C is an efficient programming language that can handle various types of data. It is suitable for processing large amounts of data, but if proper techniques are not used to handle large data, the program can become very slow and unstable. In this article, we will introduce some techniques for working with big data in C.
1. Use dynamic memory allocation
In C, the memory allocation of variables can be static or dynamic. Static memory allocation allocates memory space before the program runs, while dynamic memory allocation allocates memory space as needed while the program is running.
When processing large amounts of data, using dynamic memory allocation can avoid wasting a large amount of memory space. There are two ways to implement dynamic memory allocation: using the new and delete operators, or using an STL container.
The following is a code example that uses the new and delete operators to implement dynamic memory allocation:
int* arr = new int[1000000]; // 动态分配1000000个整型变量的内存空间 // Do something delete[] arr; // 释放内存
When using STL containers, you can use vector or list. The following is a code example of using vector to implement dynamic memory allocation:
#include <vector> std::vector<int> arr(1000000); // 动态分配1000000个整型变量的内存空间 // Do something
2. Using bit operations
Bit operations are a technique for quickly processing big data. Bit operations include operations such as AND, OR, XOR, shift, and negation.
The following is a code example of using bit operations to process big data:
int x = 1000000; int y = 2000000; // 按位与运算 int z1 = x & y; // 按位或运算 int z2 = x | y; // 按位异或运算 int z3 = x ^ y; // 左移动运算 int z4 = x << 2; // 右移动运算 int z5 = x >> 2;
3. Using multi-threading
Multi-threading can allocate tasks to different threads for processing. Thereby speeding up the running speed of the program.
The following is a code example of using multi-threading to process big data:
#include <iostream> #include <thread> #include <vector> void func(int start, int end, std::vector<int>& arr) { for (int i = start; i < end; i++) { // Do something with arr[i] } } int main() { std::vector<int> arr(1000000); // 要处理的数据 int num_threads = 4; int batch_size = arr.size() / num_threads; std::vector<std::thread> threads; for (int i = 0; i < num_threads; i++) { int start = i * batch_size; int end = (i == num_threads - 1) ? arr.size() : (i + 1) * batch_size; threads.push_back(std::thread(func, start, end, std::ref(arr))); } // 等待所有线程完成工作 for (auto& th : threads) { th.join(); } return 0; }
The above are three tips for processing big data in C. Use these tips to make your program run faster and make it more robust.
The above is the detailed content of Big data processing skills in C++. For more information, please follow other related articles on the PHP Chinese website!
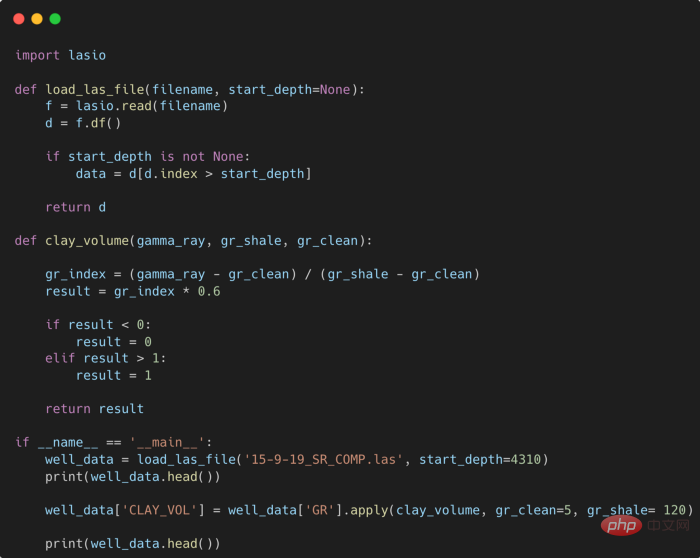
Python 中有许多方法可以帮助我们理解代码的内部工作原理,良好的编程习惯,可以使我们的工作事半功倍!例如,我们最终可能会得到看起来很像下图中的代码。虽然不是最糟糕的,但是,我们需要扩展一些事情,例如:load_las_file 函数中的 f 和 d 代表什么?为什么我们要在 clay 函数中检查结果?这些函数需要什么类型?Floats? DataFrames?在本文中,我们将着重讨论如何通过文档、提示输入和正确的变量名称来提高应用程序/脚本的可读性的五个基本技巧。1. Comments我们可
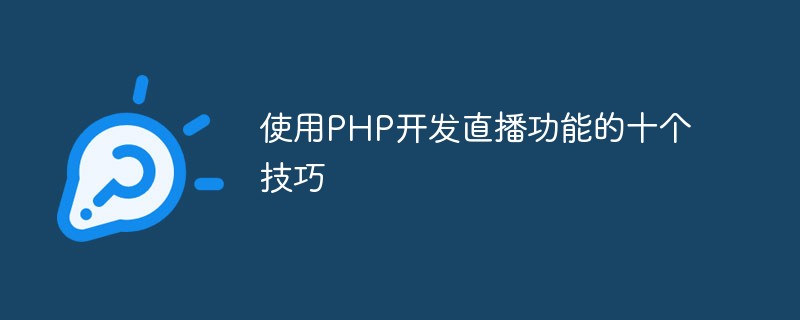
随着直播业务的火爆,越来越多的网站和应用开始加入直播这项功能。PHP作为一种流行的服务器端语言,也可以用来开发高效的直播功能。当然,要实现一个稳定、高效的直播功能需要考虑很多问题。下面列出了使用PHP开发直播功能的十个技巧,帮助你更好地实现直播。选择合适的流媒体服务器PHP开发直播功能,首先需要考虑的就是流媒体服务器的选择。有很多流媒体服务器可以选择,比如常
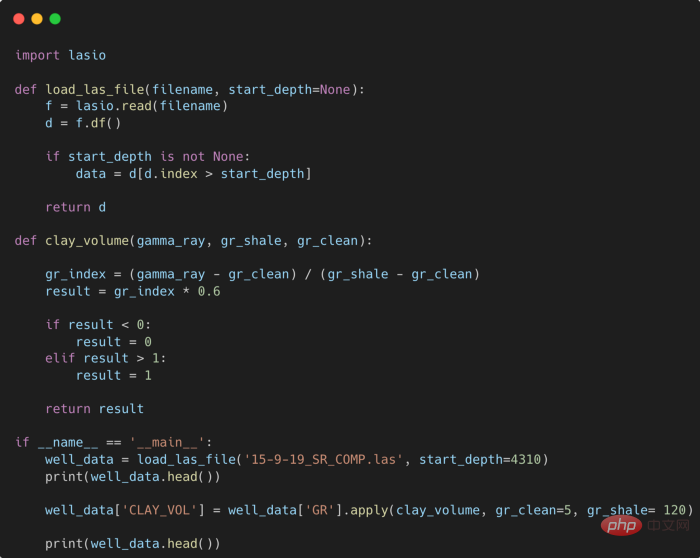
译者 | 赵青窕审校 | 孙淑娟你是否经常回头看看6个月前写的代码,想知道这段代码底是怎么回事?或者从别人手上接手项目,并且不知道从哪里开始?这样的情况对开发者来说是比较常见的。Python中有许多方法可以帮助我们理解代码的内部工作方式,因此当您从头来看代码或者写代码时,应该会更容易地从停止的地方继续下去。在此我给大家举个例子,我们可能会得到如下图所示的代码。这还不是最糟糕的,但有一些事情需要我们去确认,例如:在load_las_file函数中f和d代表什么?为什么我们要在clay函数中检查结果
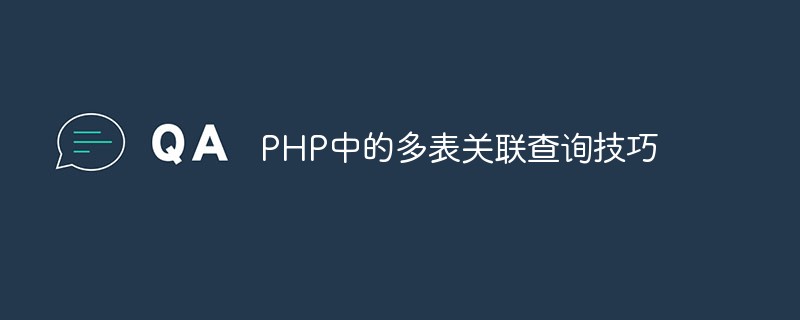
PHP中的多表关联查询技巧关联查询是数据库查询的重要部分,特别是当你需要展示多个相关数据库表内的数据时。在PHP应用程序中,在使用MySQL等数据库时,多表关联查询经常会用到。多表关联的含义是,将一个表中的数据与另一个或多个表中的数据进行比较,在结果中将那些满足要求的行连接起来。在进行多表关联查询时,需要考虑表之间的关系,并使用合适的关联方法。下面介绍几种多
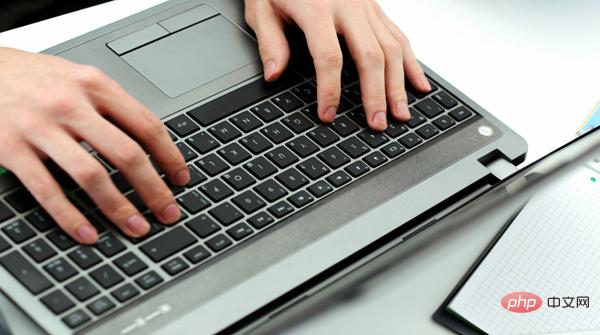
1.简介我们在日常使用Python进行各种数据计算处理任务时,若想要获得明显的计算加速效果,最简单明了的方式就是想办法将默认运行在单个进程上的任务,扩展到使用多进程或多线程的方式执行。而对于我们这些从事数据分析工作的人员而言,以最简单的方式实现等价的加速运算的效果尤为重要,从而避免将时间过多花费在编写程序上。而今天的文章费老师我就来带大家学习如何利用joblib这个非常简单易用的库中的相关功能,来快速实现并行计算加速效果。2.使用joblib进行并行计算作为一个被广泛使用的第三方Python库(
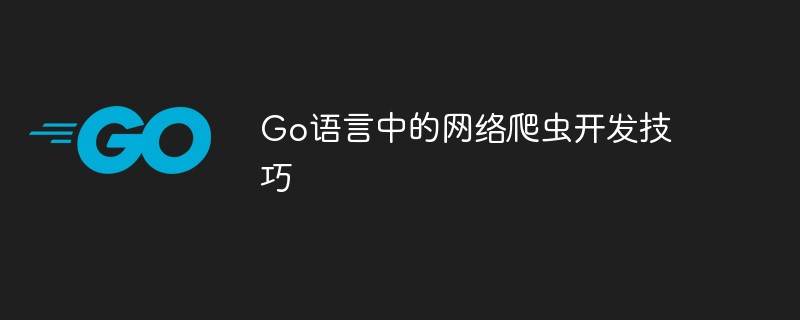
近年来,随着网络信息的急剧增长,网络爬虫技术在互联网行业中扮演着越来越重要的角色。其中,Go语言的出现为网络爬虫的开发带来了诸多优势,如高速度、高并发、低内存占用等。本文将介绍一些Go语言中的网络爬虫开发技巧,帮助开发者更快更好地进行网络爬虫项目开发。一、如何选择合适的HTTP客户端在Go语言中,有多种HTTP请求库可供选择,如net/http、GoRequ
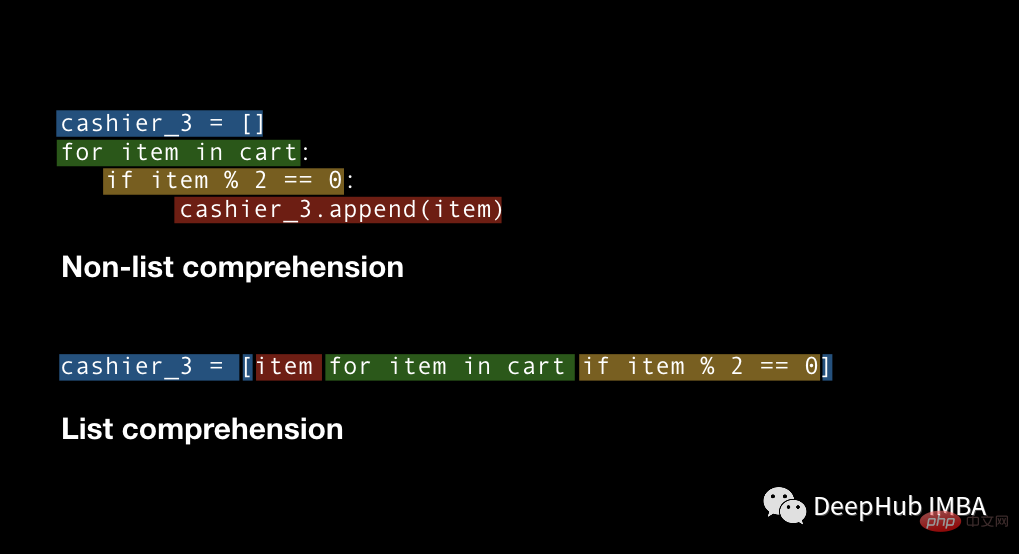
对于数据科学,Python通常被广泛地用于进行数据的处理和转换,它提供了强大的数据结构处理的函数,使数据处理更加灵活,这里说的“灵活性”是什么意思?这意味着在Python中总是有多种方法来实现相同的结果,我们总是有不同的方法并且需要从中选择易于使用、省时并能更好控制的方法。要掌握所有的这些方法是不可能的。所以这里列出了在处理任何类型的数据时应该知道的4个Python技巧。列表推导式ListComprehension是创建列表的一种优雅且最符合python语言的方法。与for循环和if语句相比,列
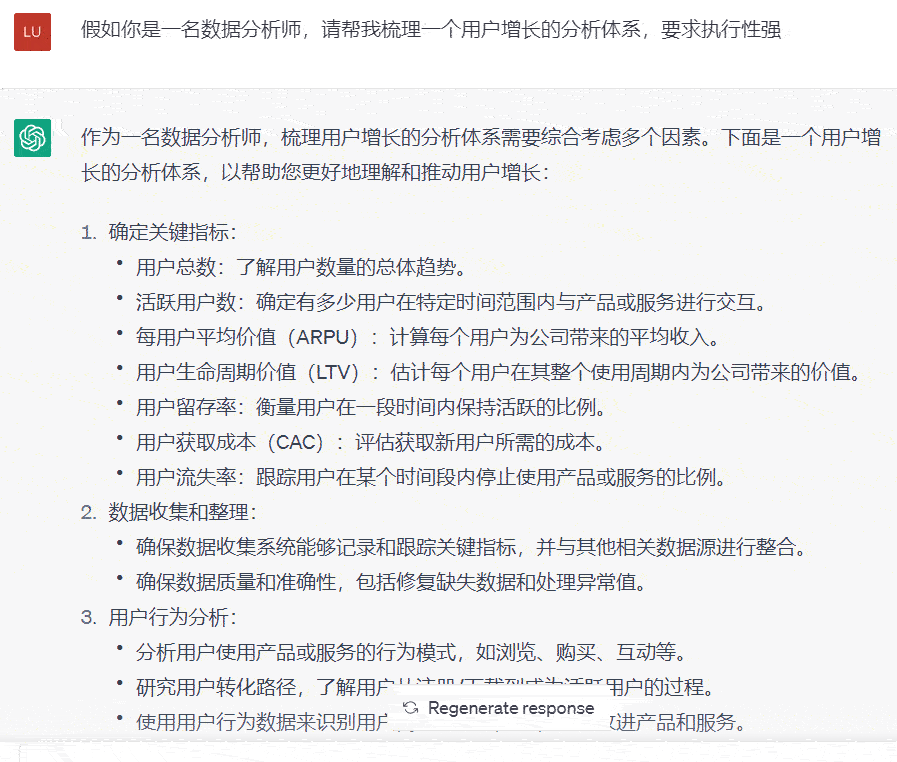
今天给大家分享二个小技巧,第一个可以增加输出的逻辑,让框架逻辑变的更加清晰。先来看看正常情况下GPT的输出,以用户增长分析体系为例:下来我给加一个简单的指令,我们再对比看看效果:是不是效果更好一些?而且逻辑很清晰,当然上面的输出其实不止这些,只是为了举例而已。我们直接让GPT扮演一个资深的Python工程师,帮我写个学习计划吧!提问的时候只需后面加以下这句话即可!let'sthinkstepbystep接下来再看看第二个实用的指令,可以让你的文章更上一个台阶,比如我们让GPT写一个述职报告,这里


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
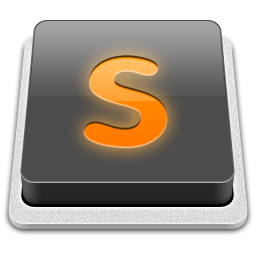
SublimeText3 Mac version
God-level code editing software (SublimeText3)
