Develop interactive map applications using JavaScript
Develop interactive map applications using JavaScript
Introduction:
Nowadays, map applications have become an important part of our daily lives. Whether it's finding directions, checking out nearby stores, or exploring unknown areas, Maps apps make it easy. In this article, we will learn to use JavaScript to develop an interactive map application, and add code examples to help readers better understand.
- Create the HTML structure:
First, we need to create the basic structure required for the map application in the HTML file. By using HTML5's<div> element, we can create a container to hold the map. The following is a simple HTML structure example: <pre class='brush:php;toolbar:false;'><!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>交互式地图应用</title> <style> #map { width: 100%; height: 600px; } </style> </head> <body> <div id="map"></div> <script src="app.js"></script> </body> </html></pre><p> In this example, we create a <code><div> element with the id <code>map
, Used to display maps. We've also included a JavaScript file calledapp.js
, which will contain our map app code.- Add map API:
Next, we need to load the map by introducing the map API. In this article, we will use Google Maps API as the map provider. In theapp.js
file, we can introduce the Google Maps API as follows:
// app.js function initMap() { // 创建地图实例 var map = new google.maps.Map(document.getElementById('map'), { center: {lat: 37.7749, lng: -122.4194}, // 地图中心点坐标 zoom: 12 // 缩放级别 }); } // 页面加载完成时调用初始化函数 window.onload = function() { initMap(); };
In this example, we first define a file called
initMap( )
function, used to initialize the map when the page is loaded. Inside the function body, we create a newgoogle.maps.Map
instance and set its center point to the latitude and longitude coordinates of San Francisco. The zoom level is set to 12 to show moderate detail.- Add interactive functions:
In order to make the map application more interactive, we can add some functions to meet user needs. Here are some common examples of interactive functionality:
a. Adding markers:
// app.js function initMap() { var map = new google.maps.Map(...); // 创建标记点 var marker = new google.maps.Marker({ position: {lat: 37.7749, lng: -122.4194}, // 标记点坐标 map: map, // 关联到地图实例 title: '旧金山' // 标记点标题(可选) }); }
In this example, we use the
google.maps.Marker
class A marker namedmarker
is created. We set the latitude and longitude coordinates of the marker point through theposition
attribute, and associate the marker point to the map using themap
attribute. Finally, we can also add a title (optional) to the marker using thetitle
attribute.b. Find a location:
// app.js function initMap() { var map = new google.maps.Map(...); // 创建搜索框 var input = document.getElementById('search'); var searchBox = new google.maps.places.SearchBox(input); // 监听搜索框值改变事件 searchBox.addListener('places_changed', function() { var places = searchBox.getPlaces(); if (places.length == 0) { return; } // 标记搜索结果 var bounds = new google.maps.LatLngBounds(); places.forEach(function(place) { if (!place.geometry) { console.log("返回的结果不包含几何信息"); return; } var marker = new google.maps.Marker({ map: map, position: place.geometry.location }); bounds.extend(place.geometry.location); }); map.fitBounds(bounds); }); }
In this example, we first create an input box for the user to enter the location they want to find. Then, we use the
google.maps.places.SearchBox
class to create a search box object namedsearchBox
and associate the input box with the search box. We use theaddListener
method to listen to the search box value change event, and in the event handler function, obtain the search results through thesearchBox.getPlaces()
method, mark these results on the map, and Automatically adapt the map perspective to display search results.- Conclusion:
By using JavaScript to develop interactive map applications, we can provide users with a better map browsing and interactive experience. This article explains how to create a basic HTML structure, load maps using the Google Maps API, and add interactive features. I hope this article can help readers better understand and apply JavaScript to develop map applications.
Reference:
- [Google Maps JavaScript API](https://developers.google.com/maps/documentation/javascript/overview)
- [Google Places API](https://developers.google.com/maps/documentation/places/web-service/overview)
The copyright of the code examples belongs to the original author. Please comply with relevant licenses and legal regulations when using it.
- Add map API:
The above is the detailed content of Develop interactive map applications using JavaScript. For more information, please follow other related articles on the PHP Chinese website!
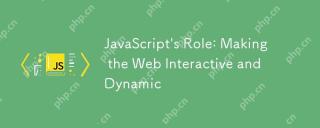
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
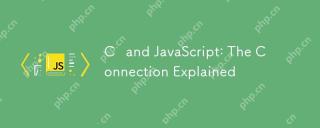
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
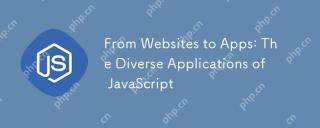
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
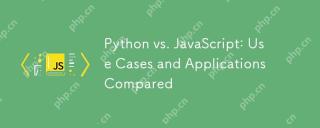
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
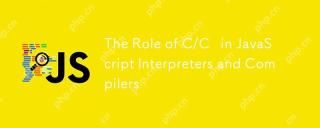
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
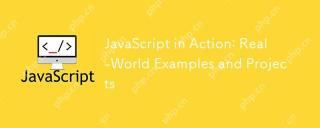
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
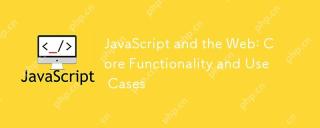
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
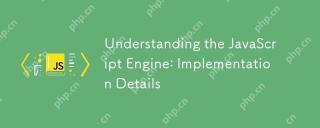
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
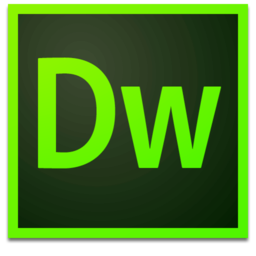
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
