


How to implement intelligent health management applications through C++ development?
How to develop intelligent health management applications through C?
Smart health management applications are a type of applications that have emerged in recent years as people's health awareness increases. They can help users record and manage health-related data, provide health advice and early warning information and other functions. In this article, we will use C as the development language to introduce how to develop a simple intelligent health management application.
First of all, we need to clarify the functional requirements of the application. A typical smart health management application should include the following functions:
- User registration and login: Users can register an account and log in to use the application.
- Health data recording: Users can record their height, weight, blood pressure, heart rate and other health data.
- Health data display: The application can display the health data recorded by the user and provide functions such as charts and statistical analysis.
- Health suggestions and warnings: The application can provide corresponding health suggestions and warning information based on the user's health data.
Next, we will introduce how to implement the above functions through C language.
- User registration and login:
In C, file storage can be used to simulate user registration and login functions. We can define a User class to represent the user and use files as the user's storage medium. User registration and login can be achieved by reading and writing files.
#include <iostream> #include <fstream> #include <string> class User { public: User(const std::string& username, const std::string& password) : username(username), password(password) {} std::string getUsername() const { return username; } std::string getPassword() const { return password; } bool saveToFile() const { std::ofstream file(username + ".txt"); if (!file.is_open()) { return false; } file << password; file.close(); return true; } static User* loadFromFile(const std::string& username) { std::ifstream file(username + ".txt"); if (!file.is_open()) { return nullptr; } std::string password; file >> password; file.close(); return new User(username, password); } private: std::string username; std::string password; }; int main() { // 用户注册 User user("admin", "password"); if (!user.saveToFile()) { std::cout << "Failed to save user to file" << std::endl; return 1; } // 用户登录 std::string username, password; std::cout << "Username: "; std::cin >> username; std::cout << "Password: "; std::cin >> password; User* loadedUser = User::loadFromFile(username); if (loadedUser == nullptr || loadedUser->getPassword() != password) { std::cout << "Login failed" << std::endl; return 1; } // 用户登录成功 std::cout << "Welcome, " << loadedUser->getUsername() << "!" << std::endl; delete loadedUser; return 0; }
- Health data record:
We can use a HealthRecord class to represent the user's health record. This class can contain attributes such as height, weight, blood pressure, heart rate, etc., and provides methods to modify and read these attributes.
#include <iostream> #include <string> class HealthRecord { public: HealthRecord(double height, double weight, int bloodPressure, int heartRate) : height(height), weight(weight), bloodPressure(bloodPressure), heartRate(heartRate) {} double getHeight() const { return height; } double getWeight() const { return weight; } int getBloodPressure() const { return bloodPressure; } int getHeartRate() const { return heartRate; } void setHeight(double newHeight) { height = newHeight; } void setWeight(double newWeight) { weight = newWeight; } void setBloodPressure(int newBloodPressure) { bloodPressure = newBloodPressure; } void setHeartRate(int newHeartRate) { heartRate = newHeartRate; } private: double height; double weight; int bloodPressure; int heartRate; }; int main() { HealthRecord record(175.0, 70.0, 120, 80); std::cout << "Height: " << record.getHeight() << std::endl; std::cout << "Weight: " << record.getWeight() << std::endl; std::cout << "Blood pressure: " << record.getBloodPressure() << std::endl; std::cout << "Heart rate: " << record.getHeartRate() << std::endl; record.setHeight(180.0); std::cout << "Updated height: " << record.getHeight() << std::endl; return 0; }
- Health data display:
For the display of health data, you can use C chart library (such as matplotplusplus) to draw charts, and use data analysis libraries (such as Boost) to draw charts. conduct statistical analysis. Here we use a simple example to show how to use these libraries.
#include <iostream> #include "matplot/matplot.h" int main() { std::vector<double> heights = {165, 170, 175, 180}; std::vector<double> weights = {60, 65, 70, 75}; // 绘制身高和体重的散点图 auto scatter = matplot::scatter(heights, weights); scatter->marker_size(weights).marker(matplot::marker::circle).line_width(2); matplot::xlabel("Height"); matplot::ylabel("Weight"); matplot::show(); return 0; }
- Health recommendations and early warnings:
The implementation of health recommendations and early warnings usually requires a combination of medical knowledge and technologies such as rule engines. In C, we can use if statements or switch statements to provide corresponding suggestions and warning information based on health data.
#include <iostream> #include <string> void provideHealthAdvice(double weight, int heartRate) { if (weight > 80) { std::cout << "You are overweight. Please consider losing weight." << std::endl; } if (heartRate > 100) { std::cout << "Your heart rate is too high. Please consult a doctor." << std::endl; } } int main() { double weight; int heartRate; std::cout << "Weight: "; std::cin >> weight; std::cout << "Heart rate: "; std::cin >> heartRate; provideHealthAdvice(weight, heartRate); return 0; }
Through C language, we can implement a simple intelligent health management application. The application can meet basic functions such as user registration and login, health data recording, health data display, and health advice and warnings. Of course, in order to achieve more complete and rich intelligent health management applications, we can also use other related technologies and tools, such as databases, artificial intelligence, etc. I hope this article will help you understand how to use C to develop intelligent health management applications.
The above is the detailed content of How to implement intelligent health management applications through C++ development?. For more information, please follow other related articles on the PHP Chinese website!
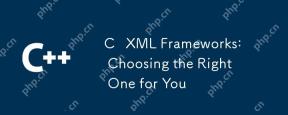
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
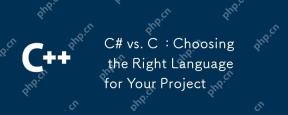
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
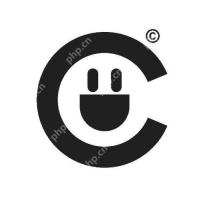
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
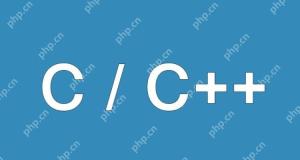
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
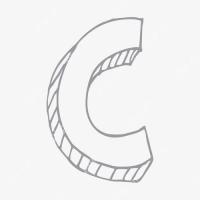
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
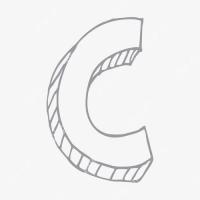
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
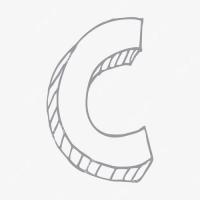
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
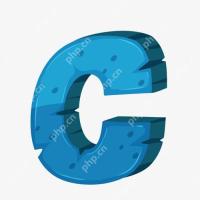
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
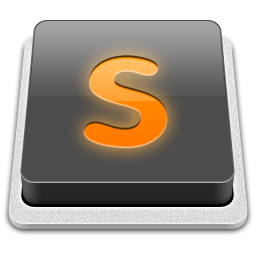
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
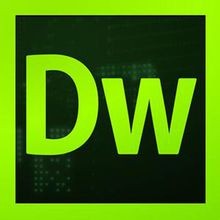
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
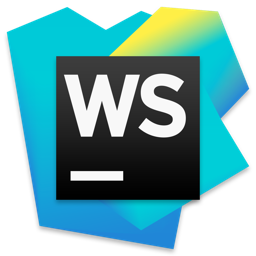
WebStorm Mac version
Useful JavaScript development tools
