In this article, we will discuss the code solution to swap every alternating bit in a given number and return the resulting number. We will solve this problem using the concept of bit operations to solve the problem in constant time without using any loops.
Problem statement − We are given a number n, we have to swap the pair of bits that are adjacent to each other.
In other words, we have to swap every odd placed bit with its adjacent even placed bit.
Constrain: While solving the problem, we have to keep In mind that we cannot use a loop for this problem, we have to execute our code in O(1) time complexity only.
Example
Input − n = 10011110
Output - After swapping the even bits and the odd bits,
the binary number obtained is: 01101101
Input − n = 10011110
Output - After swapping the even bits and the odd bits,
the binary number obtained is: 01101101
Explanation −
Let us consider the previous example for better understanding.
n = 10011110 Even position bits in n are E – 1 x 0 x 1 x 1 x Odd position bits in n are O – x 0 x 1 x 1 x 0
For the result, we want the even position bits at the odd position and vice-versa
For even position bits at odd position,
We need to right shift the even position by one position.
So, for bits in even positions, we just change E >> 1 to get the desired position.
Similarly, we have to left shift the odd position bits by one position to get the desired position of odd bits.
So, for the odd number of bits, we just need to change O
Now the next problem is to extract the odd and even position bits.
as we know,
0x55 = 01010101 in which every only odd position bits are set ( non 0 ). 0xAA = 10101010 in position bits are set. which, only odd
Hence to extract E from n, we just need to perform
E = n & 0xAA
Similarly, to extract O from n, we need to perform-
O = n & 0x55
Now, to find the swapped output,
step
The steps involved are-
E >> 1
O
Now, we combine E and O using or operation.
Hence our result will be – Result = ( E >> 1 | O
Example
is:Example
The code for this method is as follows:
#include<bits/stdc++.h> using namespace std; unsigned int swapbits(unsigned int n) { unsigned int E = n & 0xAA ; unsigned int O = n & 0x55 ; unsigned int result = (E >> 1)|(O << 1); return result; } int main() { unsigned int n = 14; cout << "After swapping the even position bits with off position bits, the binary number obtained is " << swapbits(n) << endl; return 0; // code is contributed by Vaishnavi tripathi }
Output
After swapping the even position bits with off position bits, the binary number obtained is 13
Time complexity - The time complexity of this method is O(1).
Space Complexity - We are not using any extra space. The auxiliary space complexity is O(1).
The above is the detailed content of swap every two bits in every two bytes. For more information, please follow other related articles on the PHP Chinese website!
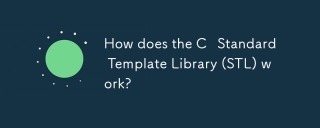
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
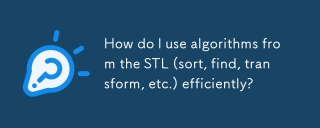
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
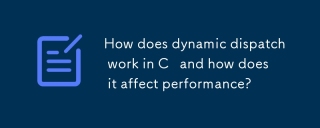
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
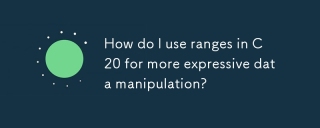
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
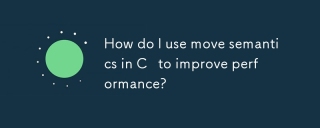
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
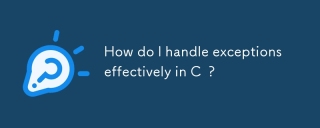
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
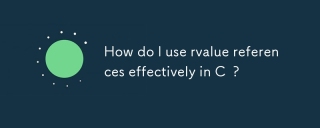
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
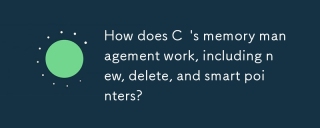
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
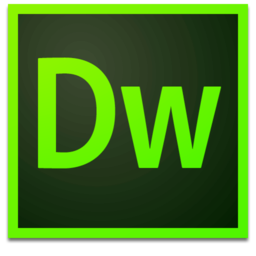
Dreamweaver Mac version
Visual web development tools
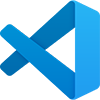
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
