Recommended visual test reporting tools for Golang
Golang’s visual test reporting tool recommendation
Introduction:
In the software development process, testing is an indispensable link. A good test report can help developers better analyze and understand test results, thereby optimizing software quality. This article will introduce several visual test reporting tools in Golang and demonstrate their usage through sample code.
- GoConvey
GoConvey is a powerful Golang testing framework that provides an easy-to-use and beautiful visual test reporting interface. Using GoConvey can greatly simplify the test code writing and result analysis process.
Sample code:
package main import ( "testing" . "github.com/smartystreets/goconvey/convey" ) func TestAddition(t *testing.T) { Convey("Given two numbers", t, func() { a := 5 b := 3 Convey("When adding them together", func() { result := a + b Convey("The result should be correct", func() { So(result, ShouldEqual, 8) }) }) }) }
In the sample code, we introduce GoConvey’s testing framework and assertion library. Using the Convey function to organize the test case, given two numbers, we add them and assert whether the result is correct or not. If everything is OK, the test results will appear in green in GoConvey's visual test reporting interface.
- Ginkgo & Gomega
Ginkgo is another popular Golang testing framework that is used in conjunction with the Gomega assertion library to provide BDD-like test writing and visual reporting capabilities.
Sample code:
package main import ( "testing" . "github.com/onsi/ginkgo" . "github.com/onsi/gomega" ) func TestAddition(t *testing.T) { RegisterFailHandler(Fail) RunSpecs(t, "Addition Suite") } var _ = Describe("Addition", func() { Context("Given two numbers", func() { a := 5 b := 3 It("should add them together correctly", func() { result := a + b Expect(result).To(Equal(8)) }) }) })
In the sample code, we use the Ginkgo testing framework and Gomega assertion library to write test cases. Use the Describe function to describe the test scenario, and then perform specific test operations in the It function. Test results will be displayed in an easy-to-understand manner on Ginkgo's visual test reporting interface.
- Testify
Testify is another popular testing tool library in Golang. It provides a rich set of assertion functions and utility functions, which can help developers write clear and readable tests. Test code and generate beautiful test reports.
Sample code:
package main import ( "testing" "github.com/stretchr/testify/assert" ) func TestAddition(t *testing.T) { a := 5 b := 3 result := a + b assert.Equal(t, 8, result) }
In the sample code, we use the assertion function assert.Equal of the Testify library to determine whether two values are equal. If the assertion fails, Testify will display the failure message in the test report, otherwise, it will display the test passing message.
Conclusion:
In Golang, we can use visual test reporting tools such as GoConvey, Ginkgo & Gomega, and Testify to improve testing efficiency and readability. Through these tools, developers can better organize test cases and analyze test results, thereby improving software quality. I hope the introduction in this article will help you choose the appropriate visual test reporting tool in your Golang project.
The above is the detailed content of Recommended visual test reporting tools for Golang. For more information, please follow other related articles on the PHP Chinese website!
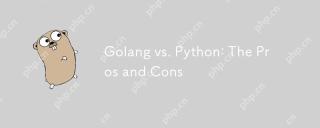
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
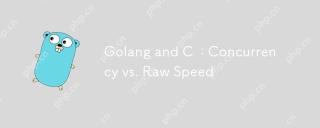
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
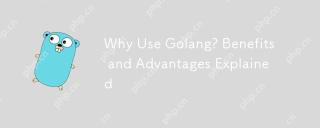
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
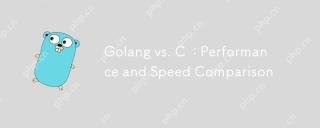
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
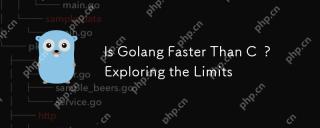
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
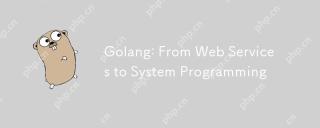
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
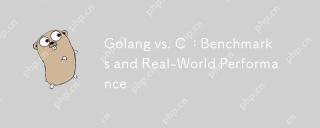
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
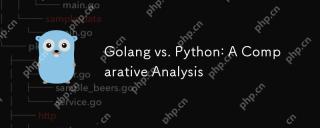
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.