Microservice task scheduler written in Go language
Introduction:
With the popularity of microservice architecture, task scheduler has become an integral part of various systems Essential component. Through the task scheduler, we can implement functions such as scheduled execution of tasks, processing of task dependencies, and monitoring of task execution results. This article will introduce the implementation method of microservice task scheduler written in Go language and illustrate it through code examples.
1. Task scheduling model design
- Task model
The core of the task scheduler is task scheduling, so the task model needs to be defined first. A task can contain the following attributes: - ID: The unique identifier of the task, used to uniquely identify the task.
- Name: The name of the task, used to describe the task.
- Cron: The scheduling period of the task, which can be a fixed time, interval or expression.
- Dependencies: Task dependencies, indicating the task’s predecessor tasks.
- Handler: Task processing function, used to execute specific task logic.
You can define the task model through the following code:
type Task struct {
ID string Name string Cron string Dependencies []string Handler func() error
}
- Task scheduler model
The task scheduler needs to have functions such as task addition, task deletion, task execution, and task dependency processing. The model of the task scheduler can be defined through the following code:
type Scheduler struct {
m sync.Mutex tasks map[string]*Task dependencies map[string][]string
}
- Task scheduler method implementation
Connection Next, we need to implement the task scheduler method. You can add tasks, delete tasks, execute tasks, handle task dependencies and other functions to the task scheduler through the following code:
func (s Scheduler) AddTask(task Task) {
s.m.Lock() defer s.m.Unlock() s.tasks[task.ID] = task // 处理任务依赖关系 for _, dependency := range task.Dependencies { s.dependencies[dependency] = append(s.dependencies[dependency], task.ID) }
}
func (s *Scheduler) RemoveTask(taskID string) {
s.m.Lock() defer s.m.Unlock() task, ok := s.tasks[taskID] if ok { delete(s.tasks, taskID) // 清理任务依赖关系 for _, dependent := range s.dependencies[taskID] { dependentTask, ok := s.tasks[dependent] if ok { dependentTask.Dependencies = remove(dependentTask.Dependencies, taskID) } } delete(s.dependencies, taskID) }
}
func (s *Scheduler) RunTask(taskID string) {
s.m.Lock() defer s.m.Unlock() task, ok := s.tasks[taskID] if ok { err := task.Handler() if err != nil { fmt.Printf("Task %s failed to execute: %s
", taskID, err.Error())
} }
}
func (s *Scheduler) handleDependencies(taskID string) {
dependentTasks, ok := s.dependencies[taskID] if ok { for _, dependent := range dependentTasks { s.RunTask(dependent) } }
}
func (s *Scheduler) RunAllTasks() {
s.m.Lock() defer s.m.Unlock() for _, task := range s.tasks { s.RunTask(task.ID) }
}
Code analysis:
- The AddTask method is used to add tasks to tasks Add tasks to the scheduler and handle task dependencies.
- RemoveTask method is used to remove tasks from the task scheduler and clean up task dependencies.
- RunTask method is used to execute tasks , and print the error message that the task execution failed.
- The handleDependencies method is used to handle the dependencies of the task, that is, execute all tasks that depend on the specified task.
- The RunAllTasks method is used in the execution scheduler All tasks.
2. Usage Example
The following is a simple example to show how to use the microservice task scheduler.
func main() {
scheduler := &Scheduler{ tasks: make(map[string]*Task), dependencies: make(map[string][]string), } // 初始化任务 task1 := &Task{ ID: "1", Name: "Task 1", Cron: "* * * * *", // 每分钟执行一次 Handler: func() error { fmt.Println("Task 1 Executed") return nil }, } task2 := &Task{ ID: "2", Name: "Task 2", Cron: "* * * * *", // 每分钟执行一次 Dependencies: []string{"1"}, Handler: func() error { fmt.Println("Task 2 Executed") return nil }, } // 添加任务到任务调度器中 scheduler.AddTask(task1) scheduler.AddTask(task2) // 执行任务 scheduler.RunAllTasks() // 删除任务 scheduler.RemoveTask("2") // 再次执行任务 scheduler.RunAllTasks()
}
Code analysis:
- First, we create a task scheduler instance and initialize the task scheduler.
- Then, we create Two tasks, and set the scheduling cycle, dependencies and processing functions of the tasks.
- Next, we add the task to the task scheduler.
- Then, we execute the tasks in the task scheduler All tasks.
- Finally, we delete a task and execute all tasks in the task scheduler again.
Summary:
This article introduces the microprocessor written in Go language The implementation method of the service task scheduler is explained through code examples. Through the task scheduler, we can implement functions such as scheduled execution of tasks, processing of task dependencies, and monitoring of task execution results, providing a microservice architecture for the system. Provides powerful task scheduling support.
The above is the detailed content of Microservice task scheduler written in Go language. For more information, please follow other related articles on the PHP Chinese website!
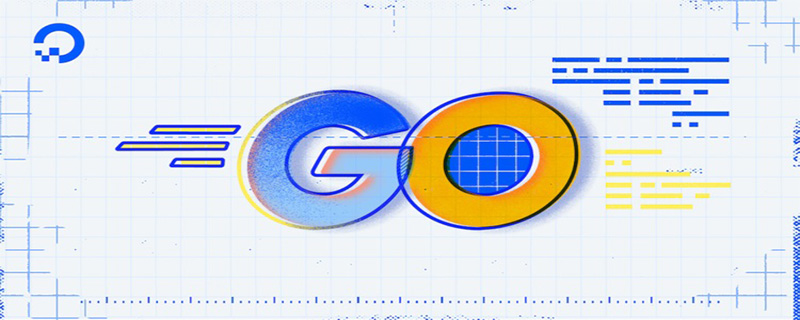
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
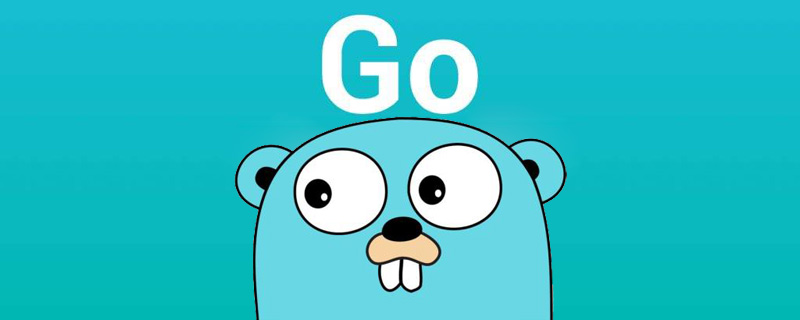
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
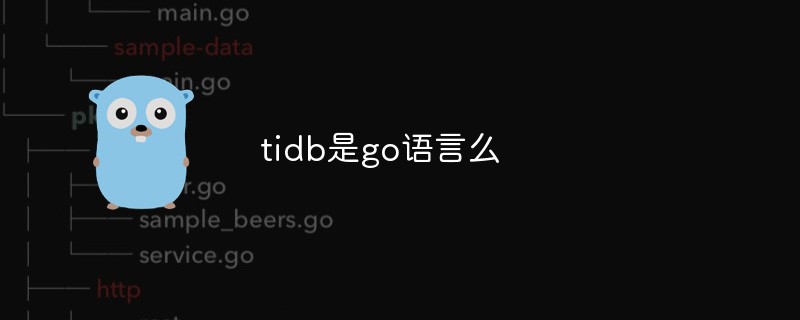
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
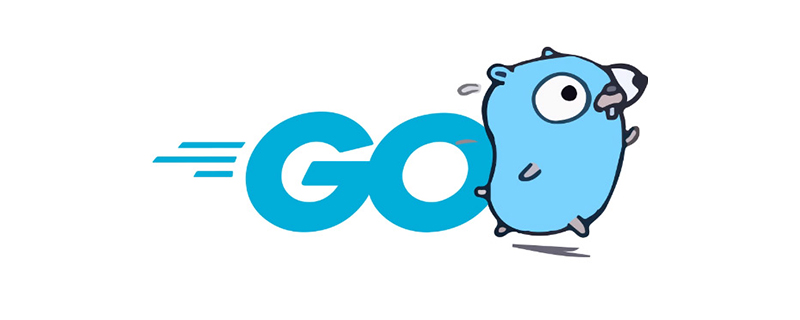
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
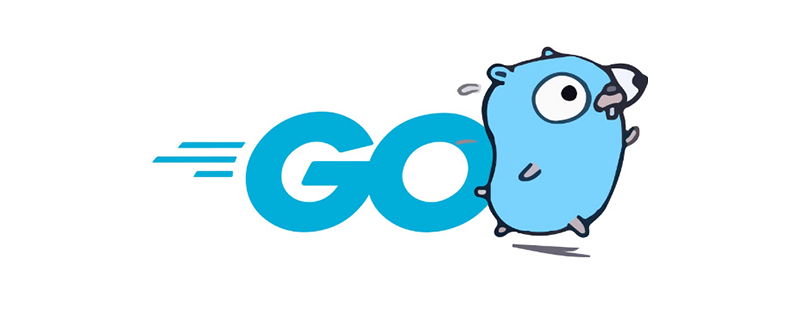
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
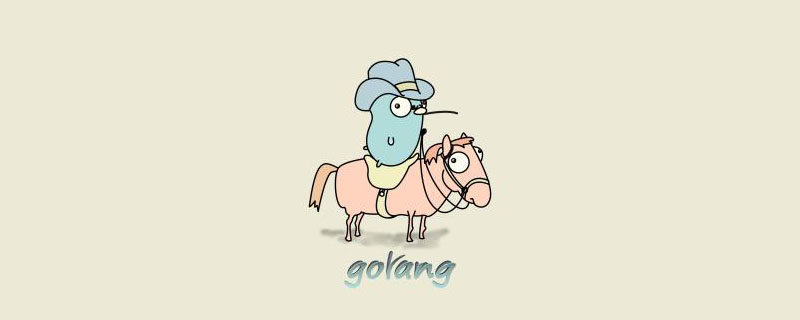
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
