Basic network programming knowledge in C++
C, as a high-performance and efficient programming language, is also widely used in the field of network programming. This article will introduce basic network programming knowledge in C, including how to use the socket library for network programming, specific network communication processes, and some common network programming techniques.
1. Use the socket library for network programming
Socket is a network communication interface based on the TCP/IP protocol, which allows programs on different machines to communicate with each other. In C, you can use the socket library for network programming. The socket library provides a set of system calls and library functions that can easily establish network connections, send and receive data in programs.
When using the socket library for network programming, you first need to create a socket object. The method of creating a socket object is usually to call the socket() function, which accepts three parameters: address family, socket type and protocol type. For example:
#include <sys/socket.h> int fd = socket(AF_INET, SOCK_STREAM, 0);
Among them, AF_INET means using the IPv4 address cluster, SOCK_STREAM means using the TCP protocol, and 0 means using the system default protocol.
Next, you need to connect the created socket object to the server. The way to connect to the server is usually to use the connect() function. For example:
#include <arpa/inet.h> struct sockaddr_in server_addr; server_addr.sin_family = AF_INET; server_addr.sin_port = htons(SERVER_PORT); inet_pton(AF_INET, SERVER_ADDR, &server_addr.sin_addr); if (connect(fd, (const struct sockaddr *)&server_addr, sizeof(server_addr)) == -1) { perror("connect error"); exit(1); }
Among them, SERVER_PORT and SERVER_ADDR are the port number and IP address of the server respectively. The function inet_pton() converts the IP address of string type into numeric type. If the connection fails, you can use the perror() function to output error information.
After the connection is successful, you can use the send() function and recv() function to send and receive data. For example:
char buf[BUFSIZ]; ssize_t n; if ((n = recv(fd, buf, BUFSIZ, 0)) == -1) { perror("recv error"); exit(1); }
Among them, BUFSIZ represents the size of the buffer, and the function recv() represents receiving data from the socket object.
2. Specific network communication process
When using the socket library for network programming, common network communication processes include the connection between the client and the server, the sending and receiving of data, and the closing of the connection. . The specific process is as follows:
- Create a socket object: use the socket() function to create a socket object, for example:
int fd = socket(AF_INET, SOCK_STREAM, 0);
- Connect to the server: use the connect() function Connect to the server, for example:
struct sockaddr_in server_addr; server_addr.sin_family = AF_INET; server_addr.sin_port = htons(SERVER_PORT); inet_pton(AF_INET, SERVER_ADDR, &server_addr.sin_addr); if (connect(fd, (const struct sockaddr *)&server_addr, sizeof(server_addr)) == -1) { perror("connect error"); exit(1); }
- Send data: use the send() function to send data, for example:
char *data = "hello, world!"; if (send(fd, data, strlen(data), 0) == -1) { perror("send error"); exit(1); }
- Receive data: use recv( ) function to receive data, for example:
char buf[BUFSIZ]; ssize_t n; if ((n = recv(fd, buf, BUFSIZ, 0)) == -1) { perror("recv error"); exit(1); }
- Close the connection: Use the close() function to close the socket object, for example:
close(fd);
3. Commonly used network programming Tips
When doing network programming, you need to pay attention to some common network programming skills to ensure the reliability and security of network communication.
- Retransmission mechanism: Packet loss may occur during network communication. In order to ensure reliable transmission of data, a data retransmission mechanism can be used.
- Timeout mechanism: When conducting network communication, a reasonable timeout should be set to avoid program blocking caused by too long waiting time.
- Data encryption: Data involving user privacy should be encrypted and transmitted to prevent the data from being stolen and tampered with by criminals.
- Code optimization: When performing network programming, code optimization should be performed to avoid the impact of redundant code on network communication.
In short, the knowledge of network programming in C is very important. Mastering this knowledge can help us easily communicate on the network and implement various network applications.
The above is the detailed content of Basic network programming knowledge in C++. For more information, please follow other related articles on the PHP Chinese website!
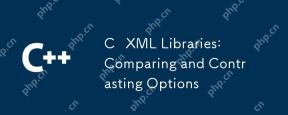
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
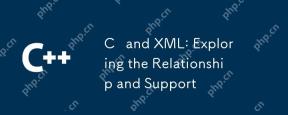
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
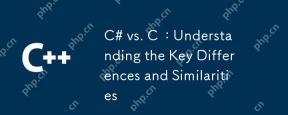
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
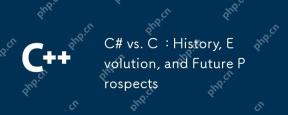
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
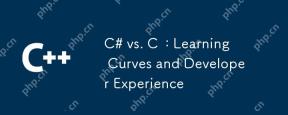
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
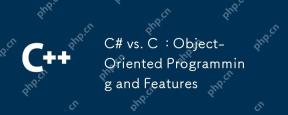
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
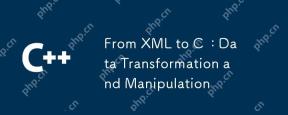
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
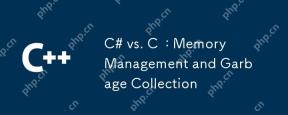
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.