


How to use Python to adjust the brightness and contrast of pictures
Introduction:
In image processing, adjusting brightness and contrast is one of the common and important operations. Python provides a wealth of image processing libraries, such as PIL, OpenCV, etc., which can easily implement these two operations. This article will introduce how to use Python code to adjust the brightness and contrast of images and give corresponding code examples.
Part 1: Adjust image brightness
The adjustment of image brightness can be achieved by changing the RGB value of each pixel. The specific method is to add a fixed increment to the RGB value of each pixel. The following is a sample code that uses the PIL library to adjust the brightness of an image:
from PIL import ImageEnhance # 打开图像 image = Image.open('image.jpg') # 创建Enhancer对象 enhancer = ImageEnhance.Brightness(image) # 调整亮度为原来的2倍 bright_image = enhancer.enhance(2) # 显示图像 bright_image.show() # 保存图像 bright_image.save('bright_image.jpg')
In the above code, first open an image through the Image.open() function, and then use the ImageEnhance.Brightness() function to create an Enhancer object. Then use the enhance() method of the Enhancer object to adjust the brightness of the image. The parameter represents the multiple of the brightness. Finally, call the show() method to display the adjusted image, or you can use the save() method to save the image.
Part 2: Adjusting Image Contrast
The adjustment of image contrast is achieved by changing the relative brightness of each pixel. The specific method is to multiply the RGB value of each pixel by a fixed increment. The following is a sample code that uses the OpenCV library to adjust the contrast of an image:
import cv2 # 读取图像 image = cv2.imread('image.jpg') # 提取亮度通道 hsv_image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV) v_channel = hsv_image[:,:,2] # 调整亮度为原来的2倍 contrast_image = cv2.addWeighted(v_channel, 2, np.zeros(v_channel.shape, v_channel.dtype), 0, 0) # 将亮度通道替换回原图像 hsv_image[:,:,2] = contrast_image contrast_image = cv2.cvtColor(hsv_image, cv2.COLOR_HSV2BGR) # 显示图像 cv2.imshow('contrast_image', contrast_image) cv2.waitKey(0) # 保存图像 cv2.imwrite('contrast_image.jpg', contrast_image)
In the above code, an image is first read using the cv2.imread() function, and then the image is converted using the cv2.cvtColor() function. Convert from BGR color space to HSV color space because HSV color space is more sensitive to changes in brightness. Then by extracting the brightness channel hsv_image[:,:,2], a two-dimensional array v_channel representing brightness is obtained.
Then use the cv2.addWeighted() function to linearly combine the brightness channels to adjust the contrast, where the first parameter represents the input image, and the second parameter represents the multiple of brightness, here set to 2. Finally, the adjusted brightness channel is replaced back to the original image, and the image is converted from the HSV color space back to the BGR color space through the cv2.cvtColor() function.
Finally, the adjusted image is displayed through the cv2.imshow() function, cv2.waitKey(0) is used to wait for the key, and the cv2.imwrite() function is used to save the image.
Conclusion:
Through the above code examples, we can see that using Python to adjust the brightness and contrast of an image is very simple and can be achieved with just a few lines of code. Through different parameter settings, different degrees of brightness and contrast adjustment can be achieved to meet different needs. In practical applications, appropriate adjustment methods and parameters can be selected according to specific situations to achieve the best results. I hope this article will be helpful to readers in their learning and practice in image processing.
The above is the detailed content of How to adjust the brightness and contrast of an image using Python. For more information, please follow other related articles on the PHP Chinese website!
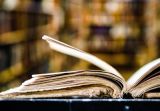
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
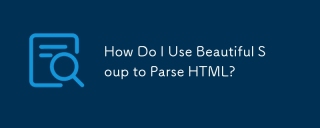
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
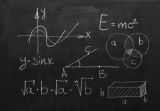
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
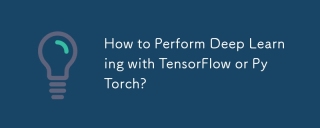
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
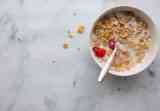
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
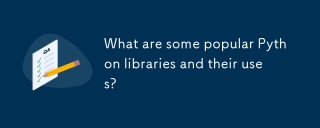
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
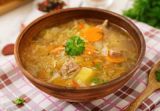
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
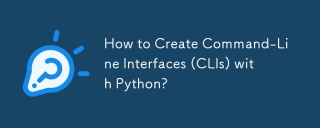
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
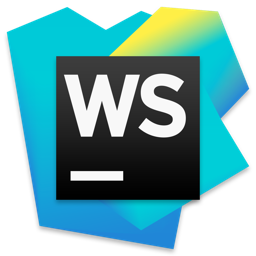
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
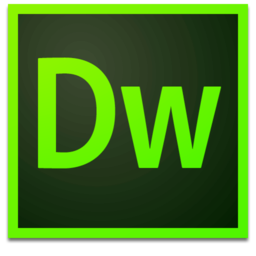
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
