use Java code to create an index for a column of a database table:
// 创建数据库连接 Connection connection = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password"); // 创建索引 Statement statement = connection.createStatement(); String createIndexQuery = "CREATE INDEX idx_name ON users (name)"; statement.executeUpdate(createIndexQuery); // 关闭连接 statement.close(); connection.close();
- Optimizing query statements
Optimizing query statements is to improve database searches Another important aspect of efficiency. First of all, you should try to avoid using wildcards in queries, especially at the beginning of fields, because this will cause the database to perform a full table scan. Secondly, use appropriate keywords, such as WHERE clause, JOIN clause, etc., to reduce the amount of data in the search process as much as possible.
The following is an example that shows how to use Java code to optimize query statements:
// 创建数据库连接 Connection connection = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password"); // 创建查询语句 String query = "SELECT * FROM users WHERE name LIKE 'John%'"; // 执行查询 Statement statement = connection.createStatement(); ResultSet resultSet = statement.executeQuery(query); // 处理结果 while (resultSet.next()) { String name = resultSet.getString("name"); System.out.println(name); } // 关闭连接 resultSet.close(); statement.close(); connection.close();
- Batch operation
When the amount of data to be searched is large, use Batch operations can significantly improve search efficiency. By sending multiple query statements to the database at once, you can reduce the number of communications with the database, thereby saving time and resources.
Here is an example that shows how to do batch operations using Java code:
// 创建数据库连接 Connection connection = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password"); // 创建批量操作 Statement statement = connection.createStatement(); // 添加批量操作语句 statement.addBatch("INSERT INTO users (name) VALUES ('John')"); statement.addBatch("INSERT INTO users (name) VALUES ('Jane')"); statement.addBatch("INSERT INTO users (name) VALUES ('Tom')"); // 执行批量操作 int[] result = statement.executeBatch(); // 关闭连接 statement.close(); connection.close();
- Using caching
In some cases, caching can be used to improve Database search results. By caching query results in memory, frequent database accesses are avoided, thereby reducing search time. However, it should be noted that updating the cache is also a problem, because when the data in the database changes, the cache needs to be updated accordingly.
The following is an example showing how to implement caching using Java code:
// 创建缓存 Map<String, List<User>> cache = new HashMap<>(); // 搜索缓存 String keyword = "John"; List<User> result = cache.get(keyword); // 如果缓存中不存在结果,则从数据库中搜索 if (result == null) { Connection connection = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password"); String query = "SELECT * FROM users WHERE name LIKE '%" + keyword + "%'"; Statement statement = connection.createStatement(); ResultSet resultSet = statement.executeQuery(query); result = new ArrayList<>(); while (resultSet.next()) { String name = resultSet.getString("name"); result.add(new User(name)); } // 缓存结果 cache.put(keyword, result); // 关闭连接 resultSet.close(); statement.close(); connection.close(); } // 处理结果 for (User user : result) { System.out.println(user.getName()); }
When using cache, you need to pay attention to cache capacity and update strategy to ensure cache effectiveness and consistency.
To sum up, these are some Java tips and experience sharing for optimizing database search results. By properly using indexes, optimizing query statements, using batch operations and caching, the performance and efficiency of database searches can be significantly improved. However, these techniques need to be selected and implemented according to specific application scenarios and needs, and appropriately adjusted and optimized. Hope this article helps you!
The above is the detailed content of Java skills experience sharing and best practice summary for database search effect optimization. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
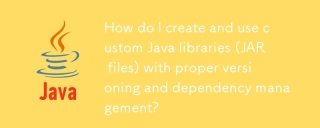
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
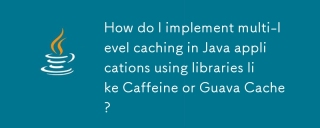
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
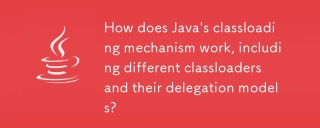
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
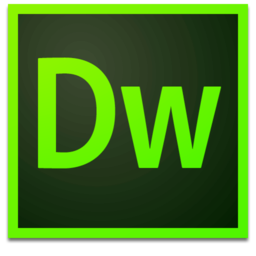
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.