How to Fix: Java Concurrency Error: Thread Safety Issue
How to solve: Java Concurrency Error: Thread Safety Issue
Introduction:
When developing Java applications, we often encounter thread safety issues. Because multiple threads access shared resources simultaneously, data inconsistency and unpredictable results may result. This article will explore common thread safety issues in Java concurrent programming and provide solutions and sample code.
1. The difference between thread safety and non-thread safety:
In multi-thread programming, thread safety means that when multiple threads operate on shared data, inconsistent results will not occur. Non-thread safety means that operations on shared data by multiple threads may lead to inconsistent results.
2. Common thread safety issues:
-
Race Condition:
When multiple threads access and operate shared data concurrently, Due to the uncertainty of the execution sequence, the program may produce erroneous results. For example, if two threads read and increment the value of a variable at the same time, without synchronization control, the increment operation may be overwritten, and the final result does not meet expectations.public class RaceConditionExample { private int count; public void increment() { count++; } public int getCount() { return count; } }
Solution:
-
Use synchronized keyword or ReentrantLock for synchronization control:
public class RaceConditionExample { private int count; private Object lock = new Object(); public synchronized void increment() { count++; } public int getCount() { synchronized (lock) { return count; } } }
- Deadlock:
A deadlock may occur when multiple threads wait for each other to release resources. For example, if thread A owns lock A and is waiting for lock B, and thread B owns lock B and is waiting for lock A, neither thread will be able to continue executing.
Solution:
- Use an algorithm to avoid deadlock, such as applying for locks in order.
- Set the timeout. When the lock cannot be acquired within a period of time, release the currently acquired lock.
- Use the tryLock() method of the Lock object to try to acquire the lock, and handle it accordingly based on success or failure.
- Thread-unsafe collection class usage:
In Java, there are some collection classes (such as ArrayList, HashMap) that are not thread-safe. When multiple threads access and modify these collections at the same time, problems such as array out-of-bounds and data overwriting may occur.
Solution:
- Use thread-safe collection classes, such as Vector, Hashtable, ConcurrentHashMap, etc.
- Use the synchronizedList() and synchronizedMap() methods of the Collections tool class to synchronize the collection.
- Visibility Problem:
When a thread modifies shared data, other threads may not be able to see the modification immediately, leading to incorrect results. This is because each thread has its own working memory, and modifications to shared variables are not immediately synchronized to main memory.
Solution:
- Use the volatile keyword to modify shared variables to ensure visibility.
- Use the synchronized keyword or Lock object for synchronization operations to ensure data synchronization and visibility.
3. Summary:
When developing Java applications, we must pay attention to thread safety issues to avoid program errors in a multi-threaded environment. Thread safety issues can be effectively solved by using synchronization control, avoiding deadlocks, using thread-safe collection classes, and ensuring visibility.
Reference materials:
-"Java Concurrency in Practice"
-"Java Concurrency Programming in Practice"
The above are some solutions to Java concurrency errors: thread safety issues Suggestions and sample code. Hope this helps!
The above is the detailed content of How to Fix: Java Concurrency Error: Thread Safety Issue. For more information, please follow other related articles on the PHP Chinese website!
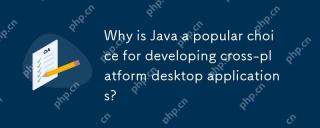
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
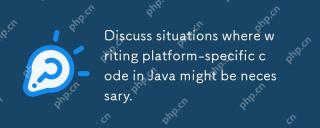
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
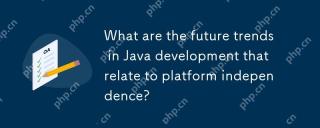
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.
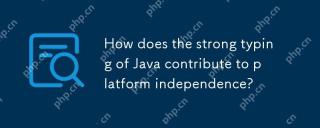
Java's strong typed system ensures platform independence through type safety, unified type conversion and polymorphism. 1) Type safety performs type checking at compile time to avoid runtime errors; 2) Unified type conversion rules are consistent across all platforms; 3) Polymorphism and interface mechanisms make the code behave consistently on different platforms.
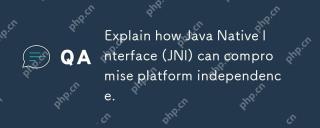
JNI will destroy Java's platform independence. 1) JNI requires local libraries for a specific platform, 2) local code needs to be compiled and linked on the target platform, 3) Different versions of the operating system or JVM may require different local library versions, 4) local code may introduce security vulnerabilities or cause program crashes.
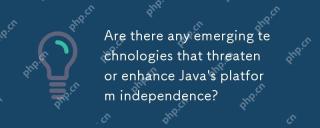
Emerging technologies pose both threats and enhancements to Java's platform independence. 1) Cloud computing and containerization technologies such as Docker enhance Java's platform independence, but need to be optimized to adapt to different cloud environments. 2) WebAssembly compiles Java code through GraalVM, extending its platform independence, but it needs to compete with other languages for performance.
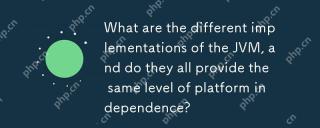
Different JVM implementations can provide platform independence, but their performance is slightly different. 1. OracleHotSpot and OpenJDKJVM perform similarly in platform independence, but OpenJDK may require additional configuration. 2. IBMJ9JVM performs optimization on specific operating systems. 3. GraalVM supports multiple languages and requires additional configuration. 4. AzulZingJVM requires specific platform adjustments.
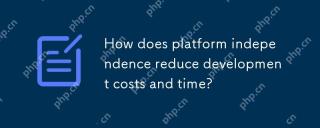
Platform independence reduces development costs and shortens development time by running the same set of code on multiple operating systems. Specifically, it is manifested as: 1. Reduce development time, only one set of code is required; 2. Reduce maintenance costs and unify the testing process; 3. Quick iteration and team collaboration to simplify the deployment process.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
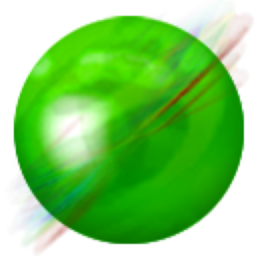
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
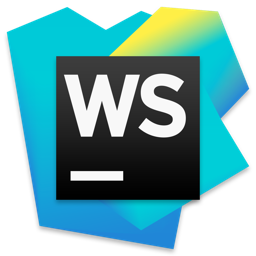
WebStorm Mac version
Useful JavaScript development tools
