In Python, we have some built-in time functions such as strftime() and datetime.now() which can be used to find the time in AM/PM format. Time in AM/PM format is used in a variety of applications such as user interfaces, reporting and documentation, data visualization, and event scheduling. When the time is between 11:59:00 midnight and 12 noon, we say AM time. Similarly, we can say that the time between 12 o'clock and 11:59:00 midnight is PM. The abbreviations AM/PM are used to indicate the exact time.
Syntax
The following syntax is used in the example &miinus;
strftime('%I:%M:%S %p')
strftime() is a built-in function in Python that can be used to represent time format.
The following format is represented in the parameters:
%I − Hours
%M − Minutes
%S − Seconds
%p − AM/PM
datetime.now()
This is a built-in function in Python that can be used to find the current time.
localtime()
This is the built-in method in Python that returns the current time.
Example 1
In the example below we will start the program by importing everything for datetime from a module called datetime which will find the local time. Then store the time in 24 hour format in variable t_str, the time will be converted to 12 hour format and check if it is AM or PM. Now we are using the built-in function strftime(). This function accepts two parameters - t_str (the given time) and '%H:%M%S' (set the format of the time, including hours, minutes and seconds). This time format represents the 24-hour clock. Next, we use the strftime() function again to format the time to 12-hour time. In the parameters, %p is used to check whether the time is AM or PM. Finally, we use the variables t_str and t_am_pm to print the results.
from datetime import datetime t_str = '22:45:32' t_obj = datetime.strptime( t_str, '%H:%M:%S') #Time format representation t_am_pm = t_obj.strftime('%I:%M:%S %p') print("The given time", t_str) print("The format in (AM/PM):", t_am_pm)
Output
The given time 22:45:32 The format in (AM/PM): 10:45:32 PMThe Chinese translation of
Example 2
is:Example 2
In the example below we will start the program by importing everything for datetime from a module called datetime which will find the local time. Then, we create the variable dt_obj to store the value of the current time obtained by using the predefined function datetime.now(). Then use the predefined function strftime() to set the 12-hour time format. This function will be used as an object with the variable dt_obj and stored in the variable ts_am_pm. Finally, we print the results with the help of variables dt_obj and ts_am_pm.
from datetime import datetime dt_obj = datetime.now() ts_am_pm = dt_obj.strftime('%I:%M:%S %p') print("The current time:",dt_obj) print("The format in (AM/PM):",ts_am_pm)
Output
The current time: 2023-04-18 17:50:01.963742 The format in (AM/PM): 05:50:01 PMThe Chinese translation of
Example 3
is:Example 3
In the following example, we will write a program using a function called format_time(), which takes a datetime object as a parameter and returns a string representing the time, displayed in AM/PM format. The function then uses the strftime() method of the datetime object to format the time according to the specified format string '%I:%M %p'. This format specifies a timetable in 12-hour format (%I), including minutes (%M) and AM/PM indicators (%p).
from datetime import datetime def p_time(time): return time.strftime('%I:%M %p') time = datetime.now() time_format = p_time(time) print("Time in AM/PM:",time_format)
Output
Time in AM/PM: 06:04 PMThe Chinese translation of
Example 4
is:Example 4
In the following example, we first import the module named time, which defines the time to set the current time and allows the user to format the time using built-in methods. Then a variable named present_time that stores the value is initialized by using the built-in method localtime(). We then use the strftime method to format the time into AM/PM format using the format string "%I:%M %p". The %I format code represents the hour in the 12-hour format, %M represents the minute, and %p represents AM or PM, and is stored in the variable t_format. Finally, we print the result using the variable t_format.
import time present_time = time.localtime() t_format = time.strftime("%I:%M %p", present_time) print(t_format)
Output
06:18 PM
Conclusion
We see that the built-in function strftime() can help represent the AM or PM format of time. In Example 1, we discussed the method of converting 24-hour format to 12-hour format to check whether the time mode is AM/PM. In Example 2, we see how to initialize the current time using the datetime.now() function. Then use %p to check if the time is AM/PM.
The above is the detailed content of Python program to format time in AM-PM format. For more information, please follow other related articles on the PHP Chinese website!
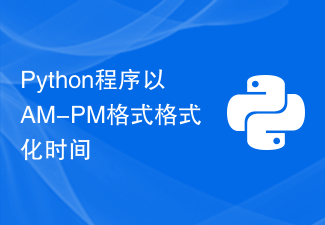
在Python中,我们有一些内置的时间函数,例如strftime()和datetime.now(),可以用来以AM/PM格式找到时间。AM/PM格式的时间在各种应用程序中使用,如用户界面、报告和文档、数据可视化和事件调度。当时间在午夜11:59:00到中午12点之间时,我们会说AM的时间。同样地,我们可以说12点到午夜11:59:00之间的时间是PM。缩写AM/PM用于指示确切的时间。Syntax在示例中使用了以下语法&miinus;strftime('%I:%M:%S%p')strft
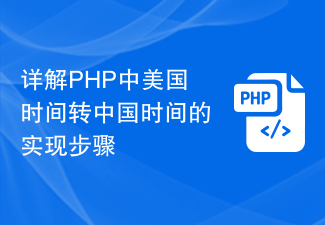
PHP是一种常用的程序设计语言,用于开发Web应用程序。在开发Web应用程序的过程中,可能涉及到不同时区时间的转换,比如将美国时间转换为中国时间。本文将详细介绍如何使用PHP实现将美国时间转换为中国时间的具体步骤,并提供代码示例。1.获取美国时间首先,我们需要获取美国时间。可以使用PHP的内置函数date_default_timezone_set来设置时区
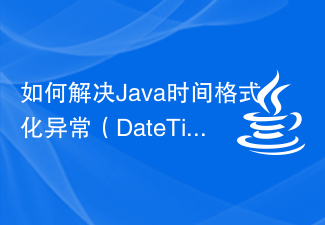
如何解决Java时间格式化异常(DateTimeFormatException)引言:Java是一种广泛应用的编程语言,在处理日期和时间时经常会遇到格式化异常(DateTimeFormatException)。本文将介绍如何解决Java中的时间格式化异常,并提供一些代码示例。一、什么是时间格式化异常(DateTimeFormatException)在Java
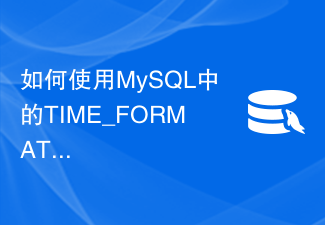
如何使用MySQL中的TIME_FORMAT函数将时间格式化为特定的字符串MySQL是一个广泛使用的关系型数据库管理系统,它提供了丰富的函数和操作符来处理数据。在MySQL中,有一个非常有用的函数,即TIME_FORMAT函数,可以将时间按照指定的格式进行格式化并返回一个字符串。TIME_FORMAT函数的基本语法如下:TIME_FORMAT(time,f
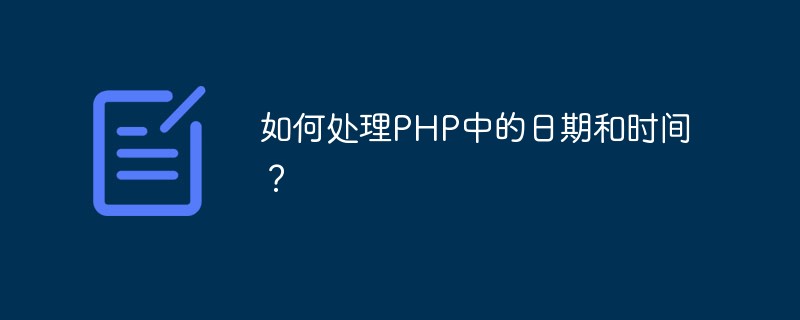
在Web开发中,日期和时间是非常重要的因素,尤其是针对交互和数据存储。在PHP中,处理日期和时间的功能非常强大,比如获取当前时间、将时间戳转换成日期时间格式、比较两个日期时间等等。在本篇文章中,将介绍如何处理PHP中的日期和时间。获取当前时间在PHP中,获取当前时间的函数是date()。该函数有两个参数,第一个参数是日期时间格式,第二个参数是可选的时间戳。以
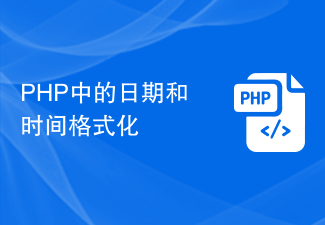
在开发网站时,您经常需要使用日期和时间。例如,您可能需要显示帖子的最后修改日期或提及读者发表评论的时间。您可能还需要显示特殊事件发生之前的倒计时。幸运的是,PHP附带了一些内置的日期和时间函数,这将帮助我们轻松完成所有这些工作。本教程将教您如何在PHP中设置当前日期和时间的格式。您还将学习如何从日期字符串获取时间戳以及如何添加和减去不同的日期。获取字符串格式的日期和时间date($format,$timestamp)是PHP中最常用的日期和时间函数之一。它将所需的日期输出格式作为第一个参数,并将
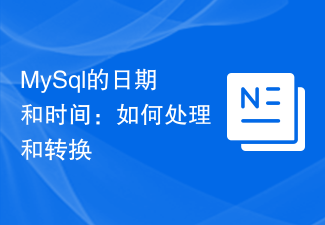
MySql是一款常用的关系型数据库管理系统,它支持多种日期和时间类型的数据存储和操作。本文将介绍如何在MySql中处理和转换日期和时间数据。一、日期和时间类型MySql支持多种日期和时间类型,包括DATE、TIME、DATETIME、TIMESTAMP等。这些类型的具体定义如下:DATE:表示日期,格式为'YYYY-MM-DD'。有效范围为'1000-01-
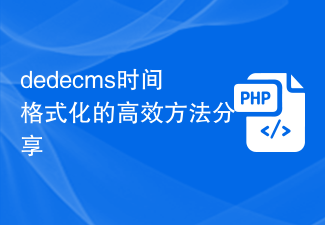
在使用DedeCMS进行网站开发过程中,经常会遇到对时间格式进行处理的情况。在进行时间格式化时,我们常常会用到PHP中的date()函数,但有时候需要更灵活、高效的方法来处理时间格式。本文将分享一些高效的DedeCMS时间格式化方法,帮助开发者更好地处理时间数据。首先,我们可以在模板或插件中使用dede对时间进行格式化,下面是一个简单的示例代码://获取时


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
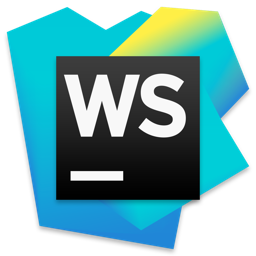
WebStorm Mac version
Useful JavaScript development tools
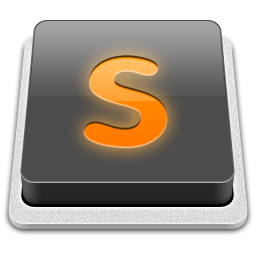
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
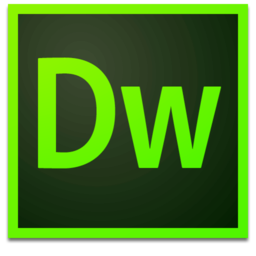
Dreamweaver Mac version
Visual web development tools
