


Actual implementation verification of database search optimization cases driven by Java technology
Actual implementation verification of database search optimization cases driven by Java technology
Abstract: As the amount of data in the database increases, the performance of search operations gradually becomes a bottleneck. This article will introduce a database search optimization solution driven by Java technology and verify its effectiveness through actual cases. Specifically, we will use the concept of database indexes and ConcurrentHashMap in the Java language to achieve fast and efficient search operations.
Introduction
Database search is a very common operation in daily applications. However, as the amount of data increases, traditional search operations gradually expose performance problems. To address this problem, this article will introduce a database search optimization solution based on Java technology and verify its effectiveness through actual cases. This solution mainly uses database indexes and ConcurrentHashMap in Java language to achieve fast and efficient search operations.
1. Database index
The database index is a special data structure used to improve the query speed of the database. The index can be simply understood as a "directory". According to the index, the location of the data can be quickly located, thereby improving query efficiency. In practical applications, we can create indexes based on frequently queried fields, such as user IDs, product names, etc. To create an index in the database, you can use the "CREATE INDEX" command in the SQL statement.
2. Concurrent HashMap
The Java language provides a concurrent HashMap (ConcurrentHashMap) class, which is a thread-safe HashMap implementation. Compared with traditional HashMap, using ConcurrentHashMap in a multi-threaded environment can avoid thread safety issues and have higher concurrency performance. In our database search optimization solution, ConcurrentHashMap will be used to store search results to improve the efficiency of search operations.
3. Case Background
In order to verify the effectiveness of our database search optimization plan, we formulated a database search scenario. Suppose we have a table that stores website user information, including user ID, user name, email, etc. Now we want to search by user ID and find out the user information for a specific ID.
4. Optimization plan
In the optimization plan, we need to use database index and ConcurrentHashMap to improve the efficiency of search operations. The specific steps are as follows:
- Create a database index: Create an index on the user ID field. You can use the SQL command "CREATE INDEX" to create an index in the database.
- Query operation: First, perform a memory search through ConcurrentHashMap based on the ID entered by the user. If the search result is empty, query through the database index.
5. Implementation code
The following is a Java code example to implement this search optimization solution:
import java.sql.*; import java.util.concurrent.*; public class DatabaseSearchOptimization { private static final ConcurrentHashMap<Integer, String> cache = new ConcurrentHashMap<>(); public static String searchUserByID(int userID) throws SQLException { // 先从内存中查询 String result = cache.get(userID); if (result == null) { // 缓存中不存在该用户,则通过数据库索引查询 Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/dbname", "username", "password"); PreparedStatement pstmt = conn.prepareStatement("SELECT * FROM user WHERE userID = ?"); pstmt.setInt(1, userID); ResultSet rs = pstmt.executeQuery(); if (rs.next()) { result = rs.getString("username"); // 将查询结果放入缓存中 cache.put(userID, result); } rs.close(); pstmt.close(); conn.close(); } return result; } public static void main(String[] args) { try { String username = searchUserByID(12345); System.out.println("Username: " + username); } catch (SQLException e) { e.printStackTrace(); } } }
In the above example code, we first search in ConcurrentHashMap, If no results are found, the query is performed through the database index. If the query result exists, it will be stored in ConcurrentHashMap for the next search.
6. Actual implementation verification
In order to verify the effectiveness of our optimization plan, we can verify it by actually running the code and conducting performance testing. You can evaluate the effectiveness of optimization by recording the time spent searching and comparing it to an unoptimized search operation.
Conclusion
Through the verification of actual cases, we can conclude that using database indexes and ConcurrentHashMap in the Java language can significantly improve the efficiency of database search operations. This solution is not only simple and easy to implement, but also suitable for most database search scenarios. In practical applications, the solution can be refined and expanded according to specific needs and scenarios to further improve search performance.
The above is the detailed content of Actual implementation verification of database search optimization cases driven by Java technology. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
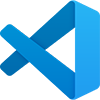
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
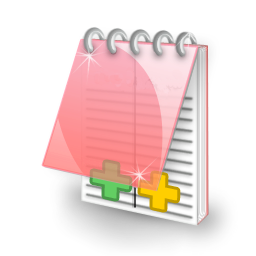
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use