Given an array of N integers and Q range queries. For each query, we need to return the XOR of the largest odd divisor of each number in the range.
The largest odd divisor is the largest odd number that can divide the number N, such as . For example, the largest odd divisor of 6 is 3.
Input: nums[ ] = { 3, 6, 7, 10 }, query[ ] = { { 0, 2 }, { 1, 3 } } Output: query1: 7 query2: 1 Explanation: greatest odd divisors of nums array are { 3, 3, 7, 5 }. For query 1 we need to find the XOR of indexes 0, 1, and 2 which is 7, and for query2 we need to find XOR of indexes 1, 2, and 3 which is 1.
Solution method
Simple method
First, in the simple method, we need to find the largest odd divisor of all array elements. Then based on the range of the query, we need to calculate the XOR of each element in the range and return it.
Efficient method
An effective way to solve this problem is to create a prefix XOR array prefix_XOR[] containing the array with the largest odd divisor, instead of each number in the range each time Performs an XOR and returns prefix_XOR[R] - prefix_XOR[L-1].
Prefix XOR array is an array in which each element contains the XOR of all previous elements.
Example
#include <bits/stdc++.h> using namespace std; int main(){ int nums[] = { 3, 6, 7, 10 }; int n = sizeof(nums) / sizeof(nums[0]); int prefix_XOR[n]; // creating an array // containing Greatest odd divisor of each element. for (int i = 0; i < n; i++) { while (nums[i] % 2 != 1) nums[i] /= 2; prefix_XOR[i] = nums[i]; } // changing prefix_XOR array to prefix xor array. for (int i = 1; i < n; i++) prefix_XOR[i] = prefix_XOR[i - 1] ^ prefix_XOR[i]; // query array to find result of these queries. int query[2][2] = {{0, 2},{1, 3}}; int q = sizeof(query) / sizeof(query[0]); // finding results of queries. for(int i = 0;i<q;i++){ if (query[i][0] == 0) cout<< prefix_XOR[query[i][1]] << endl; else{ int result = prefix_XOR[query[0][1]] ^ prefix_XOR[query[i][0] - 1]; cout << result << endl; } } return 0; }
Output
7 4
Description of the above code
Create a prefix_XOR array to store the largest odd divisor of each element, Then change this array to a prefix XOR array.
The largest odd divisor is calculated by dividing it by two until modulo 2 gives 1.
Create a prefix XOR array by traversing the array and performing a bitwise XOR of the current element with the previous element.
The query result is calculated by subtracting the right index of the prefix_XOR[] array (left - 1) The index of the prefix_XOR[] array.
Conclusion
In this tutorial, we discussed a problem where we need to find the largest odd divisor for each number in the range of a given array. We discussed a way to solve this problem by finding the largest odd divisor for each element and using a prefix XOR array. We also discussed C program for this problem and we can do this using programming languages like C, Java, Python etc. We hope this article was helpful to you.
The above is the detailed content of XOR query for maximum odd divisor in range in C++. For more information, please follow other related articles on the PHP Chinese website!
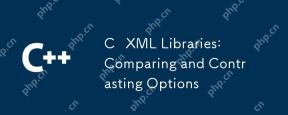
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
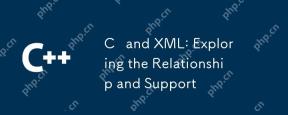
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
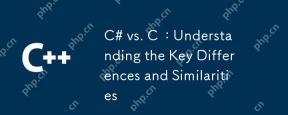
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
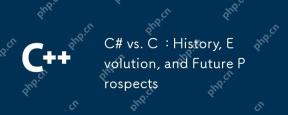
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
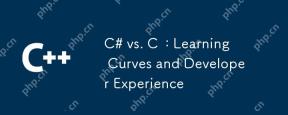
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
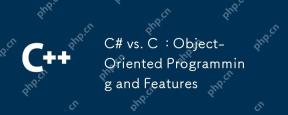
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
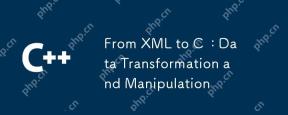
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
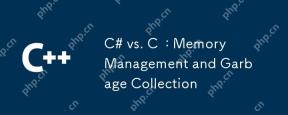
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
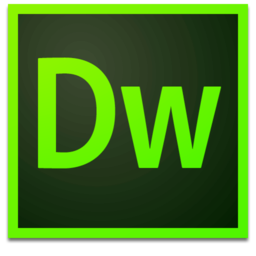
Dreamweaver Mac version
Visual web development tools
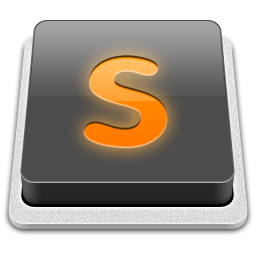
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
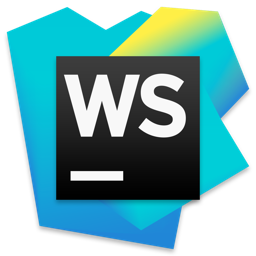
WebStorm Mac version
Useful JavaScript development tools