How to optimize data caching strategy in C++ big data development?
How to optimize the data caching strategy in C big data development?
In big data development, data caching is a commonly used optimization method. By loading frequently accessed data into memory, program performance can be greatly improved. This article will introduce how to optimize data caching strategy in C and give relevant code examples.
1. Use LRU caching algorithm
LRU (Least Recently Used) is a commonly used caching algorithm. Its principle is to put the most recently used data at the front of the cache, and the least frequently used data at the back of the cache. When the cache is full, if the newly added data is not in the cache, the least frequently used data is deleted and the new data is placed at the front of the cache. We can use list and unordered_map in STL to implement the LRU cache algorithm. The specific implementation is as follows:
#include <list> #include <unordered_map> template <typename Key, typename Value> class LRUCache { public: LRUCache(int capacity) : m_capacity(capacity) {} Value get(const Key& key) { auto it = m_map.find(key); if (it == m_map.end()) { return Value(); } m_list.splice(m_list.begin(), m_list, it->second); return it->second->second; } void put(const Key& key, const Value& value) { auto it = m_map.find(key); if (it != m_map.end()) { it->second->second = value; m_list.splice(m_list.begin(), m_list, it->second); return; } if (m_map.size() == m_capacity) { auto last = m_list.back(); m_map.erase(last.first); m_list.pop_back(); } m_list.emplace_front(key, value); m_map[key] = m_list.begin(); } private: int m_capacity; std::list<std::pair<Key, Value>> m_list; std::unordered_map<Key, typename std::list<std::pair<Key, Value>>::iterator> m_map; };
2. Pre-read data
In big data processing, there are usually many continuous data accesses. In order to reduce IO overhead, we can pre-read a certain amount of data into memory during program execution. The following is a simple sample code for pre-reading data:
#include <fstream> #include <vector> void preReadData(const std::string& filename, size_t cacheSize, size_t blockSize) { std::ifstream file(filename, std::ios::binary); if (!file) { return; } std::vector<char> cache(cacheSize, 0); while (!file.eof()) { file.read(&cache[0], blockSize); // 处理读取的数据 } file.close(); }
The above code will read the file into a buffer according to the specified block size and then process it. By adjusting cacheSize and blockSize, optimization can be performed according to the actual situation.
3. Use multi-threading and asynchronous IO
In big data processing, IO operations are often one of the bottlenecks of program performance. In order to improve IO efficiency, multi-threading and asynchronous IO can be used. The following is a sample code that uses multiple threads to read data:
#include <iostream> #include <fstream> #include <vector> #include <thread> void readData(const std::string& filename, int start, int end, std::vector<char>& data) { std::ifstream file(filename, std::ios::binary); if (!file) { return; } file.seekg(start); int size = end - start; data.resize(size); file.read(&data[0], size); file.close(); } void processLargeData(const std::string& filename, int numThreads) { std::ifstream file(filename, std::ios::binary); if (!file) { return; } file.seekg(0, std::ios::end); int fileSize = file.tellg(); file.close(); int blockSize = fileSize / numThreads; std::vector<char> cache(fileSize, 0); std::vector<std::thread> threads; for (int i = 0; i < numThreads; ++i) { int start = i * blockSize; int end = (i + 1) * blockSize; threads.emplace_back(readData, std::ref(filename), start, end, std::ref(cache)); } for (auto& t : threads) { t.join(); } // 处理读取的数据 }
The above code will use multiple threads to read different parts of the file at the same time, and then merge the data into a buffer area for processing. By adjusting the number of numThreads, optimization can be performed according to the actual situation.
Summary
In C big data development, optimizing the data caching strategy can significantly improve the performance of the program. This article introduces methods of using the LRU cache algorithm, reading data ahead, and using multi-threading and asynchronous IO. Readers can choose appropriate optimization methods according to their own needs and scenarios, and practice them with specific code examples.
References:
- https://en.wikipedia.org/wiki/Cache_replacement_policies
- https://www.learncpp.com/cpp-tutorial /182-reading-and-writing-binary-files/
The above is the detailed content of How to optimize data caching strategy in C++ big data development?. For more information, please follow other related articles on the PHP Chinese website!
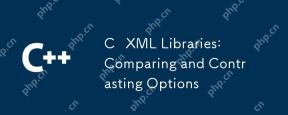
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
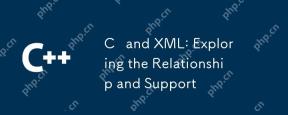
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
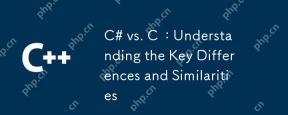
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
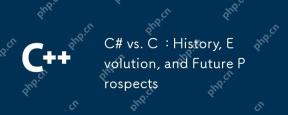
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
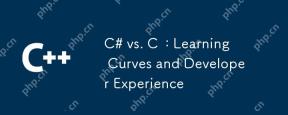
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
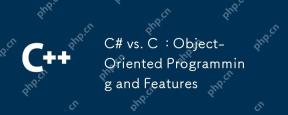
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
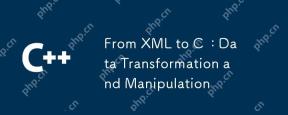
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
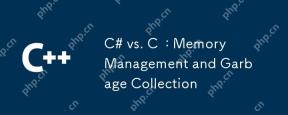
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
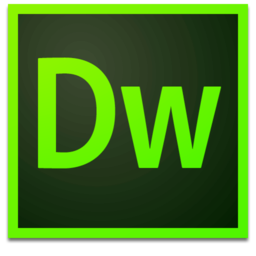
Dreamweaver Mac version
Visual web development tools
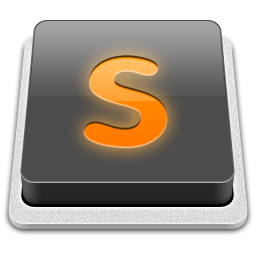
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
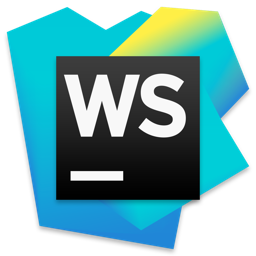
WebStorm Mac version
Useful JavaScript development tools