Building RESTful APIs with Laravel: Modernizing backend development
Building RESTful APIs with Laravel: Modernizing back-end development
Introduction:
In modern web development, building RESTful APIs has become a Mainstream way. RESTful APIs provide a flexible, extensible way to interact with front-end applications, mobile applications, or other third-party services.
Laravel is an excellent PHP framework that not only provides simple and efficient syntax, but also has many powerful built-in functions. In this article, we will explore how to build a RESTful API using Laravel and demonstrate its usage through examples.
- Installing Laravel
First, we need to install Laravel in the local environment. You can use Composer to run the following command to install the latest version of Laravel:
composer global require laravel/installer
- Create Laravel Project
After the installation is complete, you can create a new Laravel project by running the following command:
laravel new api-project
This will create a new project named api-project
in the current directory.
- Create API routing
Laravel uses routing (Route) to handle different URL requests. Next, we will create a set of API routes to handle various HTTP request methods (GET, POST, PUT, DELETE).
In the routes/api.php
file, add the following code:
<?php use IlluminateHttpRequest; use IlluminateSupportFacadesRoute; Route::get('/users', 'UserController@index'); Route::post('/users', 'UserController@store'); Route::get('/users/{id}', 'UserController@show'); Route::put('/users/{id}', 'UserController@update'); Route::delete('/users/{id}', 'UserController@destroy');
The above code defines a set of API routes for operating users, including obtaining users List, create new users, get specific user information, update user information and delete users.
- Create Controller
In Laravel, the controller (Controller) is responsible for handling the specific logic of routing. We need to create a UserController to handle user-related API requests.
Create a UserController using the following command:
php artisan make:controller UserController
This will create a userController named UserController
in the app/Http/Controllers
directory controller.
Open the UserController.php
file and add the following code:
<?php namespace AppHttpControllers; use IlluminateHttpRequest; use AppUser; class UserController extends Controller { public function index() { return User::all(); } public function store(Request $request) { return User::create($request->all()); } public function show($id) { return User::findOrFail($id); } public function update(Request $request, $id) { $user = User::findOrFail($id); $user->update($request->all()); return $user; } public function destroy($id) { $user = User::findOrFail($id); $user->delete(); return response()->json(null, 204); } }
In the above code, we use the Eloquent model to process user data through database operations. To simplify the example, we use Laravel's default User model. In actual projects, you may need to customize the model.
- Perform Migrations
Before using the database, we need to perform migrations (Migrations) first. This will create the corresponding database table.
Run the following command to perform the migration operation:
php artisan migrate
- Start the server
After everything is ready, you can start Laravel's built-in development server with the following command:
php artisan serve
This will start a server locally, listening on the http://127.0.0.1:8000
address.
- Testing the API
Now we can use Postman or other API testing tools to test the API.
- Get user list: send GET request to
http://127.0.0.1:8000/api/users
- Create new user: send POST request to
http://127.0.0.1:8000/api/users
, and attach the user information that needs to be created - Obtain specific user information: Send a GET request to
http ://127.0.0.1:8000/api/users/{id}
, replace{id}
with the actual user ID - Update user information: send a PUT request to
http://127.0.0.1:8000/api/users/{id}
, and attach the user information that needs to be updated - Delete user: Send a DELETE request to
http:/ /127.0.0.1:8000/api/users/{id}
, replace{id}
with the actual user ID
Through the above steps, we successfully created Developed a RESTful API built using Laravel and implemented modern back-end development. The Laravel framework provides a range of powerful tools and features that make building and maintaining APIs easier and more efficient.
Conclusion:
Laravel is a powerful and easy-to-use PHP framework that is ideal for building RESTful APIs. This article introduces how to use Laravel to create API routes, controllers, and database migrations, and demonstrates how to test the API through examples. I hope this article can help you better understand the Laravel framework and apply it to RESTful API development in actual projects.
The above is the detailed content of Building RESTful APIs with Laravel: Modernizing backend development. For more information, please follow other related articles on the PHP Chinese website!

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.

The Laravel development project was chosen because of its flexibility and power to suit the needs of different sizes and complexities. Laravel provides routing system, EloquentORM, Artisan command line and other functions, supporting the development of from simple blogs to complex enterprise-level systems.

The comparison between Laravel and Python in the development environment and ecosystem is as follows: 1. The development environment of Laravel is simple, only PHP and Composer are required. It provides a rich range of extension packages such as LaravelForge, but the extension package maintenance may not be timely. 2. The development environment of Python is also simple, only Python and pip are required. The ecosystem is huge and covers multiple fields, but version and dependency management may be complex.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.

Laravel's popularity includes its simplified development process, providing a pleasant development environment, and rich features. 1) It absorbs the design philosophy of RubyonRails, combining the flexibility of PHP. 2) Provide tools such as EloquentORM, Blade template engine, etc. to improve development efficiency. 3) Its MVC architecture and dependency injection mechanism make the code more modular and testable. 4) Provides powerful debugging tools and performance optimization methods such as caching systems and best practices.

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.

PHP and Laravel are not directly comparable, because Laravel is a PHP-based framework. 1.PHP is suitable for small projects or rapid prototyping because it is simple and direct. 2. Laravel is suitable for large projects or efficient development because it provides rich functions and tools, but has a steep learning curve and may not be as good as pure PHP.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
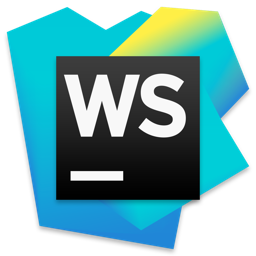
WebStorm Mac version
Useful JavaScript development tools
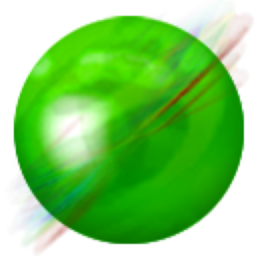
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment