A BitArray is a collection of Boolean values represented as a series of 1's and 0's. It is often used to store and manipulate binary data efficiently. In C#, the BitArray class is part of the System. Collections namespace, and it allows you to manipulate the individual bits in the array using bitwise operators.
Reverse all bit values in the bit array
In C#, to reverse all bit values in a BitArray, you can use the exclusive-OR (XOR) operator (^) with the number 1. This operator returns a value of 1 if the compared bits are different and a value of 0 if they are the same. By applying this operator to each bit in a BitArray, all bit values can be reversed.
The Chinese translation ofExample 1
is:Example 1
The following example demonstrates how to invert all bit values in a BitArray in C
#algorithm
Step 1 - Create a new BitArray to store the inverted value.
Step 2 - Loop through each bit in the original BitArray.
Step 3 − Use the bitwise negation operator (~) to invert the value of each bit.
Step 4 - Store the inverted value in a new BitArray.
Step 5 - Return the new BitArray.
using System; using System.Collections; class Program{ static void Main(string[] args){ // Create a new BitArray with some initial values BitArray bits = new BitArray(new[] { true, false, true, false }); // Invert all the bit values using XOR with 1 for (int i = 0; i < bits.Length; i++){ bits[i] ^= true; } // Print the inverted bit values for (int i = 0; i < bits.Length; i++){ Console.Write(bits[i] ? "1" : "0"); } Console.ReadLine(); } }
Output
When you run the above code output, this output corresponds to the inverted bit value of the original BitArray (1010).
0101The Chinese translation of
Example 2
is:Example 2
The following example demonstrates reversing all bit values in a BitArray in C
#algorithm
Step 1 − Create a BitArray object with the desired size.
Step 2 - Loop through each index in the BitArray.
Step 3 - Use the BitArray.Set method to invert the value at the current index. The parameters are the index and the inverse value of the current index.
using System; using System.Collections; class Program { static void Main(string[] args) { int size = 8; BitArray bits = new BitArray(size); for (int i = 0; i < size; i++) { bits[i] = (i % 2 == 0); } Console.WriteLine("Before inversion:"); PrintBits(bits); InvertBits(bits); Console.WriteLine("After inversion:"); PrintBits(bits); } static void InvertBits(BitArray bits) { for (int i = 0; i < bits.Count; i++) { bits.Set(i, !bits[i]); } } static void PrintBits(BitArray bits) { for (int i = 0; i < bits.Count; i++) { Console.Write(bits[i] ? "1" : "0"); } Console.WriteLine(); } }
Output
Before inversion: 01010101 After inversion: 10101010
in conclusion
In C#, inverting all bit values in a BitArray is a simple task and can be accomplished using the XOR operator with the value 1. This technique is very useful when working with binary data and helps you manipulate individual bits in an array efficiently.
The above is the detailed content of Negate all bit values in BitArray in C#. For more information, please follow other related articles on the PHP Chinese website!
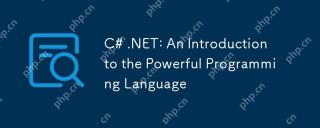
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
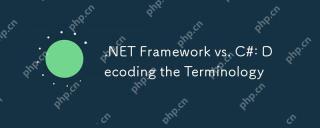
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
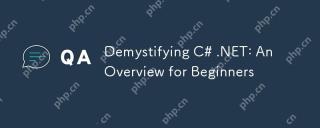
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
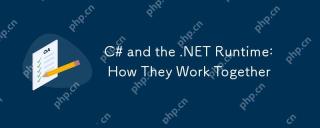
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
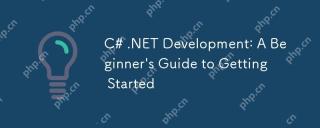
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
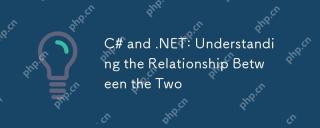
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
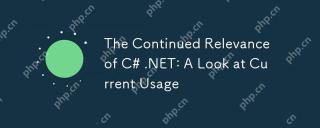
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
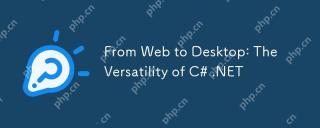
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
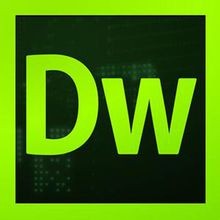
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool