Solve the problem of PHP error: Unable to parse class constants
Solution to PHP error: Unable to parse class constants
In PHP development, we often use class constants to store global configurations or commonly used values. However, sometimes when calling a class constant, there may be a problem that the constant cannot be parsed, causing the program to report an error. This article will introduce several common causes and solutions to help developers quickly solve this problem.
First, let’s understand what a class constant is. Class constants are unchangeable values defined in a class. Unlike ordinary class properties, class constants cannot be modified, nor can they be referenced using the $this keyword in class instance methods. Class constants are usually used to store global configuration information or commonly used values related to the class, such as database connection information, API keys, etc.
The following is a sample code using class constants:
class Config { const DB_HOST = 'localhost'; const DB_USERNAME = 'admin'; const DB_PASSWORD = 'password'; const DB_NAME = 'database'; } $conn = mysqli_connect(Config::DB_HOST, Config::DB_USERNAME, Config::DB_PASSWORD, Config::DB_NAME);
In the above code, we define a Config class and four constants in it to store database connection related information . By calling the constants of the Config class, we can easily connect to the database.
However, in actual development, sometimes we may encounter the problem of being unable to resolve class constants, which may be caused by the following reasons:
- Constant is not defined
Before using class constants, you must first ensure that the constants have been defined. If a constant is not defined or is defined in the wrong location, the constant cannot be resolved. The solution is to make sure that the class constant is correctly defined before using it.
- Namespace conflict
If a namespace is used in your code and a constant with the same name is defined in the namespace, a constant parsing error will occur. The solution is to use a fully qualified name to specify the class to which the constant belongs. For example, if the namespace MyApp
is used, you can use MyAppConfig::DB_HOST
to call class constants to avoid conflicts with constants in other namespaces.
- Constant prefix error
When using class constants, you need to use the form class name::constant name
to call. If you forget the class name prefix when calling, the constant will not be resolved. The solution is to always add the correct class name prefix when calling class constants.
- The class is not loaded
If the class file is not loaded correctly, the class constants cannot be parsed. The solution is to ensure that the class file has been loaded correctly. You can use require
or autoload
to load class files.
To sum up, the problem of being unable to resolve class constants may be caused by undefined constants, namespace conflicts, constant prefix errors, or classes not being loaded. When developers encounter this problem, they can investigate it one by one based on the specific situation.
When solving this problem, you can use the following methods to troubleshoot:
- Check the location of the constant definition to ensure that the constant is correctly defined.
- If a namespace is used, use fully qualified names to call class constants to avoid namespace conflicts.
- Always add the correct class name prefix when calling class constants.
- Make sure the class file has been loaded correctly.
In short, we can solve the problem of PHP not being able to resolve class constants by checking where the constants are defined and avoiding namespace conflicts while using the correct class name prefix and ensuring that the class file is loaded. Avoid errors.
I hope this article will help developers solve the problem of unresolved class constants in PHP errors.
The above is the detailed content of Solve the problem of PHP error: Unable to parse class constants. For more information, please follow other related articles on the PHP Chinese website!
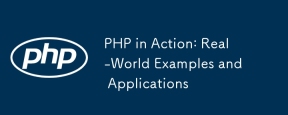
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
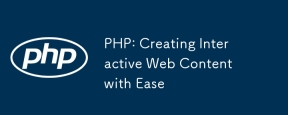
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
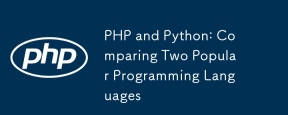
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
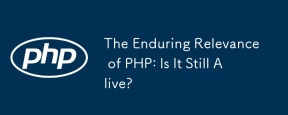
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
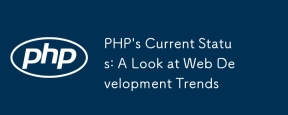
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
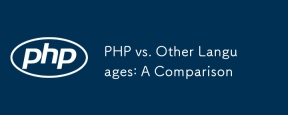
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
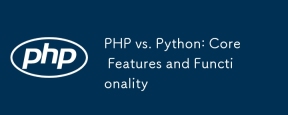
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
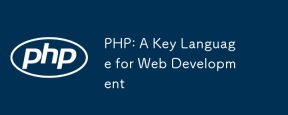
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
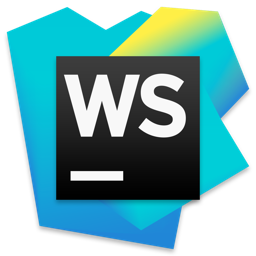
WebStorm Mac version
Useful JavaScript development tools
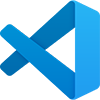
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool