Use Swoole to build a high-performance API server
With the rapid development of Internet technology, more and more applications need to face high concurrency and high performance problems . Traditional PHP applications are often unable to meet these needs due to their single-threaded characteristics. To solve this problem, Swoole came into being. Swoole is a PHP extension that provides the ability to use asynchronous, coroutine and high concurrency in PHP, allowing us to easily build high-performance API servers.
In this article, we will introduce how to use Swoole to build a simple API server and provide code samples for readers' reference.
First, we need to install the Swoole extension. It can be installed in the Linux environment through the following command:
$ pecl install swoole
After the installation is completed, add the following line in the php.ini file:
extension=swoole.so
Then restart PHP to ensure that the Swoole extension has been loaded successfully.
Next, we will write a simple API server code and implement a simple interface for querying the user's basic information. The following is a sample code:
<?php $http = new SwooleHttpServer("0.0.0.0", 8080); $http->on('request', function ($request, $response) { // 解析请求参数 $query = $request->get['query']; // 处理业务逻辑 $result = getUserInfo($query); // 返回结果 $response->header('Content-Type', 'application/json'); $response->end(json_encode($result)); }); $http->start(); // 模拟查询用户信息的方法 function getUserInfo($query) { // 这里可以连接数据库,查询用户信息,这里只是简单返回一个示例结果 return [ 'name' => 'John', 'age' => 25, 'query' => $query ]; }
In the above code, we first create a Swoole HTTP server and specify the running IP address and port number.
Then, we registered a callback function for the request
event. Whenever a request arrives, Swoole will call this callback function. In the callback function, we first parse the parameters passed in the request, then call the getUserInfo
method to process the business logic, and finally return the result.
Finally, we started the Swoole server by calling the start
method. Now, we can test this API interface by visiting http://localhost:8080?query=123
.
By using Swoole, we can easily build a high-performance API server and be able to handle a large number of concurrent requests. In actual projects, this example can be further expanded to add more interfaces and functions to meet specific business needs.
To sum up, Swoole provides powerful asynchronous, coroutine and high-concurrency processing capabilities, which can help us build high-performance API servers. Through the introduction and sample code of this article, I believe readers will have a deeper understanding of how to use Swoole to build an API server. Hope this article helps you!
The above is the detailed content of Use Swoole to build a high-performance API server. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
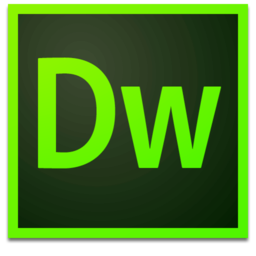
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
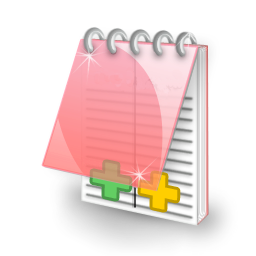
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function