How to use Python to template match images
Introduction:
Template matching is a technique used to find specific patterns or objects in images. It is widely used in the fields of computer vision and image processing. Python provides many powerful image processing libraries, allowing us to easily perform template matching tasks. This article will introduce how to use Python for image template matching, with code examples.
1. Preparation:
Before using Python for template matching, we need to install the following libraries: OpenCV, NumPy and Matplotlib. They can be installed by using pip or conda. Once the installation is complete, we can start writing code.
2. Import libraries:
First, we need to import the required libraries. The following is the corresponding code example:
import cv2 import numpy as np import matplotlib.pyplot as plt
3. Load images and templates:
Before template matching, we need to load the images and templates to be matched. The following is the corresponding code example:
# 加载图像和模板 image = cv2.imread('image.jpg') template = cv2.imread('template.jpg')
4. Implement template matching:
Next, we will use OpenCV’s matchTemplate() function to implement template matching. The following is the corresponding code example:
# 将输入图像转换为灰度 gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) gray_template = cv2.cvtColor(template, cv2.COLOR_BGR2GRAY) # 应用模板匹配 result = cv2.matchTemplate(gray_image, gray_template, cv2.TM_CCOEFF_NORMED)
5. Find the best matching result:
Template matching returns a floating point matrix, indicating the matching degree at each pixel position. We need to analyze this matrix to find the location of the best matching result. The following is the corresponding code example:
# 定义一个阈值,用于筛选匹配结果 threshold = 0.8 # 使用np.where()函数找到满足阈值条件的位置 location = np.where(result >= threshold) # 在原图像中绘制边界框 w, h = gray_template.shape[::-1] for pt in zip(*locations[::-1]): cv2.rectangle(image, pt, (pt[0]+w, pt[1]+h), (0, 255, 0), 2)
6. Display the results:
Finally, we can use the Matplotlib library to display the results. The following is the corresponding code example:
# 显示匹配结果 plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB)) plt.title('Template Matching Result') plt.axis('off') plt.show()
Conclusion:
By using Python and related image processing libraries, we can easily implement image template matching. This article explains how to load images and templates, implement template matching, find the best match, and display the results. With these basic steps, we can perform more complex image processing tasks such as target detection and object recognition.
The above is an introduction to how to use Python to template match images. Hope this article can be helpful to you!
The above is the detailed content of How to template match images using Python. For more information, please follow other related articles on the PHP Chinese website!
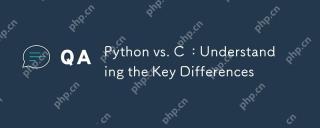
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
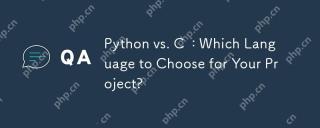
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
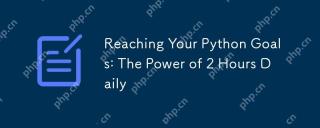
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
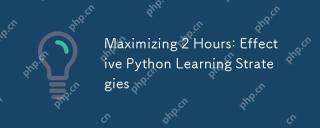
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
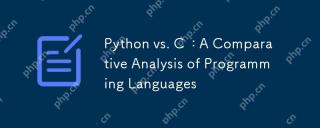
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
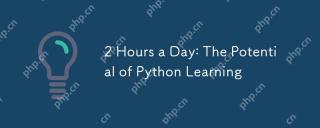
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
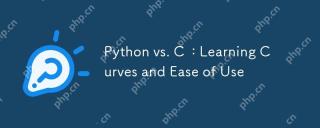
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
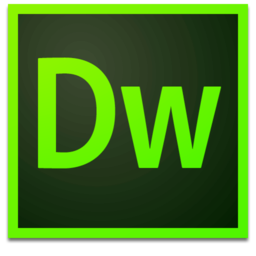
Dreamweaver Mac version
Visual web development tools
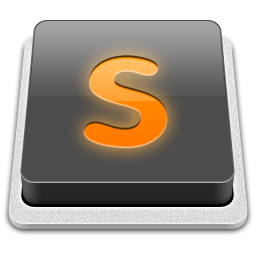
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
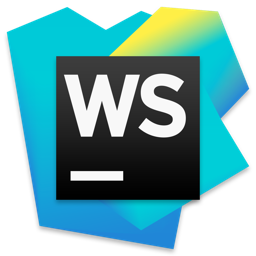
WebStorm Mac version
Useful JavaScript development tools