Use a for loop when iterating over a sequence or for a specific number of times; use a while loop when continuing until a condition is met. For loops are ideal for known sequences, while while loops suit situations with undetermined iterations.
In the world of Python, understanding when to use a for
loop versus a while
loop can significantly impact the efficiency and readability of your code. So, when should you use which?
If you're iterating over a sequence or need to perform an action a specific number of times, a for
loop is your go-to choice. It's straightforward, concise, and perfect for dealing with known quantities. On the other hand, if you need to keep running a block of code until a certain condition is met, a while
loop is the way to go. It's ideal for situations where the number of iterations isn't predetermined.
Let's dive deeper into the nuances of these loops, sharing some personal experiences and insights along the way.
When I first started coding, I found for
loops incredibly intuitive. They're great for iterating over lists, strings, or any iterable object. Here's a simple example where I used a for
loop to process a list of names:
names = ["Alice", "Bob", "Charlie"] for name in names: print(f"Hello, {name}!")
This code is clean and easy to understand. I've used it countless times when working with datasets or when I need to apply a function to each item in a collection.
However, there are situations where for
loops can become cumbersome. Once, I was working on a game where the player had to guess a number. The number of guesses wasn't fixed, so a while
loop was more appropriate:
import random target_number = random.randint(1, 100) guess = None attempts = 0 while guess != target_number: guess = int(input("Guess a number between 1 and 100: ")) attempts = 1 if guess < target_number: print("Too low!") elif guess > target_number: print("Too high!") else: print(f"Congratulations! You guessed it in {attempts} attempts.")
In this case, a while
loop allowed the game to continue until the player guessed correctly, regardless of how many attempts it took.
One of the pitfalls I've encountered with while
loops is the risk of creating an infinite loop if the condition never becomes false. It's crucial to ensure that the condition can indeed change within the loop. Here's an example of how I once fixed an infinite loop by adding a counter:
counter = 0 while counter < 5: print(f"Counter is at {counter}") counter = 1 # This line was missing in the original code, causing an infinite loop
Performance-wise, for
loops are generally more efficient when dealing with large datasets, as they're optimized for iteration. I've noticed this particularly when processing large CSV files. Here's a snippet where I used a for
loop to read and process a CSV file efficiently:
import csv with open('data.csv', 'r') as file: reader = csv.reader(file) for row in reader: # Process each row print(row)
In contrast, using a while
loop for this task would be less efficient and more prone to errors, as you'd need to manually manage the iteration.
When it comes to best practices, I always emphasize readability and maintainability. For for
loops, I often use list comprehensions when the operation is simple and the result needs to be stored in a list. Here's an example:
numbers = [1, 2, 3, 4, 5] squared_numbers = [num ** 2 for num in numbers] print(squared_numbers) # Output: [1, 4, 9, 16, 25]
For while
loops, I ensure that the loop condition is clearly stated and that there's a clear exit strategy. I also try to keep the loop body as concise as possible to avoid complexity.
In conclusion, choosing between for
and while
loops depends on the specific requirements of your task. For
loops are ideal for iterating over known sequences, while while
loops are perfect for situations where you need to continue until a condition is met. By understanding the strengths and potential pitfalls of each, you can write more efficient and readable code. Remember, the key is to always consider the context and choose the loop that best fits your needs.
The above is the detailed content of Python For Loop vs While Loop: When to Use Which?. For more information, please follow other related articles on the PHP Chinese website!
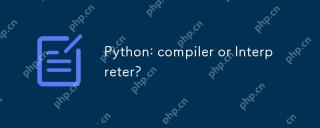
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
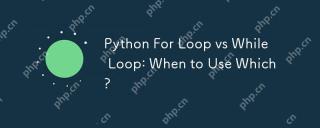
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
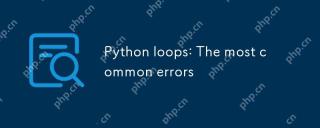
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
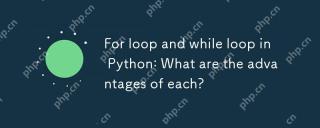
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
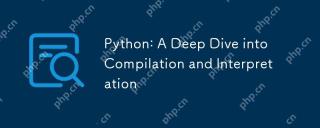
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
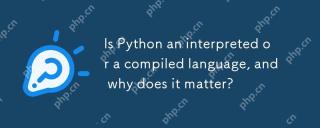
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
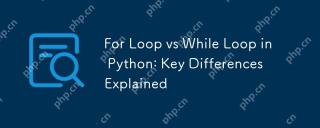
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
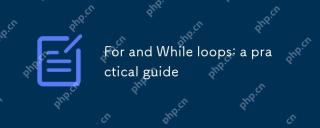
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
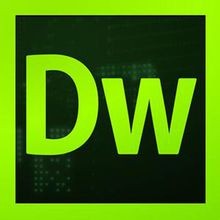
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
