How to use PHP to implement data analysis and report generation
Introduction:
In today's information age, data analysis and report generation are essential for corporate decision-making part. Fortunately, this functionality can be easily achieved using the PHP programming language. This article will introduce the basic methods and techniques of using PHP to implement data analysis and report generation, and provide some code examples.
1. Data analysis
- Data collection
First, we need to collect and prepare the data to be analyzed. Data can come from various sources, such as databases, log files, API interfaces, etc. PHP provides some powerful functions and classes to handle data reading and parsing.
Here is an example showing how to read data from a CSV file using PHP:
$file = fopen('data.csv', 'r'); $data = []; while (($row = fgetcsv($file)) !== false) { $data[] = $row; } fclose($file);
- Data Processing and Transformation
Once the data has been collected and Read, we can perform various processing and transformations on it for deeper analysis. This may include data cleaning, calculated metrics, filters, etc. PHP’s built-in functions and libraries help us accomplish these tasks easily.
Here is an example that shows how to calculate the average in an array:
$numbers = [10, 20, 30, 40, 50]; $average = array_sum($numbers) / count($numbers); echo 'The average is: ' . $average;
- Data Analysis and Visualization
After completing the data processing, we can use Various charting libraries and drawing tools for data analysis and visualization. PHP provides a wealth of chart libraries, such as Chart.js, PHPlot, etc., which can help us create various chart types.
The following is an example showing how to use Chart.js to create a histogram:
$data = [ 'labels' => ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'], 'datasets' => [ [ 'label' => 'My Dataset', 'data' => [12, 19, 3, 5, 2, 3], 'backgroundColor' => [ 'red', 'blue', 'yellow', 'green', 'purple', 'orange' ] ] ] ]; echo '<canvas id="myChart"></canvas>'; echo '<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>'; echo '<script>'; echo 'var ctx = document.getElementById("myChart").getContext("2d");'; echo 'var myChart = new Chart(ctx, {'; echo ' type: "bar",'; echo ' data: ' . json_encode($data) . ','; echo ' options: {}'; echo '});'; echo '</script>';
2. Report generation
- Report template design
Before generating the report, we need to design the report template, including report title, data table, chart, etc. PHP provides various HTML and CSS processing functions and classes to help us design flexible and beautiful report templates.
The following is an example of a report template:
<!DOCTYPE html> <html> <head> <title>Report</title> <style> table { width: 100%; } </style> </head> <body> <h1 id="Report-Title">Report Title</h1> <table> <tr> <th>Column 1</th> <th>Column 2</th> <th>Column 3</th> </tr> <?php foreach ($data as $row): ?> <tr> <td><?= $row[0] ?></td> <td><?= $row[1] ?></td> <td><?= $row[2] ?></td> </tr> <?php endforeach; ?> </table> </body> </html>
- Data filling and exporting
After designing the report template, we can use PHP to fill the data into the report , and then export to PDF or other formats. PHP provides some libraries and extensions, such as TCPDF, PHPExcel, etc., that can help us achieve this function.
Here is an example that shows how to export a report to PDF using TCPDF:
require_once 'tcpdf/tcpdf.php'; $pdf = new TCPDF(); $pdf->AddPage(); $pdf->SetFont('helvetica', 'B', 16); $pdf->Cell(0, 10, 'Report Title', 0, 1, 'C'); $pdf->Ln(); $pdf->SetFont('helvetica', 'B', 12); $pdf->Cell(33, 10, 'Column 1', 1); $pdf->Cell(33, 10, 'Column 2', 1); $pdf->Cell(33, 10, 'Column 3', 1); $pdf->SetFont('helvetica', '', 12); foreach ($data as $row) { $pdf->Ln(); $pdf->Cell(33, 10, $row[0], 1); $pdf->Cell(33, 10, $row[1], 1); $pdf->Cell(33, 10, $row[2], 1); } $pdf->Output('report.pdf', 'I');
Conclusion:
By using PHP programming language, we can easily implement data analysis and report generation functions. This article introduces basic methods and techniques, as well as gives some code examples. It is hoped that readers can further explore and expand based on these examples to meet their own needs.
The above is the detailed content of How to use PHP for data analysis and report generation. For more information, please follow other related articles on the PHP Chinese website!
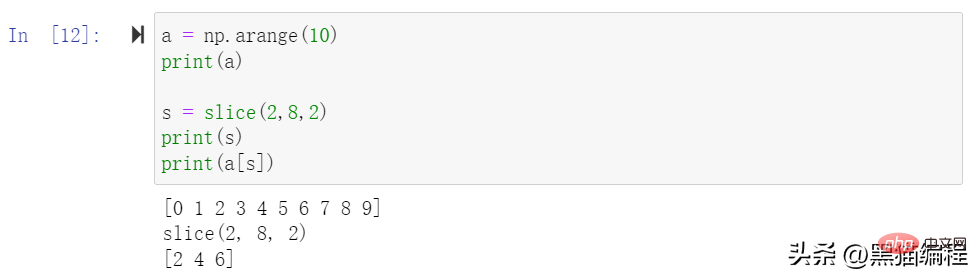
Numpy切片和索引ndarray对象的内容可以通过索引或切片来访问和修改,与 Python 中 list 的切片操作一样。ndarray 数组可以基于 0 ~ n-1 的下标进行索引,切片对象可以通过内置的 slice 函数,并设置 start, stop 及 step 参数进行,从原数组中切割出一个新数组。切片还可以包括省略号 …,来使选择元组的长度与数组的维度相同。 如果在行位置使用省略号,它将返回包含行中元素的 ndarray。高级索引整数数组索引以下实例获取数组中 (0,0),(1,1
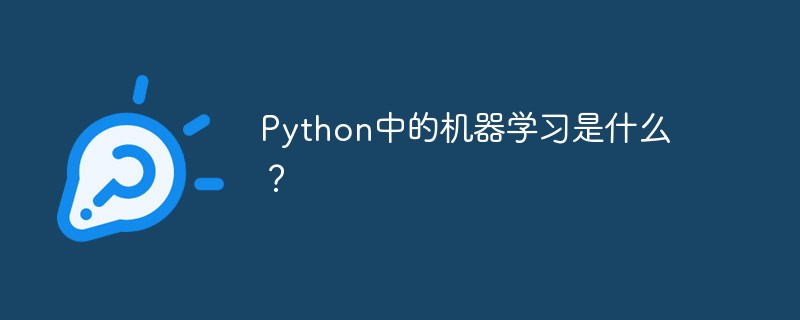
近年来,机器学习(MachineLearning)成为了IT行业中最热门的话题之一,Python作为一种高效的编程语言,已经成为了许多机器学习实践者的首选。本文将会介绍Python中机器学习的概念、应用和实现。一、机器学习概念机器学习是一种让机器通过对数据的分析、学习和优化,自动改进性能的技术。其主要目的是让机器能够在数据中发现存在的规律,从而获得对未来
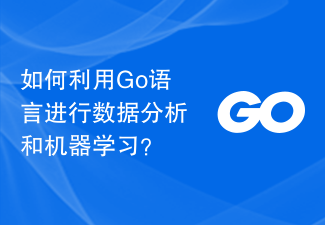
随着互联网技术的发展和大数据的普及,越来越多的公司和机构开始关注数据分析和机器学习。现在,有许多编程语言可以用于数据科学,其中Go语言也逐渐成为了一种不错的选择。虽然Go语言在数据科学上的应用不如Python和R那么广泛,但是它具有高效、并发和易于部署等特点,因此在某些场景中表现得非常出色。本文将介绍如何利用Go语言进行数据分析和机器学习
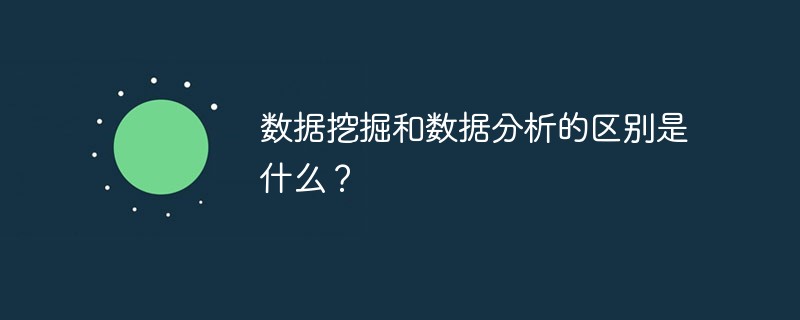
区别:1、“数据分析”得出的结论是人的智力活动结果,而“数据挖掘”得出的结论是机器从学习集【或训练集、样本集】发现的知识规则;2、“数据分析”不能建立数学模型,需要人工建模,而“数据挖掘”直接完成了数学建模。
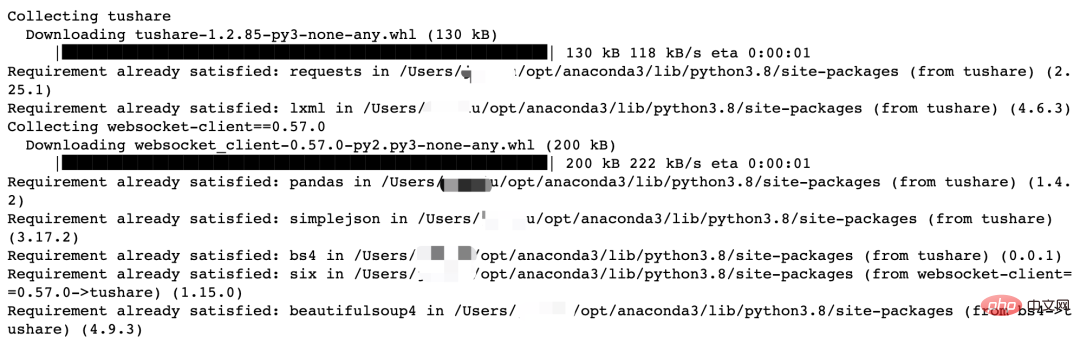
量化交易(也称自动化交易)是一种应用数学模型帮助投资者进行判断,并且根据计算机程序发送的指令进行交易的投资方式,它极大地减少了投资者情绪波动的影响。量化交易的主要优势如下:快速检测客观、理性自动化量化交易的核心是筛选策略,策略也是依靠数学或物理模型来创造,把数学语言变成计算机语言。量化交易的流程是从数据的获取到数据的分析、处理。数据获取数据分析工作的第一步就是获取数据,也就是数据采集。获取数据的方式有很多,一般来讲,数据来源主要分为两大类:外部来源(外部购买、网络爬取、免费开源数据等)和内部来源
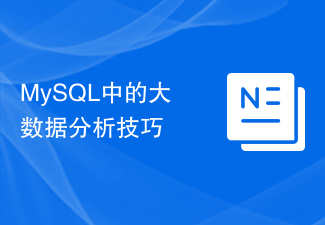
随着大数据时代的到来,越来越多的企业和组织开始利用大数据分析来帮助自己更好地了解其所面对的市场和客户,以便更好地制定商业策略和决策。而在大数据分析中,MySQL数据库也是经常被使用的一种工具。本文将介绍MySQL中的大数据分析技巧,为大家提供参考。一、使用索引进行查询优化索引是MySQL中进行查询优化的重要手段之一。当我们对某个列创建了索引后,MySQL就可
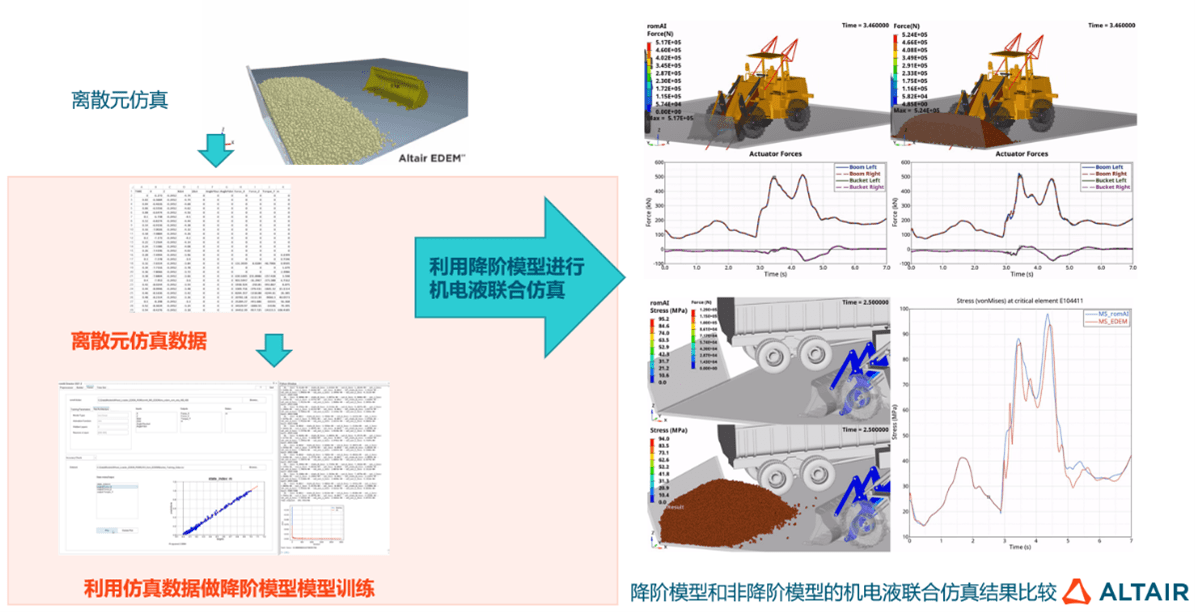
CAE和AI技术双融合已成为企业研发设计环节数字化转型的重要应用趋势,但企业数字化转型绝不仅是单个环节的优化,而是全流程、全生命周期的转型升级,数据驱动只有作用于各业务环节,才能真正助力企业持续发展。数字化浪潮席卷全球,作为数字经济核心驱动,数字技术逐步成为企业发展新动能,助推企业核心竞争力进化,在此背景下,数字化转型已成为所有企业的必选项和持续发展的前提,拥抱数字经济成为企业的共同选择。但从实际情况来看,面向C端的产业如零售电商、金融等领域在数字化方面走在前列,而以制造业、能源重工等为代表的传
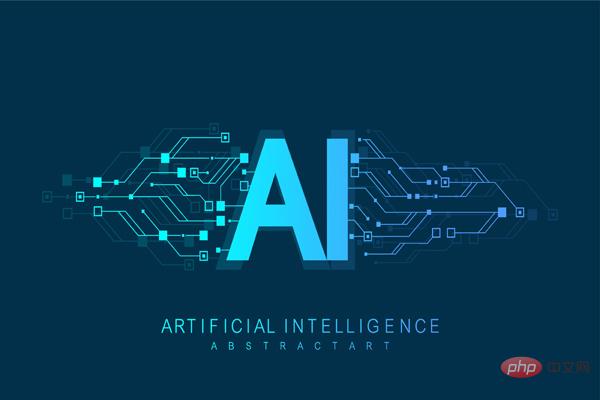
俄乌冲突爆发 2 周后,数据分析公司 Palantir 的首席执行官亚历山大·卡普 (Alexander Karp) 向欧洲领导人提出了一项建议。在公开信中,他表示欧洲人应该在硅谷的帮助下实现武器现代化。Karp 写道,为了让欧洲“保持足够强大以战胜外国占领的威胁”,各国需要拥抱“技术与国家之间的关系,以及寻求摆脱根深蒂固的承包商控制的破坏性公司与联邦政府部门之间的资金关系”。而军队已经开始响应这项号召。北约于 6 月 30 日宣布,它正在创建一个 10 亿美元的创新基金,将投资于早期创业公司和


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
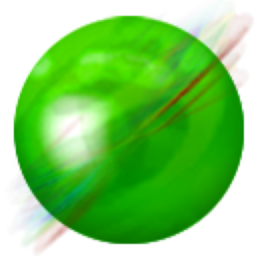
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
