Solve golang error: undefined package name 'x', solution
Solution to golang error: undefined package name 'x', solution
In the process of using Go language development, we sometimes encounter some errors, one of which is common The error is "undefined package name 'x'". When we introduce third-party packages or custom packages into the code, this error will occur if the package name is not imported correctly. This article explains how to resolve this error, along with code examples.
First, we need to confirm whether the corresponding package is imported correctly. In the statement that introduces the package, you should ensure that the package name is correct, including not only the path of the package, but also the name of the package.
For example, suppose we want to use the third-party library "gin" to build a Web application. Our code may look like the following:
package main import "gin" func main() { // code here }
However, this code will cause the error "undefined package name 'gin'". This is because we did not import the "gin" package correctly. The correct import method should be:
package main import "github.com/gin-gonic/gin" func main() { // code here }
Note that "gin" is a third-party library, so you need to specify the complete package path "github.com/gin- gonic/gin".
Similarly, if we use a custom package in the code, we also need to ensure that it is imported correctly. The following is a code example of a custom package:
package main import "myapp/mylib" func main() { // code here }
If we place the custom package in the same directory as the main program and name it "mylib", then we need to import the custom package through a relative path package, the code example is as follows:
package main import "./mylib" func main() { // code here }
Note that when importing a custom package, "./" represents the current directory.
If you still encounter the "undefined package name 'x'" error, we can try to execute the go mod tidy
or go get
command in the terminal to download the corresponding package. These commands automatically download and install the required packages, updating the go.mod and go.sum files.
In addition, we can also try clearing the cache. Execute the go clean -modcache
command to clear the module cache so that the corresponding package can be re-downloaded.
Finally, if none of the above methods can solve the problem, it is possible that the package was not downloaded correctly or the version of the package is incompatible. We can try to delete the go.mod and go.sum files and re-execute the go mod init
and go get
commands to regenerate and download the package.
To summarize, the methods to solve "golang error: undefined package name 'x'" are as follows:
- Confirm the correctness of the package name import, including the path and name;
- Execute the
go mod tidy
orgo get
command to download the corresponding package; - Clear the cache and execute
go clean -modcache
Command; - Delete the go.mod and go.sum files, and re-execute the
go mod init
andgo get
commands.
I hope the solution in this article can help developers who encounter the "golang error: undefined package name 'x'" problem. I wish you all good luck in solving problems during the development of Go language!
The above is the detailed content of Solve golang error: undefined package name 'x', solution. For more information, please follow other related articles on the PHP Chinese website!
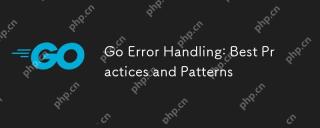
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
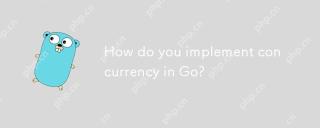
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
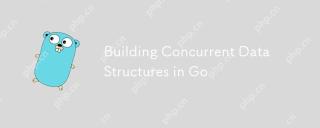
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
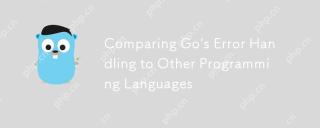
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand
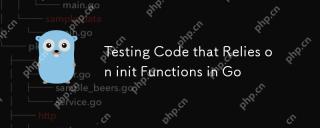
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
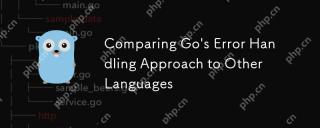
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
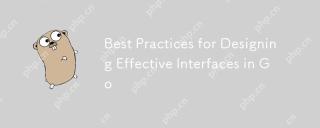
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
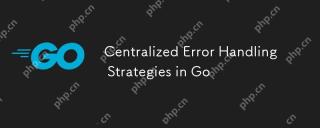
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
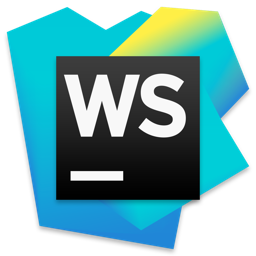
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
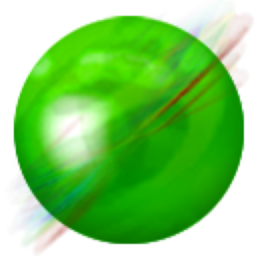
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
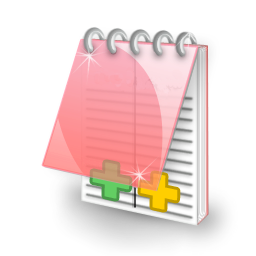
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
