What are some Python secret tricks?
Python is the most suitable and used language in the entire programming world. It is a huge language with many uses.
Although many developers know the basics of Python, there are many unknown techniques and strategies that can greatly improve your programming efficiency. In this article, we will cover some of the best kept secret tips for the Python programming language.
Use enumeration (Enumerate) to loop through the list with index
In Python, one of the most common tasks is to loop through a list of items. While most developers are familiar with the simple syntax of a for loop, there is an easy way to iterate through a list simultaneously and maintain a tempo of indexing. The enumerate function in Python allows you to do exactly this -
Example
vegetables = ['tomato', 'potato', 'ladyfinger'] for v, vegetable in enumerate(vegetables): print(v, vegetable)
Output
0 tomato 1 potato 2 ladyfinger
By using enumerate, you can avoid having to create an index variable and increment it manually, which can make your code more readable and maintainable.
Write concise code using list comprehensions
Python is considered to have the potential to write concise code. One good way to do this is to use list comprehensions. List comprehensions allow you to generate a new list by applying an expression to each object in the current list.
Example
nums = [2, 4, 6, 8, 10] squares = [a ** 2 for a in nums] print(squares)
Output
[4, 16, 36, 64, 100]
You can reduce the number of lines of code by using list comprehensions instead of creating a loop and appending it to a new list.
Combining lists using Zip
Zip is another helpful Python function. The zip function takes two or more lists and merges them into a single record consisting of tuples.
Example
vegetables = ['tomato', 'potato', 'ladyfinger'] rates = [80, 60, 70] inventory = zip(vegetables, rates) print(list(inventory))
Output
[('tomato', 80), ('potato', 60), ('ladyfinger', 70)]
By using zip, you can save time and improve the readability of your code by avoiding the use of zip Need to manually loop through many lists and splice them together.
Use the Join function to connect strings
Concatenating strings is a common operation in Python, however, writing out all the string literals and plus signs can be cumbersome. The be part of functions in Python allows you to concatenate strings more easily.
Example
words = ['I', 'love', 'Python'] sentence = ' '.join(words) print(sentence)
Output
I love Python
By using join you avoid writing all the string literals and plus signs, which might Make your code more readable and maintainable.
Use collections to store unique values
In Python, units are data kind that lets you save special values. Sets are similar to lists or tuples, however, they don't permit duplicate values.
Example
values = [12, 14, 16, 18, 20, 20, 18, 16, 14, 12] diff_nums = set(values) print(diff_nums)
Output
{12, 14, 16, 18, 20}
By leveraging sets, you can easily eliminate duplicate values from a tuple or list.
Use the name attribute to check whether the module is running directly
In Python, you can see whether a module is being run directly or imported as a module by checking the value of the discover attribute. If a module is run directly, its identity attribute will be set to 'main'. Please see the example below −
Example
# example.py def main(): print('The main function executed') if __name__ == '__main__': main()
Output
The main function executed
If you run example.py, the main function will be executed. However, if you import example.py as a module, key features will not be executed. This is beneficial for developing modules that can be used in different programs, but can also be run directly for testing or demonstration purposes.
Fun with Python
If you type "import hello" in your program and execute it, you will get exciting output. Give it a try!
>>> import __hello__ Hello world!
Next, type "from __future__ import braces" into your program and execute it to see what Python will say to you.
>>> from __future__ import braces SyntaxError: not a chance
in conclusion
Anyway, these are just a few of the many helpful tips and tricks for Python programming. By using these tips, you can write code that is more concise, readable, and efficient. As you continue to learn the language further, you are sure to discover more hidden gems that can improve your programming abilities.
The above is the detailed content of What are some Python secret tricks?. For more information, please follow other related articles on the PHP Chinese website!
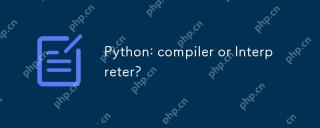
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
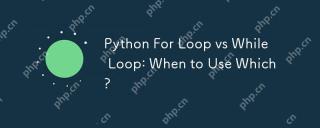
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
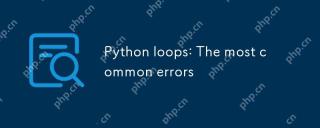
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
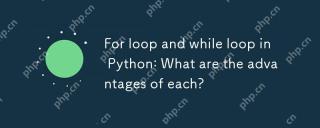
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
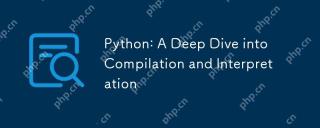
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
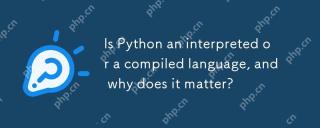
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
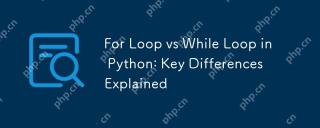
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
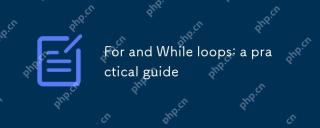
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
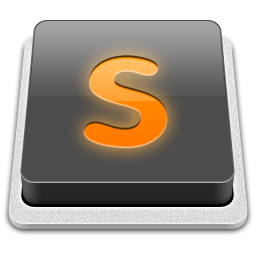
SublimeText3 Mac version
God-level code editing software (SublimeText3)
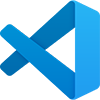
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
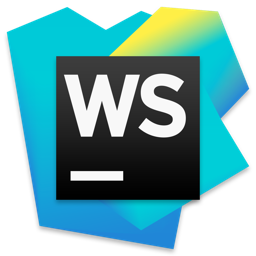
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
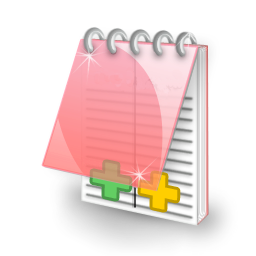
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
