


Essential for Java engineers: Performance monitoring and tuning strategies for Baidu AI interface docking
Must-have for Java engineers: Performance monitoring and tuning strategies for Baidu AI interface docking
Abstract: With the rapid development of artificial intelligence technology, Baidu AI interface provides Rich functions and services, such as voice recognition, face recognition, etc. At the same time, in order to ensure the performance and stability of the system, performance monitoring and tuning are required during docking. This article will introduce the performance monitoring and tuning strategies of Baidu AI interface and provide corresponding Java code examples.
- Introduction
Baidu AI interface is a set of artificial intelligence services provided by Baidu with high accuracy and reliability. During the interface docking process, in order to ensure the performance and stability of the system, performance monitoring and tuning are required. - Performance Monitoring
Performance monitoring refers to the process of evaluating system performance by monitoring interface calls, response time and other indicators. In Baidu AI interface docking, we can obtain relevant performance indicators through the interface provided by Baidu, and conduct real-time monitoring and early warning.
2.1 Monitoring the number of requests
During the operation of the system, we can understand the usage of the system by recording the number of requests for the interface. You can use the getUsage
method provided by Baidu AI interface to obtain the number of requests. The code example is as follows:
import com.baidu.aip.util.HttpUtil; public class BaiduAIInterface { private static final String API_KEY = "YOUR_API_KEY"; private static final String SECRET_KEY = "YOUR_SECRET_KEY"; public static void main(String[] args) { String result = HttpUtil.get(String.format("https://aip.baidubce.com/rpc/2.0/usage?access_token=%s", getAccessToken())); System.out.println(result); } private static String getAccessToken() { // 实现获取AccessToken的逻辑 } }
2.2 Response time monitoring
In addition to the number of requests, we also need to monitor the response time of the interface. By measuring the processing time of each request, we can understand the load and response performance of the system. You can use the getAITraffic
method provided by Baidu AI interface to obtain the response time. The code example is as follows:
import com.baidu.aip.util.HttpUtil; public class BaiduAIInterface { private static final String API_KEY = "YOUR_API_KEY"; private static final String SECRET_KEY = "YOUR_SECRET_KEY"; public static void main(String[] args) { String result = HttpUtil.get(String.format("https://aip.baidubce.com/rpc/2.0/aipTraffic?access_token=%s", getAccessToken())); System.out.println(result); } private static String getAccessToken() { // 实现获取AccessToken的逻辑 } }
- Performance Tuning
Performance tuning refers to optimizing the resource utilization of the system , algorithm design and other means to improve system performance. In Baidu AI interface docking, we can perform performance tuning from the following aspects.
3.1 Concurrency Tuning
In high concurrency scenarios, in order to improve the system's concurrent processing capabilities, you can use thread pools or thread reuse to process requests. It can be implemented using the Java ThreadPoolExecutor
class. The code example is as follows:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class BaiduAIInterface { private static final int THREAD_POOL_SIZE = 10; // 其他代码省略 public static void main(String[] args) { ExecutorService executorService = Executors.newFixedThreadPool(THREAD_POOL_SIZE); // 提交任务到线程池 executorService.execute(new Runnable() { @Override public void run() { // 实现接口调用逻辑 } }); } }
3.2 Cache Tuning
During Baidu AI interface docking, cache can be used to reduce the number of calls to the docking interface and improve system performance. You can use Java caching libraries, such as Ehcache or Caffeine, to cache interface results.
3.3 Asynchronous Tuning
For long-time interface calls, Java’s asynchronous processing mechanism can be used to improve the system’s concurrent processing capabilities. You can use Java8's CompletableFuture class to implement asynchronous calls. The code example is as follows:
import java.util.concurrent.CompletableFuture; public class BaiduAIInterface { // 其他代码省略 public static void main(String[] args) { CompletableFuture.supplyAsync(BaiduAIInterface::callAIInterface) .thenAccept(result -> { // 处理接口返回结果 }); } private static String callAIInterface() { // 实现接口调用逻辑,并返回结果 } }
- Conclusion
When docking Baidu AI interface, performance monitoring and tuning are very necessary. Through performance monitoring, we can understand system usage and response performance; through performance tuning, we can improve the system's concurrent processing capabilities and response speed. This article introduces the performance monitoring and tuning strategies of Baidu AI interface and provides corresponding Java code examples. I hope it will be helpful to Java engineers in optimizing the performance of Baidu AI interface docking.
The above is the detailed content of Essential for Java engineers: Performance monitoring and tuning strategies for Baidu AI interface docking. For more information, please follow other related articles on the PHP Chinese website!
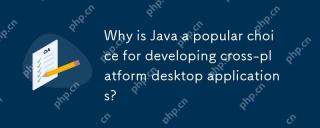
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
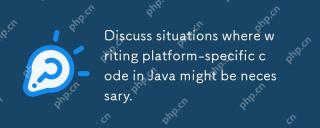
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
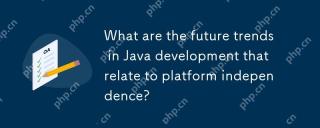
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.
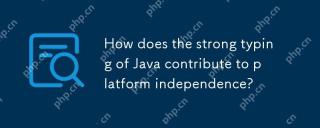
Java's strong typed system ensures platform independence through type safety, unified type conversion and polymorphism. 1) Type safety performs type checking at compile time to avoid runtime errors; 2) Unified type conversion rules are consistent across all platforms; 3) Polymorphism and interface mechanisms make the code behave consistently on different platforms.
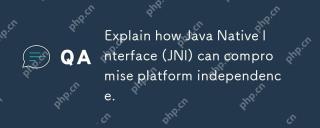
JNI will destroy Java's platform independence. 1) JNI requires local libraries for a specific platform, 2) local code needs to be compiled and linked on the target platform, 3) Different versions of the operating system or JVM may require different local library versions, 4) local code may introduce security vulnerabilities or cause program crashes.
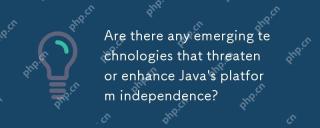
Emerging technologies pose both threats and enhancements to Java's platform independence. 1) Cloud computing and containerization technologies such as Docker enhance Java's platform independence, but need to be optimized to adapt to different cloud environments. 2) WebAssembly compiles Java code through GraalVM, extending its platform independence, but it needs to compete with other languages for performance.
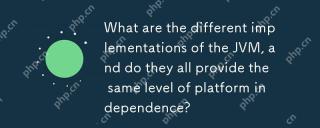
Different JVM implementations can provide platform independence, but their performance is slightly different. 1. OracleHotSpot and OpenJDKJVM perform similarly in platform independence, but OpenJDK may require additional configuration. 2. IBMJ9JVM performs optimization on specific operating systems. 3. GraalVM supports multiple languages and requires additional configuration. 4. AzulZingJVM requires specific platform adjustments.
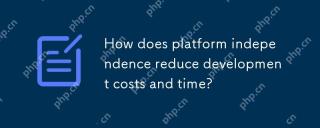
Platform independence reduces development costs and shortens development time by running the same set of code on multiple operating systems. Specifically, it is manifested as: 1. Reduce development time, only one set of code is required; 2. Reduce maintenance costs and unify the testing process; 3. Quick iteration and team collaboration to simplify the deployment process.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
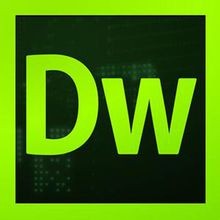
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
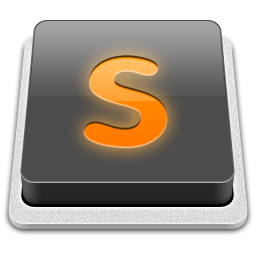
SublimeText3 Mac version
God-level code editing software (SublimeText3)
