Remove duplicates from sorted linked list using recursion
A linked list is a sequence of elements connected together. Each list has a header and a sequence of nodes, each of which holds data for the current node and links to the next node. The basic operations of linked lists are insertion, deletion, search and deletion.
Remove duplicates from sorted linked list
One way to delete nodes is to use recursion. The idea is to compare each node with its neighboring nodes and remove duplicate nodes where they are equal.
Our recursive call will return to the next node. So for the next element we will call the recursive function like current_node->next = our_function(node->next).
We trust our recursion and current_node->next now contains the linked list without any duplicate elements.
In the main method we bid on the list from scratch -
Node* head = new Node(5); head->next = new Node(5); head->next->next = new Node(6); head->next->next->next = new Node(7); head->next->next->next->next = new Node(7); head->next->next->next->next->next = new Node(7);
algorithm
The process of using recursion to remove duplicates from a sorted linked list is as follows.
Step 1 - Create a linked list with all values sorted in order
Step 2 - If the linked list does not exist, the program terminates.
Step 3 - If the linked list does exist, compare the next value of the head node with the value in the head node. If both values are the same, remove the header.
Step 4 - Step 3 is performed recursively, treating each node as a head until the list removes all duplicate values from itself .
Step 5 - The output obtained is a sorted linked list with different values
Example
For example, we have a sorted linked list with the following values -
1->1->1->2->3->3->4
Let's look at a C program that will remove duplicates from the above sorted linked list using recursion -
#include <iostream> using namespace std; class Node { public: int data; Node* next; Node(int data) { this->data = data; next = NULL; } }; Node* solve(Node* head) { if (head == NULL) return NULL; head->next = solve(head->next); if (head->next != NULL && head->next->data == head->data) { Node* temp = head->next; delete head; return temp; } return head; } void printList(Node* node) { while (node != NULL) { cout << node->data << (node->next == NULL ? "" : "->"); node = node->next; } } int main() { Node* head = new Node(1); head->next = new Node(1); head->next->next = new Node(1); head->next->next->next = new Node(2); head->next->next->next->next = new Node(3); head->next->next->next->next->next = new Node(3); head->next->next->next->next->next->next = new Node(4); cout << "Linked list before: "; printList(head); head = solve(head); cout << "\nLinked list after: "; printList(head); return 0; }
After that, we check whether the current node is included in the linked list. If the satisfying linked list we get from current node -> next node has the same value as that node, we don't include that node; otherwise, we include it.
Note - When the current node is NULL, we return the base condition of the recursion.
Output
Linked list before: 1->1->1->2->3->3->4 Linked list after: 1->2->3->4
in conclusion
As we saw with the recursive call, we trust that the next call will achieve the expected result of the rest of the problem. We just solved the current subproblem. With this in mind, we check if the current element can be contained and hand the rest of the linked list over to our recursive call, trusting it to give us a valid linked list from that point on. When we traverse the entire linked list, the time complexity of our method is O(n).
The above is the detailed content of Remove duplicates from sorted linked list using recursion. For more information, please follow other related articles on the PHP Chinese website!
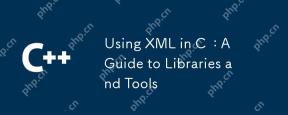
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
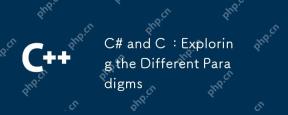
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
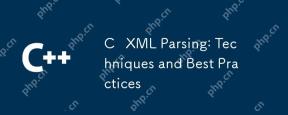
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
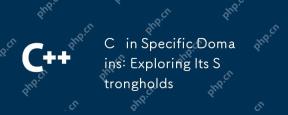
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
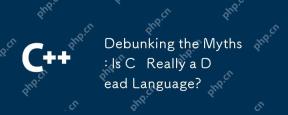
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
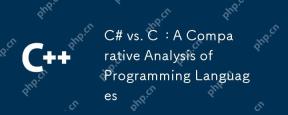
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
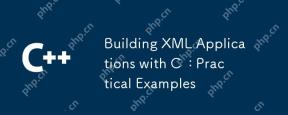
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
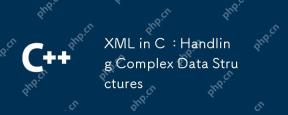
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
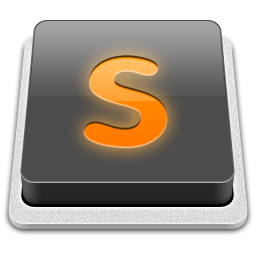
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version
