When you visit a web page, it usually generates text files containing small data such as usernames and passwords, and stores them on the user's browser. These are known cookies that are used to identify a user's system and can be accessed by the web server or the client computer (the computer where they are stored).
The information stored in cookies is specific to the web server.
Once you connect to the server, a cookie tagged with a unique ID is created and stored on your computer.
Once the cookie is exchanged/stored in the client, and if you connect to the server again, it will recognize your system based on the stored cookie.
This helps the server serve personalized pages to specific users.
Example 1
The following example creates a cookie and verifies that it is set.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Cookie; class UserController extends Controller { public function index(Request $request) { Cookie::queue('msg', 'cookie testing', 10); echo $value = $request->cookie('msg'); } }
Output
The output of the above code is -
Example 2
Another way to test whether the cookie is set can be seen in the example below -
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Cookie; class UserController extends Controller { public function index(Request $request) { Cookie::queue('msg', 'cookie testing', 10); return view('test'); } }
Test.blade.php
<!DOCTYPE html> <html> <head> <style> body { font-family: 'Nunito', sans-serif; } </style> <head> <body class="antialiased"> <div> {{ Cookie::get('msg') }} </div> </body> </html>
Output
The output of the above code is -
Example 3
Use the hasCookie() method to test whether a given cookie has been set.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Cookie; class UserController extends Controller{ public function index(Request $request) { if($request->hasCookie('msg')) { echo "Cookie present"; } else { echo "Cookie msg is not set"; } } }
Output
Cookie present
Example 4
Another example of testing cookie settings.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Cookie; class UserController extends Controller{ public function index(Request $request) { return view('test'); } }
Test.blade.php
<!DOCTYPE html> <html> <head> <style> body { font-family: 'Nunito', sans-serif; } </style> </head> <body class="antialiased"> <div> @if (Cookie::get('msg') !== false) <p>cookie is present.</p> @else <p>cookie is not set.</p> @endif </div> </body> </html>
Output
The output of the above code is -
cookie is present.
The above is the detailed content of How to check if cookie is set in Laravel?. For more information, please follow other related articles on the PHP Chinese website!
![修复:谷歌浏览器请求太多错误 429 [已解决]](https://img.php.cn/upload/article/000/887/227/168160812385289.png)
近期很多Windows用户反映,当他们尝试访问某个URL时,PC上的GoogleChrome浏览器显示错误429。这是因为每次用户尝试在短时间内通过浏览器。通常,此错误是由网站生成的,以避免通过向服务器发送过多请求而被机器人或黑客入侵病毒。用户对在这个阶段可以做什么感到困惑,并因此感到失望。导致此错误的因素可能很多,我们在下面列出了其中一些因素。缓存内存和其他站点数据未清除从第三方来源安装的扩展系统上的一些有害软件病毒攻击在研究了上面列出的因素之后,我们在这篇文章中收集了一些修复程序,这
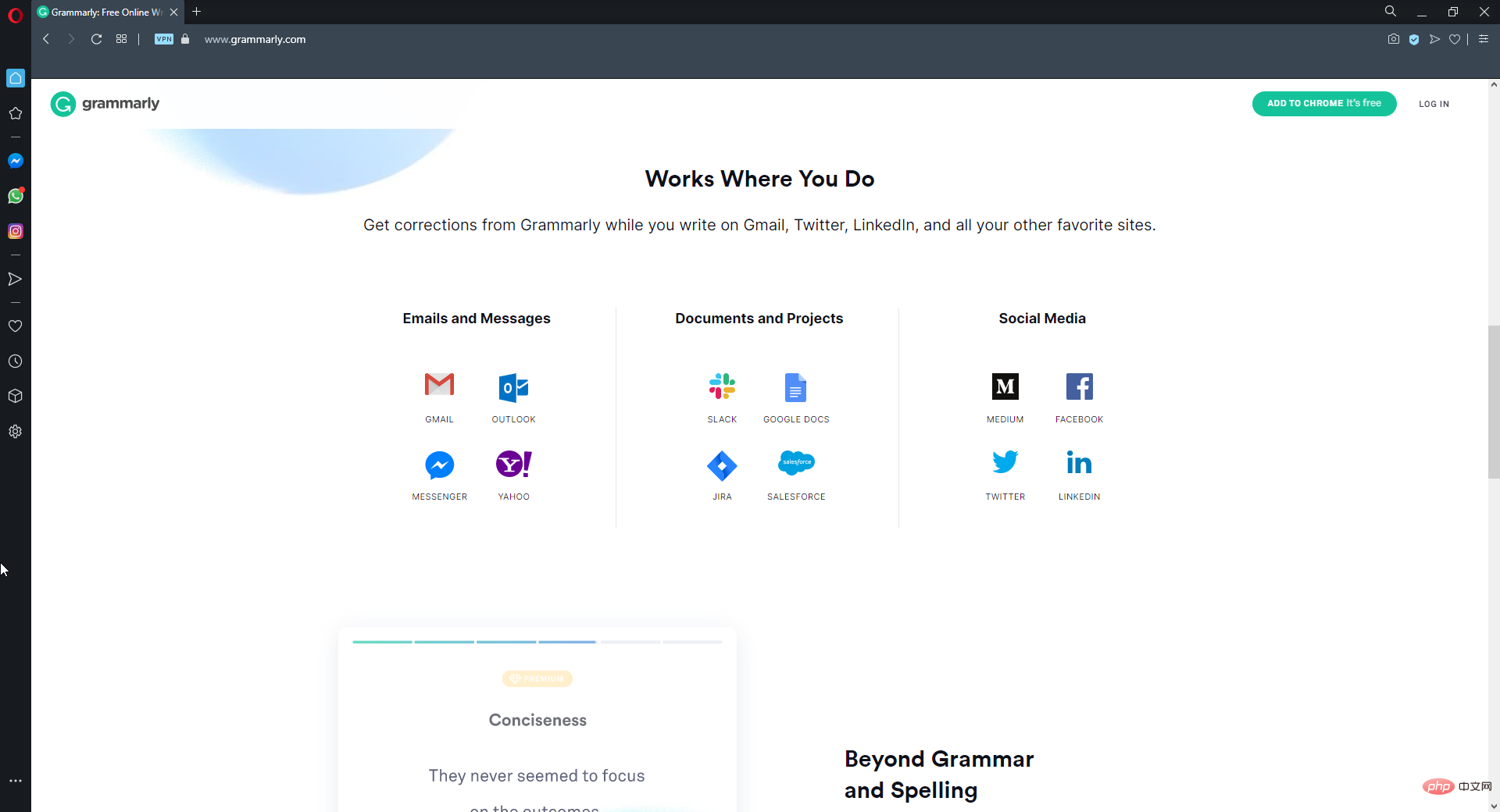
如果您在Windows10或11PC上遇到语法问题,本文将帮助您解决此问题。Grammarly是最流行的打字助手之一,用于修复语法、拼写、清晰度等。它已经成为写作专业人士必不可少的一部分。但是,如果它不能正常工作,它可能是一个非常令人沮丧的体验。许多Windows用户报告说此工具在他们的计算机上运行不佳。我们做了深入的分析,找到了这个问题的原因和解决方案。为什么Grammarly无法在我的PC上运行?由于几个常见原因,PC上的Grammarly可能无法正常工作。它包括以下内
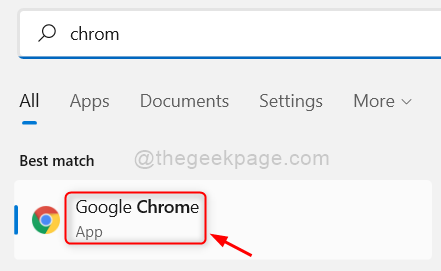
许多Windows用户最近在尝试访问GoogleChrome浏览器中的网站URL时遇到了一个不寻常的错误,称为Roblox403禁止错误。即使在多次重新启动Chrome应用程序后,他们也无能为力。此错误可能有几个潜在原因,我们在下面概述并列出了其中一些。Chrome的浏览历史和其他缓存以及损坏的数据不稳定的互联网连接网站网址不正确从第三方来源安装的扩展在考虑了上述所有方面之后,我们提出了一些修复程序,可以帮助用户解决此问题。如果您遇到同样的问题,请查看本文中的解决方案。修复1
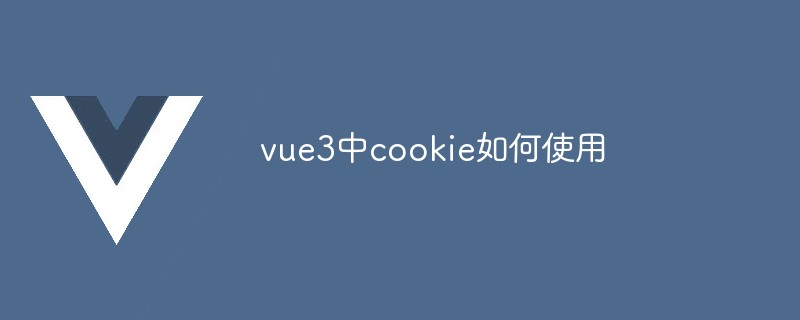
前言cookie使用最多的地方想必是保存用户的账号与密码,可以避免用户每次登录时都要重新输入1.vue中cookie的安装在终端中输入命令npminstallvue-cookies--save,即可安装cookies,安装之后在main.js文件中写下以下代码import{createApp}from'vue'importVueCookiesfrom'vue-cookies'constapp=createApp(App)app.co
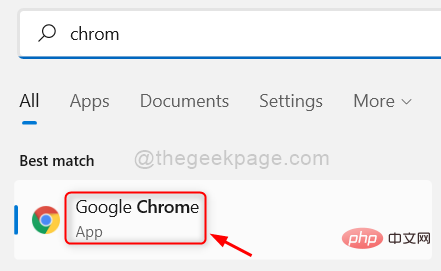
每个网站都通过创建cookie使用户更容易浏览他们的网页和浏览他们的网站。然而,网站创建了一些第三方cookie,使他们能够跟踪访问其他网站的用户,以便更好地了解他们,从而有助于展示广告和其他帖子。一些用户可能认为他们的数据遭到破坏或存在安全风险,而另一些用户可能认为允许这些第三方cookie跟踪他们以在浏览器上获取更多内容是很好的。所以我们在这篇文章中解释了如何在谷歌浏览器中启用或禁用第三方cookies,详细步骤如下。如何在GoogleChrome中启用第三方Cookie如果您认为要
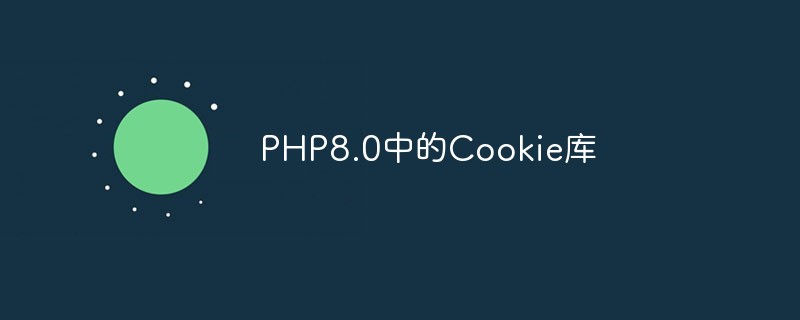
在互联网应用开发中,使用Cookie是常见的一种方式来维护用户会话状态。在PHP语言中,处理Cookie的相关功能在语言的核心库中得到了完善的支持,在最新的PHP8.0版本中,Cookie库得到了进一步的增强。一、PHP中的CookieCookie是一个小文本文件,可以存储在用户的浏览器中,它通常被用来记录用户的个性化设置、登录状态等信息。Cookie是基
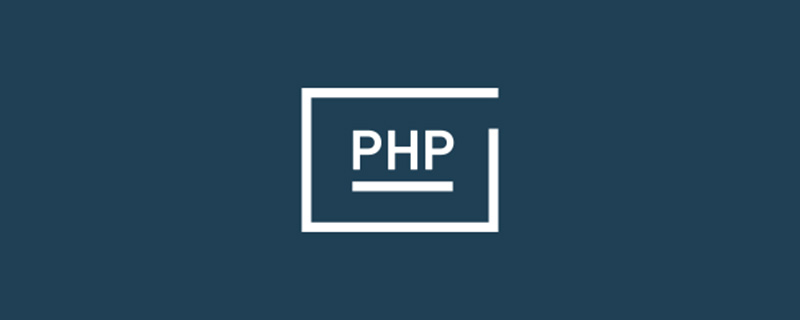
php curl设置cookie的方法:1、创建PHP示例文件;2、通过“curl_setopt”函数设置cURL传输选项;3、在CURL中传递cookie即可。
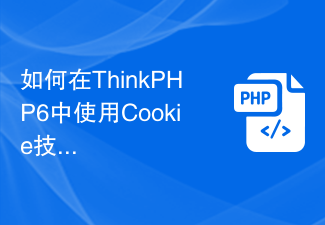
随着互联网技术的不断发展,越来越多的网站需要用户登录才能使用其功能。但是每次用户访问时都需要输入账号密码显然很不方便,因此“记住我”的功能应运而生。本文将介绍如何在ThinkPHP6中采用Cookie技术实现记住我功能。一、Cookie简介Cookie是一种服务器向客户端发送的小文件,在用户访问网站时存储在用户的计算机上。这些文件包含与用户相关的信息,如登录


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
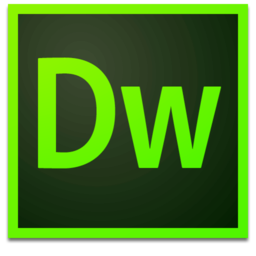
Dreamweaver Mac version
Visual web development tools
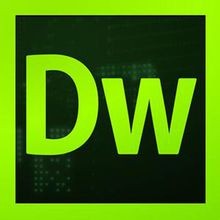
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
