


Given a string, find a subsequence of length at least two that is repeated in the string. The indices of the subsequence element numbers cannot be in the same order.
string s = "PNDPNSP"; print("Repeated subsequence of length 2 or more: ", (check(s) ? "Yes" : "No"));
Let’s look at a few examples below to understand how this approach works in different situations -
Example 1 - str = "PNDPNSP"
Explanation − Here, the answer is true because there is a recurring subsequence "PN" in the string.
Example 2 - str = "PPND"
Explanation - Here, the answer is wrong because there is no repeated subsequence of length at least two in the string.
Example 3 − str = "PPNP"
Explanation - Here, the answer is correct because "PP" indexes 0 and 1 and "PP" indexes 1 and 3 exist, and the "PP" used have different orderings in the subsequence index of. (0-based index)
The Chinese translation ofBrute force Approach
is:Brute force approach
This method will generate all possible subsequences of length 2 (minimum length) and find if we have already seen that subsequence with the found subsequence. If the subsequence already exists, we return true and terminate the program; otherwise, after completing the iteration, if we found nothing, we return false.
In the worst case, this subsequence may not exist and we end up generating all possible results.
subsequences of two lengths and store them. So assuming you hash the calculated subsequence to achieve O(1) insertion and search, this becomes O(n^2). The total subsequence is also O(n^2), so the storage space is as well.Modified longest common subsequence (LCS)
LCS algorithm finds the longest common subsequence in 2 strings. It is a standard dynamic programming method that uses an iterative method of two-dimensional matrices. The time complexity is O(n^2). We will only search the given string itself in the modified method. Nonetheless, we will also check if the index of the current position is not the same.
Example
See the C code below to implement the modified longest common subsequence algorithm that facilitates our method to find repeating subsequences of length 2 or more -
#include <iostream> using namespace std; bool modifiedLongestCommonSubsequence(string s) { int n = s.length(); int dp[n+1][n+1]; for (int i=0; i<=n; i++) fill(dp[i], dp[i]+n+1, 0); for (int i=1; i<=n; i++) { for (int j=1; j<=n; j++) { if (s[i-1]==s[j-1] && i!=j) { dp[i][j] = 1 + dp[i-1][j-1]; } else { dp[i][j] = max(dp[i][j-1], dp[i-1][j]); } } } if(dp[n][n] > 1) return true; return false; } int main() { string str = "PNDPNSP"; cout << "Repeated subsequence of length 2 or more: " << (modifiedLongestCommonSubsequence(str) ? "Yes" : "No"); return 0; }
Output
Repeated subsequence of length 2 or more: Yes
Of course, the time and space complexity is O(n^2), but from the first method, it is more elegant and easy to produce O(1) hash.
Improved solution
In this approach we will try to make some observations based on the previous approach.
Observation 1 − If a character appears more than twice, the answer is always true. Let's see why?
Example - In the string str = "AVHJFBABVNHFA" we have "AAA" in positions 0, 6 and 12. so We can have "AA" at index 0 and 6 as a subsequence, and "AA" at index 6 and 12 as a subsequence as another.
Observation 2 - If a character is repeated only once, it cannot contribute to our results subsequence, since it will only be available in at most one subsequence. it won't work Because we need at least two subsequences. So we can remove or ignore all characters happened at the same time.
Observation 3 − If a string is a palindrome, and the first two observations apply, then the answer is Always false unless the string length is odd. Let's see why?
Example - String str = "PNDDNP"
Explanation - Now, the characters are not in order, so we can never find The subsequences have the same order, so this is not possible.
Example
Based on all three observations above, we conclude that if we remove all characters that appear once in the string and then check if a certain character appears more than twice or if the string is not a palindrome, then we The answer is correct. Let’s see the improved solution implemented in C -
#include <iostream> using namespace std; bool isPalindrome(string s) { for(int i=0;i<s.size()/2;i++) { if(s[i]!=s[s.size()-1-i]) { return false; } } return true; } bool check(string s) { int hash[26] = {0}; for (int i = 0; i < s.size(); i++) { hash[s[i]-'a']++; if (hash[s[i]-'a'] > 2) { return true; } } int k = 0; string mstr = ""; for (int i = 0; i < s.size(); i++) { if (hash[s[i]-'a'] > 1) { mstr[k++] = s[i]; } } if (isPalindrome(mstr)) { return false; } return true; } int main() { string s = "PNDPNSP"; cout << "Repeated subsequence of length 2 or more: " << (check(s) ? "Yes" : "No"); return 0; }
Output
Repeated subsequence of length 2 or more: Yes
in conclusion
We concluded that using observations and hashes is the best way to solve this problem. The time complexity is O(n). Space complexity is also on the order of O(n), creating a new string and a constant 26 character hash.
The above is the detailed content of C++ program to find if a given string has a repeating subsequence of length 2 or more. For more information, please follow other related articles on the PHP Chinese website!
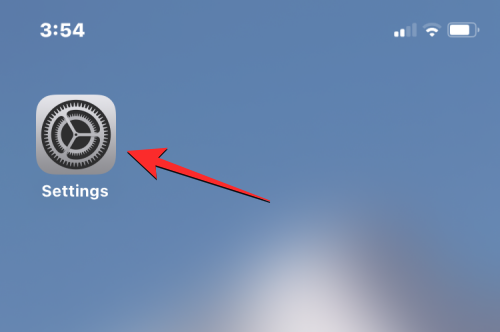
Apple的“查找”应用程序允许您定位您的iPhone或其他设备,以防止丢失或遗忘。虽然“查找”是一个有用的工具来追踪设备,但如果您关注隐私问题、不想耗尽电池或其他原因,您可能希望禁用它。幸运的是,有几种方法可以关闭iPhone上的“查找”,我们将在这篇文章中解释所有这些方法。如何在iPhone上关闭“查找”[4种方法]您可以通过四种方式关闭iPhone的“查找”。如果您使用方法1关闭“查找”,则可以从要禁用它的设备上执行此操作。要继续执行方法2、3和4,要关闭“查找”的iPhone应关闭电源或
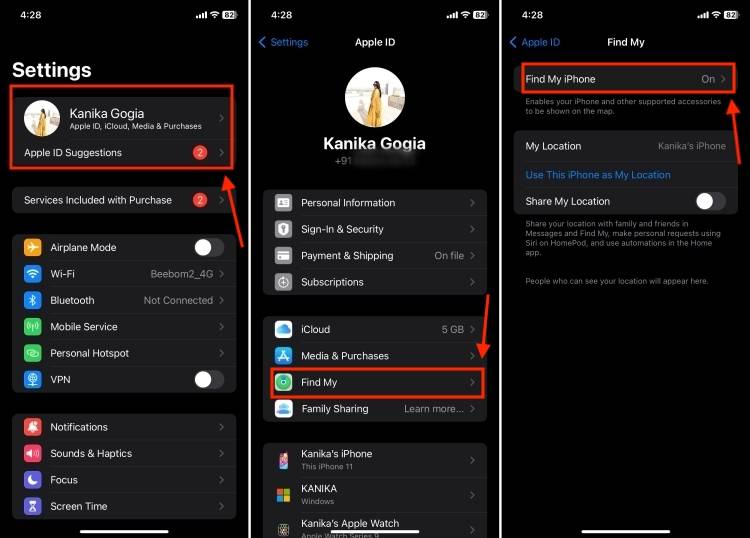
关闭iPhone版“查找”后会发生什么?“查找我的iPhone”可帮助您定位丢失或被盗的设备。启用后,“查找我的iPhone”可让您在地图上跟踪设备的位置、播放声音并帮助您找回设备。“查找”还包括一个激活锁,可防止任何人使用您的iPhone。当您关闭“查找我的iPhone”时,您将失去所有这些功能,这可能会使恢复丢失的Apple设备变得困难。虽然“查找我的iPhone”非常有用,但当您想出售、捐赠、以旧换新手机或想要将其送去更换电池或任何其他服务时,您应该禁用它。这样做将确保没有人可以访问有关您
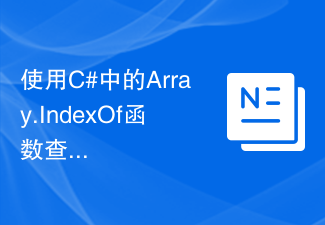
使用C#中的Array.IndexOf函数查找数组中某个元素的索引在C#程序中,当我们需要查找数组中某个元素的索引时,可以使用Array.IndexOf函数。Array.IndexOf函数会在指定的数组范围内查找指定的元素,并返回其第一次出现的索引。如果未找到该元素,则返回-1。下面是一段示例代码,演示了如何使用Array.IndexOf函数查找数组中某个元
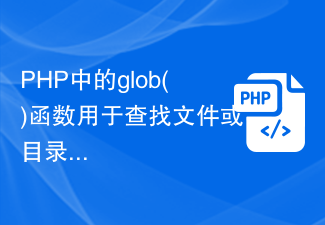
PHP中的glob()函数用于查找文件或目录,是一种强大的文件操作函数。它可以根据指定的模式匹配,返回文件或目录的路径。glob()函数的语法如下:glob(pattern,flags)其中,pattern表示要匹配的模式字符串,可以是一个通配符表达式,如*.txt(匹配以.txt结尾的文件),或者是具体的文件路径。flags是一个可选参数,用于控制函数
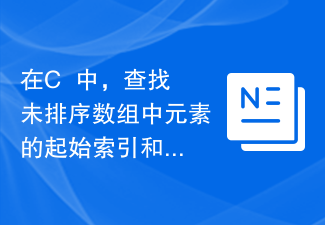
在这个问题中,我们得到一个包含n个未排序整数值的数组aar[]和一个整数val。我们的任务是在未排序的数组中查找元素的开始和结束索引。对于数组中元素的出现,我们将返回,“起始索引和结束索引”(如果在数组中找到两次或多次)。“单个索引”(如果找到)如果数组中不存在,则“元素不存在”。让我们举个例子来理解问题,示例1Input:arr[]={2,1,5,4,6,2,3},val=2Output:startingindex=0,endingindex=5解释元素2出现两次,第一次出现在索引=0处,第二
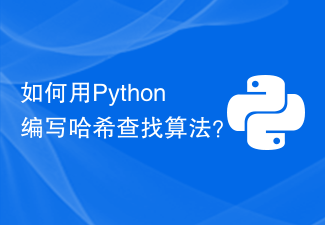
如何用Python编写哈希查找算法?哈希查找算法,又称为散列查找算法,是一种基于哈希表的数据查找方法。相比于线性查找和二分查找等传统查找算法,哈希查找算法具有更高的查找效率。在Python中,我们可以使用字典(dictionary)来实现哈希表,进而实现哈希查找。哈希查找算法的基本思想是将待查找的关键字通过哈希函数转换成一个索引值,然后根据索引值在哈希表中查
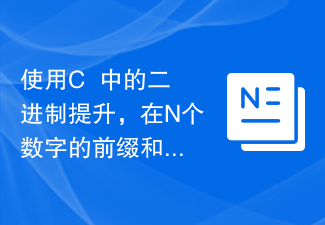
在这个问题中,我们得到一个由N个数字和一个整数值x组成的数组arr[]。我们的任务是创建一个程序,使用二进制提升在N个数字的前缀和中查找大于或等于X的第一个元素。前缀和是一个数组,其每个元素是初始数组中直到该索引为止的所有元素的总和。示例-array[]={5,2,9,4,1}prefixSumArray[]={5,7,16,20,21}让我们举个例子来理解这个问题,Input:arr[]={5,2,9,4,1},X=19Output:3解决方案在这里,我们将使用二元提升的概念来解决问题。二元提
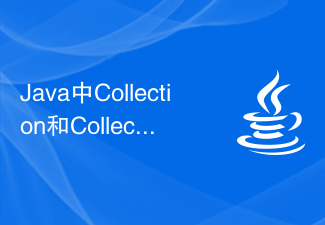
Collection是一个接口,而Collections是Java中的一个实用程序类。Set、List、和Queue是Collection接口的一些子接口,Map接口也是一部分Collections框架的一部分,但它不继承Collection接口。Collection接口的重要方法有add()、remove()、size()、clear()等,并且Collections类仅包含静态方法,如sort()、min()、max()、fill()、copy()、reverse()等。集合接口的语法pub


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
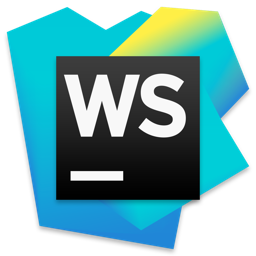
WebStorm Mac version
Useful JavaScript development tools
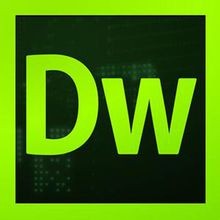
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
