How to implement efficient queue processing in PHP?
Queue is a common data structure, often used to implement asynchronous task processing, message queues and other scenarios. In PHP, achieving efficient queue processing can improve performance through appropriate data structures and algorithms. This article will introduce several commonly used queue processing methods and provide corresponding code examples.
1. Array Queue
The simplest way to implement a queue is to use a PHP array, and use the push and shift methods of the array to implement the queue entry and exit operations. The following is a sample code implemented using an array queue:
class ArrayQueue { private $queue = array(); public function enqueue($item) { array_push($this->queue, $item); } public function dequeue() { if ($this->isEmpty()) { return null; } return array_shift($this->queue); } public function isEmpty() { return empty($this->queue); } } // 使用示例 $queue = new ArrayQueue(); $queue->enqueue("Task 1"); $queue->enqueue("Task 2"); $task = $queue->dequeue(); echo $task; // 输出 Task 1
The advantage of the array queue is that it is simple and easy to use, but its performance is poor when processing large amounts of data, because each dequeue operation requires re-indexing the array elements.
2. Queue library
There are some mature queue libraries in PHP, such as Beanstalkd, RabbitMQ, etc., which have high performance, reliability and good scalability. Using these queue libraries, tasks can be distributed to multiple Worker processes for processing, improving overall performance.
The following is a sample code for using Beanstalkd as a queue library:
// 安装 beanstalkd 扩展库 // 执行命令:pecl install beanstalk // 生产者代码 $beanstalk = new Beanstalkd(); $beanstalk->connect(); $beanstalk->useTube('task_queue'); $beanstalk->put(json_encode(["data" => "Task data"])); // 消费者代码 $beanstalk = new Beanstalkd(); $beanstalk->connect(); $beanstalk->watch('task_queue'); while (true) { $job = $beanstalk->reserve(); $data = json_decode($job->getData(), true); // 处理任务逻辑 $beanstalk->delete($job); }
Using the queue library can effectively decouple producers and consumers, improving processing efficiency when the task volume is large.
3. Multi-process queue processing
In PHP, you can improve the performance of queue processing through multi-process. By using the pcntl library and multi-process, tasks can be distributed to multiple sub-processes for processing, thereby achieving parallel processing and improving efficiency.
The following is a sample code for using multiple processes:
// 创建子进程处理任务 function worker($queue) { while (true) { $task = $queue->dequeue(); if ($task == null) { break; } // 处理任务逻辑 } } // 主进程代码 $queue = new ArrayQueue(); // 创建5个子进程 $processes = 5; $pid = pcntl_fork(); if ($pid == -1) { die("Error forking"); } elseif ($pid == 0) { worker($queue); // 子进程处理任务 exit(0); } // 生产者向队列中添加任务 // 例如: for ($i = 0; $i < 100; $i++) { $queue->enqueue("Task $i"); } // 等待子进程结束 while ($processes > 0) { pcntl_wait($status); $processes--; }
Using multiple processes can handle multiple tasks at the same time, improving the overall efficiency of queue processing.
In summary, to achieve efficient queue processing, you can choose appropriate data structures and algorithms, use mature queue libraries, or combine multiple processes to handle tasks. The specific selection method depends on actual needs and specific scenarios. The sample code provided above can be used as a reference, and readers can make appropriate modifications and optimizations according to their own needs.
The above is the detailed content of How to implement efficient queue processing in PHP?. For more information, please follow other related articles on the PHP Chinese website!
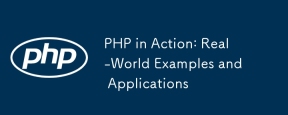
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
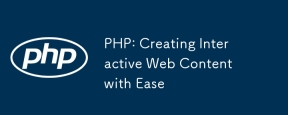
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
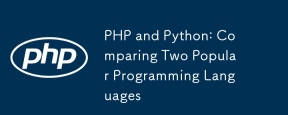
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
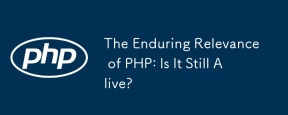
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
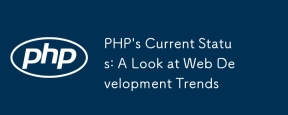
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
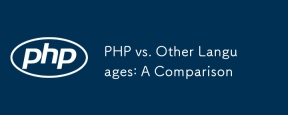
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
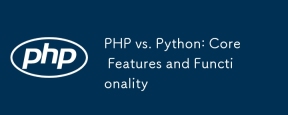
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
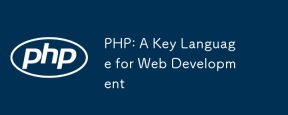
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
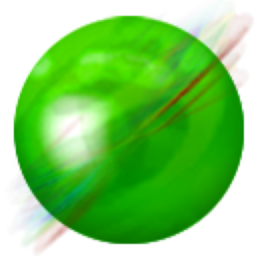
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
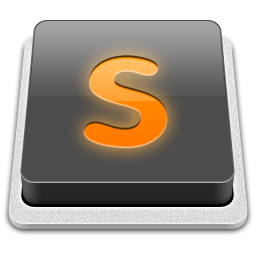
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.