Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1. Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2. Laravel is based on PHP and emphasizes the developer experience, suitable for small to medium-sized projects.
introduction
In modern web development, choosing the right framework is crucial. It not only affects development efficiency, but also determines the maintainability and scalability of the project. Today we will dive into two popular web frameworks, Django and Laravel, to help you make informed choices. Through this article, you will learn about the core features of Django and Laravel, their respective strengths and weaknesses, and how to choose in different scenarios.
Review of basic knowledge
Both Django and Laravel are full-stack frameworks designed to simplify the development of web applications. Django is based on Python, follows the philosophy of "full battery" and has built-in many functions, such as ORM, management background, certification system, etc. Laravel is based on PHP, emphasizing elegant syntax and developer experience, providing powerful ORM Eloquent, art command line tool Artisan, etc.
Core concept or function analysis
The definition and function of Django
Django is known as the "complete" web framework because it provides a complete suite of solutions from databases to user interfaces. Its design philosophy is "DRY" (Don't Repeat Yourself), which means developers can build powerful web applications in a short time.
from django.http import HttpResponse def hello_world(request): return HttpResponse("Hello, world!")
This simple view function demonstrates the simplicity and ease of use of Django.
The definition and function of Laravel
Laravel is known for its elegant syntax and rich feature library, aiming to make PHP development more enjoyable and efficient. Its Blade template engine and Eloquent ORM make data processing and view rendering extremely simple.
Route::get('/', function () { return 'Hello, world!'; });
Here is a simple Laravel routing example that demonstrates its concise syntax.
How it works
Django works based on MVC (Model-View-Controller) mode, but it calls it MTV (Model-Template-View). Django's ORM allows developers to manipulate databases through Python code without writing SQL queries. Its request processing process starts with URL parsing, is processed by view functions, and finally returns a response.
Laravel's working principle is also based on MVC mode. Its request processing process starts from the routing, is processed by the controller, and finally returns the response through the view. Laravel's Eloquent ORM provides powerful data manipulation capabilities, supporting relationship mapping and query construction.
Example of usage
Basic usage of Django
The basic usage of Django includes defining models, creating views, and writing templates. Here is a simple example of model definition:
from django.db import models class Book(models.Model): title = models.CharField(max_length=200) author = models.CharField(max_length=100)
This model defines the title and author of the book, and Django will automatically generate the corresponding database table.
Basic usage of Laravel
The basic usage of Laravel includes defining models, creating controllers, and writing views. Here is a simple example of model definition:
namespace App\Models; use Illuminate\Database\Eloquent\Model; class Book extends Model { protected $fillable = ['title', 'author']; }
This model defines the title and author of the book, and Laravel will automatically generate the corresponding database table.
Advanced Usage
Advanced usage of Django includes using signals, middleware, and custom management commands. Here is an example of using signals:
from django.db.models.signals import post_save from django.dispatch import receiver from .models import Book @receiver(post_save, sender=Book) def book_saved(sender, instance, created, **kwargs): If created: print(f"New book created: {instance.title}")
This signal will be triggered when the book is saved and performs the corresponding operation.
Advanced usage of Laravel includes using events, middleware, and custom Artisan commands. Here is an example of using events:
namespace App\Events; use App\Models\Book; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class BookCreated { use Dispatchable, SerializesModels; public $book; public function __construct(Book $book) { $this->book = $book; } }
This event will be triggered when the book is created and performs the corresponding operation.
Common Errors and Debugging Tips
Common errors in Django include model field definition errors, URL configuration errors, etc. Debugging skills include using Django's debug toolbar, viewing log files, etc.
Common errors in Laravel include model field definition errors, routing configuration errors, etc. Debugging skills include using Laravel's debugging tools, viewing log files, etc.
Performance optimization and best practices
In Django, performance optimization can start from database query optimization, cache usage, asynchronous task processing, etc. Here is an example of using cache:
from django.core.cache import cache def get_book(title): book = cache.get(title) If book is None: book = Book.objects.get(title=title) cache.set(title, book) return book
This function shows how to use Django's cache system to improve performance.
In Laravel, performance optimization can start from database query optimization, cache usage, queue processing, etc. Here is an example of using cache:
use Illuminate\Support\Facades\Cache; function getBook($title) { $book = Cache::get($title); if (is_null($book)) { $book = Book::where('title', $title)->first(); Cache::put($title, $book); } return $book; }
This function shows how to use Laravel's cache system to improve performance.
In-depth insights and suggestions
Django and Laravel each have their own advantages, and which one is chosen depends on your project requirements and the team's technology stack. Django is suitable for fast development and complex business logic, suitable for Python developers; while Laravel attracts PHP developers with its elegant syntax and rich ecosystem.
When choosing, the following points need to be considered:
- Team Skills : Django may be more suitable if your team is familiar with Python; Laravel may be more suitable if your team is familiar with PHP.
- Project requirements : Django is suitable for projects that require rapid development and complex business logic, while Laravel is suitable for projects that require elegant syntax and rich ecosystems.
- Performance requirements : Django performs well when dealing with high concurrency and large data volumes, while Laravel performs well in small to medium-sized projects.
Tap points and suggestions
- Django's Learning Curve : Django's "Battery Full" philosophy provides rich features, but also increases the difficulty of learning. It is recommended that novices start with Django's official tutorial and gradually master its core concepts.
- Laravel's performance issues : Laravel may encounter performance bottlenecks when processing large-scale data. It is recommended to consider using cache and queues to optimize performance early in the project.
- Version Compatibility : Whether it is Django or Laravel, you may encounter compatibility issues when upgrading the version. It is recommended to read the official documents carefully before upgrading and conduct sufficient testing.
Through the in-depth discussion of this article, I hope you can better understand the pros and cons of Django and Laravel, and make the best choice for your project.
The above is the detailed content of Which is better, Django or Laravel?. For more information, please follow other related articles on the PHP Chinese website!

The Laravel development project was chosen because of its flexibility and power to suit the needs of different sizes and complexities. Laravel provides routing system, EloquentORM, Artisan command line and other functions, supporting the development of from simple blogs to complex enterprise-level systems.

The comparison between Laravel and Python in the development environment and ecosystem is as follows: 1. The development environment of Laravel is simple, only PHP and Composer are required. It provides a rich range of extension packages such as LaravelForge, but the extension package maintenance may not be timely. 2. The development environment of Python is also simple, only Python and pip are required. The ecosystem is huge and covers multiple fields, but version and dependency management may be complex.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.

Laravel's popularity includes its simplified development process, providing a pleasant development environment, and rich features. 1) It absorbs the design philosophy of RubyonRails, combining the flexibility of PHP. 2) Provide tools such as EloquentORM, Blade template engine, etc. to improve development efficiency. 3) Its MVC architecture and dependency injection mechanism make the code more modular and testable. 4) Provides powerful debugging tools and performance optimization methods such as caching systems and best practices.

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.

PHP and Laravel are not directly comparable, because Laravel is a PHP-based framework. 1.PHP is suitable for small projects or rapid prototyping because it is simple and direct. 2. Laravel is suitable for large projects or efficient development because it provides rich functions and tools, but has a steep learning curve and may not be as good as pure PHP.

LaravelisabackendframeworkbuiltonPHP,designedforwebapplicationdevelopment.Itfocusesonserver-sidelogic,databasemanagement,andapplicationstructure,andcanbeintegratedwithfrontendtechnologieslikeVue.jsorReactforfull-stackdevelopment.
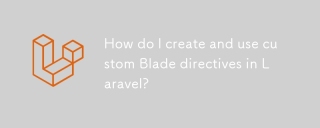
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
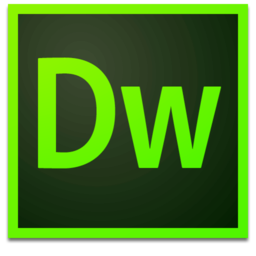
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.