


We get an array of integer type containing positive and negative numbers, say, arr[] of any given size. The task is to rearrange the array in such a way that all elements at even positions or indices should be larger than elements at odd positions or indices, and Print the results.
Let's look at various input and output scenarios for this -
Input− int arr[] = {2, 1, 4, 3, 6, 5, 8, 7}
Output− Array before sorting: 2 1 4 3 6 5 8 7 Rearrange the array so that even positions are larger than odd positions: 1 2 3 4 5 6 7 8
Explanation− We get an integer array of size 8, which contains positive and negative elements . Now, we rearrange the array so that all elements in even positions are larger than elements in odd positions. The resulting array is 1 2 3 4 5 6 7 8.
Input− int arr[] = {-3, 2, -4, -1}
Output− Array before arrangement: - 3 2 -4 -1 Rearrange an array so that even positions are larger than odd ones: -4 -3 -1 2
Explanation - We get an integer array of size 8 containing positive and negative elements. Now, we rearrange the array so that all elements at even positions are larger than elements at odd positions. The resulting array after doing this is -4 -3 -1 2.
The following program uses the following method-
Input an array of integer elements and calculate the size of the array.
li> Sort an array using the C STL's sort method by passing the array and the size of the array to the sort function.
Declare an integer variable and set it by calling the function Rearrangement(arr, size)
-
In the function Rearrangement(arr, size) Inside
Declare an integer type array, assuming that the size of ptr[size] is the same as the array arr[size]
Declare a temporary integer type variable , i.e. the first one is 0 and the last one is of size -1.
Loop FOR from i to 0 until i is less than the size of the array. Inside the loop, check IF (i 1) % 2 equals 0, then set ptr[i] to arr[last--].
ELSE, set ptr[i ] to arr[first ].
Print the result.
Example#include <bits/stdc++.h>
using namespace std;
void Rearrangement(int* arr, int size){
int ptr[size];
int first = 0;
int last = size - 1;
for (int i = 0; i < size; i++){
if((i + 1) % 2 == 0){
ptr[i] = arr[last--];
}
else{
ptr[i] = arr[first++];
}
}
}
int main(){
//input an array
int arr[] = {2, 1, 4, 3, 6, 5, 8, 7};
int size = sizeof(arr) / sizeof(arr[0]);
//print the original Array
cout<<"Array before Arrangement: ";
for (int i = 0; i < size; i++){
cout << arr[i] << " ";
}
//sort an Array
sort(arr, arr + size);
//calling the function to rearrange the array
Rearrangement(arr, size);
//print the array after rearranging the values
cout<<"\nRearrangement of an array such that even positioned are greater than odd is: ";
for(int i = 0; i < size; i++){
cout<< arr[i] << " ";
}
return 0;
}
Output
If we run the above code it will generate the following output
Array before Arrangement: 2 1 4 3 6 5 8 7 Rearrangement of an array such that even positioned are greater than odd is: 1 2 3 4 5 6 7 8
The above is the detailed content of Rearrange an array so that elements in even positions are larger than elements in odd positions (C++). For more information, please follow other related articles on the PHP Chinese website!
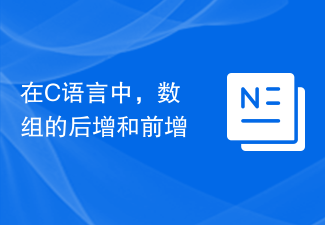
问题使用C程序解释数组的后置递增和前置递增的概念。解决方案递增运算符(++)-用于将变量的值增加1有两种类型的递增运算符-前置递增和后置递增。在前置递增中,递增运算符放在操作数之前,值先递增,然后进行操作。eg:z=++a;a=a+1z=a自增运算符在后增运算中放置在操作数之后,操作完成后值会增加。eg:z=a++;z=aa=a+1让我们考虑一个例子,通过使用前增量和后增量来访问内存位置中的特定元素。声明一个大小为5的数组并进行编译时初始化。之后尝试将前增量值赋给变量'a'。a=++arr[1]
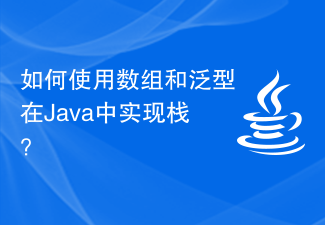
Java通过利用数组和泛型来实现堆栈。这创建了一个多功能且可重用的数据结构,该结构按照后进先出(LIFO)的原则运行。按照这个原则,元素是从顶部添加和删除的。通过利用数组作为基础,它确保了高效的内存分配和访问。此外,通过合并泛型,堆栈能够容纳不同类型的元素,从而增强其多功能性。该实现涉及包含泛型类型参数的Stack类的定义。它包括基本方法,如push()、pop()、peek()和isEmpty()。边缘情况的处理(例如堆栈溢出和下溢)对于确保无缝功能也至关重要。此实现使开发人员能够创建能够容纳
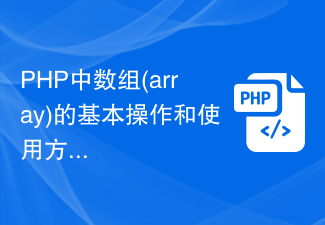
PHP中数组(array)的基本操作和使用方法一、概述数组是PHP中一种非常重要的数据类型,它可以用于存储多个值,并且可以通过索引或者键来访问这些值。数组在PHP中拥有丰富的操作和使用方法,本文将详细介绍PHP中数组的基本操作和使用方法。二、创建数组在PHP中,可以通过两种方式来创建数组:可数数组和关联数组。创建可数数组可数数组是按顺序排列并以数字索引的数组
![重新排列一个数组,使得arr变为arr],并且只使用O(1)额外的空间,使用C++实现](https://img.php.cn/upload/article/000/000/164/169319478769496.jpg)
我们得到一个正整数类型数组,比方说,任意给定大小的arr[],这样数组中的元素值应大于0但小于数组的大小。任务是重新排列一个数组,仅在给定的O(1)空间内将arr[i]变为arr[arr[i]]并打印最终结果。让我们看看这种情况的各种输入输出场景−输入−intarr[]={032154}输出−排列前的数组:032154重新排列数组,使arr[i]变为arr[arr[i]],并具有O(1)额外空间:012345解释−我们给定一个大小为6的整数数组,并且数组中的所有元素值小于6。现在,我们将重新排列
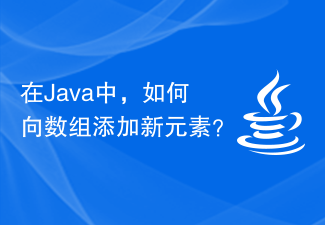
Java中向数组中添加新元素是一种常见的操作,可以使用多种方法实现。本文将介绍几种常见的添加元素到数组的方法,并提供相应的代码示例。一、使用新数组一种常见的方法是创建一个新的数组,将原数组的元素复制到新数组中,并在新数组的末尾添加新元素。具体步骤如下:创建一个新的数组,大小比原数组大1。这是因为要添加一个新元素。将原数组的元素复制到新数组中。在新数组的末尾添
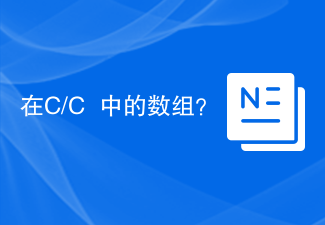
数组是相同类型元素的顺序集合。数组用于存储数据的集合,但将数组视为相同类型的变量的集合通常更有用。而不是声明单个变量,例如number0、number1、...和number99,您可以声明一个数组变量(例如数字),并使用numbers[0]、numbers[1]和...、numbers[99]来表示各个变量。数组中的特定元素通过索引访问。所有数组都由连续的内存位置组成。最低地址对应于第一个元素,最高地址对应于最后一个元素。声明数组声明数组需要指定元素的类型以及所需元素的数量。一个数组如下-ty
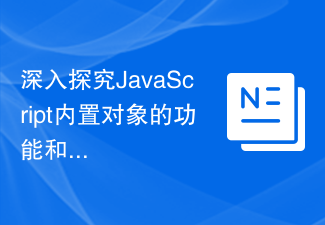
深入解析JS内置对象的功能与特点JavaScript是一门基于对象的编程语言,它提供了许多内置对象,这些对象拥有各种丰富的功能和特点。在本文中,我们将深入解析一些常用的内置对象,并给出相应的代码示例。Math对象Math对象提供了一些基本的数学运算方法,如求幂、开方、对数等。以下是一些常用的Math对象的方法示例://求绝对值Math.abs(-10
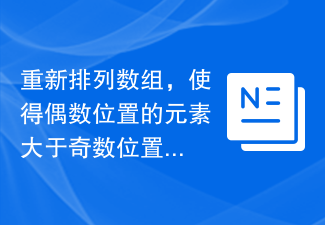
我们得到一个包含正数和负数的整数类型数组,比方说,任意给定大小的arr[]。任务是以这样的方式重新排列数组,使得偶数位置或索引处的所有元素都应大于奇数位置或索引处的元素,并且打印结果。让我们看看这个的各种输入输出场景-输入−intarr[]={2,1,4,3,6,5,8,7}输出−排列前的数组:21436587重新排列数组,使得偶数位置大于奇数位置:12345678解释−我们得到一个大小为8的整数数组,其中包含正数和负面因素。现在,我们将数组重新排列,使得偶数位置的所有元素都大于奇数位置的元素,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
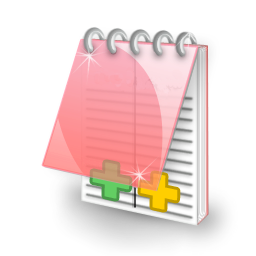
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
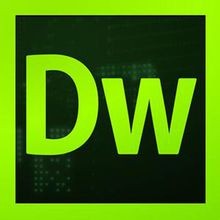
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
