


How to use the __eq__() function in Python to define equality comparison of two objects
How to use the __eq__() function in Python to define equality comparison of two objects
In Python, object comparison is a common operation. When we need to determine whether two objects are equal, we can use the __eq__() function to define and implement it. The __eq__() function is a special function in Python that is used to override the equality comparison operator of objects. Below we will introduce in detail how to use the __eq__() function in Python to define the equality comparison of two objects.
First, let's look at a simple example. Suppose we have a class named Person, which has two attributes: name and age. We hope to be able to determine whether two Person objects are equal, that is, whether their names and ages are the same.
class Person: def __init__(self, name, age): self.name = name self.age = age def __eq__(self, other): if isinstance(other, Person): return self.name == other.name and self.age == other.age return False p1 = Person("Alice", 25) p2 = Person("Bob", 30) p3 = Person("Alice", 25) print(p1 == p2) # 输出False print(p1 == p3) # 输出True
In the above code, we define a Person class and override the __eq__() function in the class. In the __eq__() function, we first use the isinstance() function to determine whether the incoming parameter (other) is an instance of the Person class. If so, we compare whether the names and ages of the two objects are the same. Returns True if they are the same, False otherwise. In this way, we have successfully defined an equality comparison of two Person objects.
It is worth noting that we can also use other methods to determine whether two objects are equal. For example, we can compare only the names of two objects, regardless of age. In this way, we only need to modify the implementation of the __eq__() function. The following is the corresponding code example:
class Person: def __init__(self, name, age): self.name = name self.age = age def __eq__(self, other): if isinstance(other, Person): return self.name == other.name return False p1 = Person("Alice", 25) p2 = Person("Bob", 30) p3 = Person("Alice", 35) print(p1 == p2) # 输出False print(p1 == p3) # 输出True
Through the above code example, we can see that we only rewrote the implementation of the __eq__() function and set it to compare only names. Therefore, the names of p1 and p2 are different, so False is returned; and the names of p1 and p3 are the same, so True is returned.
In short, the __eq__() function in Python is a function used to define and implement equality comparison of objects. By overriding the __eq__() function, we can define the equality comparison method of two objects according to specific needs. Whether all attributes of the object are compared or only some attributes are compared, it can be achieved by appropriately modifying the __eq__() function. This provides us with great flexibility in handling equality comparisons of objects in actual development.
The above is the detailed content of How to use the __eq__() function in Python to define equality comparison of two objects. For more information, please follow other related articles on the PHP Chinese website!
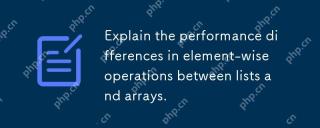
Arraysarebetterforelement-wiseoperationsduetofasteraccessandoptimizedimplementations.1)Arrayshavecontiguousmemoryfordirectaccess,enhancingperformance.2)Listsareflexiblebutslowerduetopotentialdynamicresizing.3)Forlargedatasets,arrays,especiallywithlib
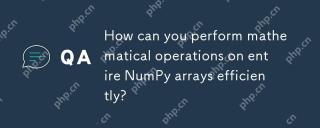
Mathematical operations of the entire array in NumPy can be efficiently implemented through vectorized operations. 1) Use simple operators such as addition (arr 2) to perform operations on arrays. 2) NumPy uses the underlying C language library, which improves the computing speed. 3) You can perform complex operations such as multiplication, division, and exponents. 4) Pay attention to broadcast operations to ensure that the array shape is compatible. 5) Using NumPy functions such as np.sum() can significantly improve performance.
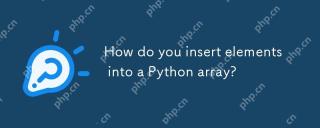
In Python, there are two main methods for inserting elements into a list: 1) Using the insert(index, value) method, you can insert elements at the specified index, but inserting at the beginning of a large list is inefficient; 2) Using the append(value) method, add elements at the end of the list, which is highly efficient. For large lists, it is recommended to use append() or consider using deque or NumPy arrays to optimize performance.
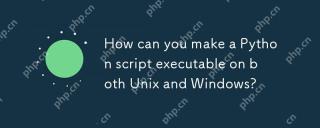
TomakeaPythonscriptexecutableonbothUnixandWindows:1)Addashebangline(#!/usr/bin/envpython3)andusechmod xtomakeitexecutableonUnix.2)OnWindows,ensurePythonisinstalledandassociatedwith.pyfiles,oruseabatchfile(run.bat)torunthescript.
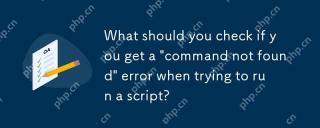
When encountering a "commandnotfound" error, the following points should be checked: 1. Confirm that the script exists and the path is correct; 2. Check file permissions and use chmod to add execution permissions if necessary; 3. Make sure the script interpreter is installed and in PATH; 4. Verify that the shebang line at the beginning of the script is correct. Doing so can effectively solve the script operation problem and ensure the coding process is smooth.
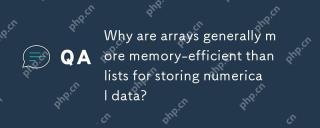
Arraysaregenerallymorememory-efficientthanlistsforstoringnumericaldataduetotheirfixed-sizenatureanddirectmemoryaccess.1)Arraysstoreelementsinacontiguousblock,reducingoverheadfrompointersormetadata.2)Lists,oftenimplementedasdynamicarraysorlinkedstruct
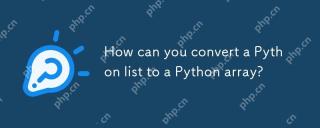
ToconvertaPythonlisttoanarray,usethearraymodule:1)Importthearraymodule,2)Createalist,3)Usearray(typecode,list)toconvertit,specifyingthetypecodelike'i'forintegers.Thisconversionoptimizesmemoryusageforhomogeneousdata,enhancingperformanceinnumericalcomp
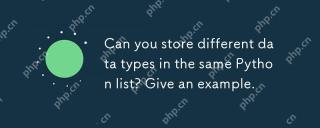
Python lists can store different types of data. The example list contains integers, strings, floating point numbers, booleans, nested lists, and dictionaries. List flexibility is valuable in data processing and prototyping, but it needs to be used with caution to ensure the readability and maintainability of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
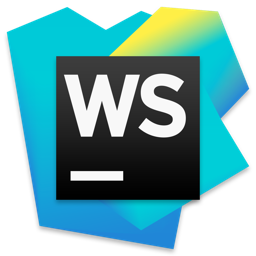
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
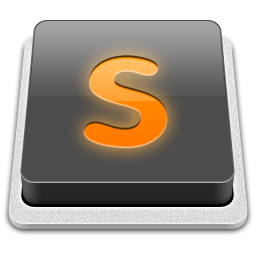
SublimeText3 Mac version
God-level code editing software (SublimeText3)
