


Translate the following into Chinese: Python program to convert local time to GMT time
When we create a web service that allows users around the world to book events, we might use this program to convert each user's local time to GMT before putting it into the database. This will make it easier for users in different time zones to compare and display event times. It is easier for users in different time zones to compare and display event times. In Python, we have some inbuilt time functions like timezone(), localize(), now() and astimezone() which can be used to convert local time to GMT. Local time represents the current time, while GMT is defined by calculating the prime meridian. GMT stands for Greenwich Mean Time, but is now called UTC (Coordinated Universal Time). This time is also known as Z time or Zulu time.
grammar
The following syntax is used in the example -
now()
This is the predefined method used in Python, which returns the local time without any time zone.
timezone()
The timezone() method is used to represent the time zone of a specific country or region. This method uses a module called pytz.
localize()
When you need to create a date and time, use the localize() method. A module called pytz helps run this built-in function.
astimezone()
This method is used as an object and passed the new time zone as parameter.
strftime()
This is a built-in method in Python that can be used to convert a date to a string. Therefore, users can easily understand what it is exactly.
utc.to().to()
This is a built-in method of the arrow module that can be used for two different time conversions.
tz_convert()
This method is used to convert one time zone to another.
tz_locallize()
This is also a built-in method that will be used to locate the time zone.
Example 1
In the example below we will start the program by importing everything datetime from a module named datetime which will look up the local time. We will then import everything for timezone and utc from a module called pytz which will look for GMT time. Then store the predefined method timezone() in the variable ltz. timezone() Method accepts parameters by taking the time zone of any country. Next, store utc in the variable time2, which will be used to calculate the GMT time zone. Continue to use the predefined methods localize() and astimezone() to find the local time. These two methods act as an object with variable ltz and store it in variable temp2. Finally, we print the local time result as GMT with the help of variables time1 and temp2.
from datetime import datetime from pytz import timezone,utc #local time time1 = datetime.now() #local timezone ltz = timezone( 'Asia/Kolkata' ) # GMT time2 = utc #Covert the local time into GMT time2 = ltz.localize( time1 ).astimezone( time2 ) print( "The current local time:", time1 ) print( "After conversion into GMT" ) print( "The current GMT:", time2 )
Output
The current local time: 2023-04-18 13:02:05.289406 After conversion into GMT The current GMT: 2023-04-18 07:32:05.289406+00:00
Example 2
In the following example, we will start the program by importing modules named datetime and pytz. Then initialize the variable l_time representing the local time, and use the predefined function datetime.now() to store the value of the current date and time. Then the timezone() method accepts parameters to pass the GMT time zone. This method acts as an object of the module named pytz, stored in the variable g_timezone. Next, the predefined function astimezone() accepts arguments as variables g_timezone. This method acts as an object with variable name l_time, converts local time to GMT and stores it in variable g_time. Finally, we print the result with the help of variable g_time.
import datetime import pytz #initialize the local time l_time = datetime.datetime.now() #Conversion of loctime - GMT g_timezone = pytz.timezone( 'GMT' ) g_time = l_time.astimezone( g_timezone ) # Print the GMT print( "The local time converts into GMT:\n", g_time )
Output
The local time converts into GMT: 2023-04-18 08:13:08.274818+00:00
Example 3
In the example below, we will start the program by importing everything datetime from a module called datetime, which will help convert local time to GMT time. Then import the time module that will be used to set the time for both time references. Then use the built-in method strftime() to initialize the two variables l_time and g_time that store the values, and find the local time and GMT time of the respective variables.
from datetime import datetime import time l_time = time.strftime('%Y-%m-%d %H:%M:%S', time.localtime()) print(f'Local time: {l_time}') g_time = time.strftime('%Y-%m-%d %H:%M:%S', time.gmtime()) print(f'GMT time: {g_time}')
Output
Local time: 2023-05-07 12:50:47 GMT time: 2023-05-07 12:50:47
Example 4
In the example below, we will first install the command named pip install arrow, which allows users to run programs based on the arrow module. Then start the program by importing the module named arrow. Next, use the built-in method utcnow() as an object of the arrow module and store it in the variable utc. Another name for GMT is UTC. Proceed to print the local time as GMT using the built-in method utc.to().to() which accepts two parameters - local and utc to display the time conversion.
import arrow utc = arrow.utcnow() print("\nlocal to utc:") print(utc.to('local').to('utc'))
输出
local to utc: 2023-05-10T11:15:37.548334+00:00
示例 5
在下面的示例中,通过导入名为 pandas 的模块并以对象 pd 作为引用来开始程序。然后从 datetime 类导入模块 datetime。接下来,使用内置方法 datetime() 存储日期和时间,该方法接受六个参数,即年、月、日、小时、分钟和秒,并将其存储在变量 local_time 中。然后使用内置方法 Timestamp 接受名为 local_time 的参数,该参数转换计算机记录的当前时间和事件。开始初始化名为 local_tz 的变量,该变量通过替换本地时区来存储值。要将本地化时间戳转换为 GMT,它将值初始化为 GMT 并将它们存储在变量 gmt_tz 中(tz 是时区的缩写)。然后使用内置方法 tz_convert() ,它充当带有时间戳的对象,接受名为 gmt_tz 的参数,这有助于将时间转换为不同的模式并存储它在变量gmt_timestamp中。最后,使用 print 函数并传递名为 gmt_timestamp 的参数来获取结果。
import pandas as pd from datetime import datetime local_time = datetime(2023, 5, 10, 17, 22, 0) timestamp = pd.Timestamp(local_time) local_tz = 'asia/kolkata' # replace with your local timezone local_timestamp = timestamp.tz_localize(local_tz) gmt_tz = 'GMT' gmt_timestamp = local_timestamp.tz_convert(gmt_tz) print(gmt_timestamp)
输出
2023-05-10 11:52:00+00:00
结论
以上两个输出显示了 GMT 与当前日期的结果。我们看到了内置函数如何帮助将本地时间的时区转换为 GMT。 timezone() 方法接受定义本初子午线的 GMT 参数。 astimezone() 接受参数作为时区并生成结果。
The above is the detailed content of Translate the following into Chinese: Python program to convert local time to GMT time. For more information, please follow other related articles on the PHP Chinese website!
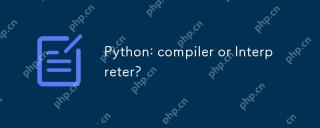
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
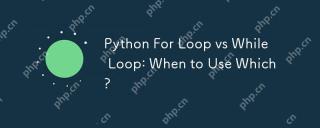
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
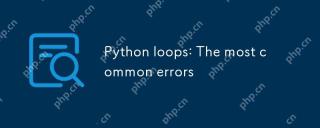
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
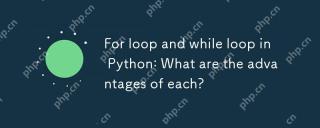
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
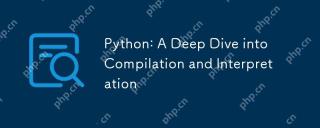
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
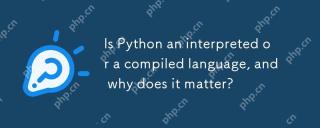
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
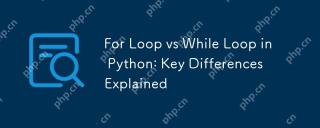
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
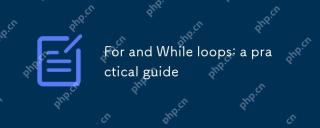
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
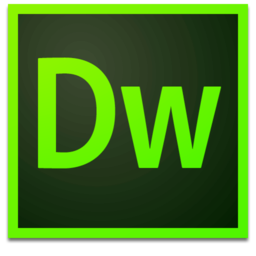
Dreamweaver Mac version
Visual web development tools
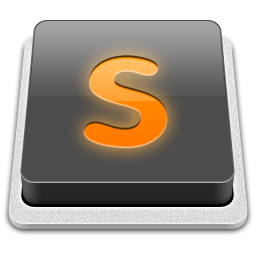
SublimeText3 Mac version
God-level code editing software (SublimeText3)
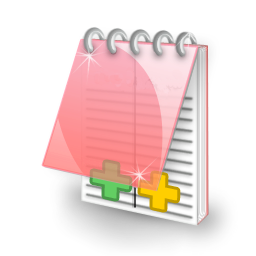
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
